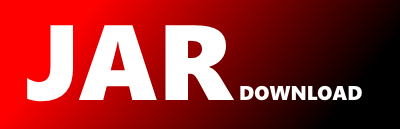
org.abego.guitesting.swing.FocusSupport Maven / Gradle / Ivy
/*
* MIT License
*
* Copyright (c) 2019 Udo Borkowski, ([email protected])
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
package org.abego.guitesting.swing;
import org.abego.commons.timeout.TimeoutSupplier;
import org.abego.commons.timeout.Timeoutable;
import org.eclipse.jdt.annotation.Nullable;
import java.awt.Component;
import java.awt.KeyboardFocusManager;
/**
* (Keyboard) Focus related operations.
*/
public interface FocusSupport extends TimeoutSupplier {
/**
* Returns the focus owner, i.e. the {@link Component} that will receive all
* KeyEvents generated by the user; returns {@code null} if no focus owner is
* defined.
*
* For details see {@link KeyboardFocusManager#getFocusOwner()}.
*
* @return the focus owner or {@code null} if no focus owner is defined.
*/
@Nullable
Component focusOwner();
/**
* Waits until any component has the focus.
*/
@Timeoutable
void waitUntilAnyFocus();
/**
* Waits until {@code component} has the focus.
*
*
* Other than {@link #setFocusOwner(Component)} this method does NOT
* actively change the focus but just waits until this happens.
*
* @param component the {@link Component} expected to receive the focus.
*/
@Timeoutable
void waitUntilInFocus(Component component);
/**
* Makes the {@code component} have the focus.
*
*
* The method returns when the {@code component} received the focus.
*
* @param component the {@link Component} to receive the focus.
*/
@Timeoutable
void setFocusOwner(Component component);
/**
* Moves the focus to the next focusable component and waits until the focus
* switched to a different component.
*
* Important: as the function only returns when the focus has changed make
* sure there is a "next" component to switch to (that is different from the
* current focus owner).
*/
@Timeoutable
void focusNext();
/**
* Moves the focus to the previous focusable component and returns when the
* focus switched to a different component.
*
* Important: as the function only returns when the focus has changed make
* sure there is a "previous" component to switch to, that is different from
* the current focus owner.
*/
@Timeoutable
void focusPrevious();
/**
* Sets the focus to the {@code component} and runs the {@code runnable}.
*
* @param component the {@link Component} to receive the focus.
* @param runnable the {@link Runnable} to run.
*/
@Timeoutable
default void runWithFocusIn(Component component, Runnable runnable) {
setFocusOwner(component);
runnable.run();
}
}