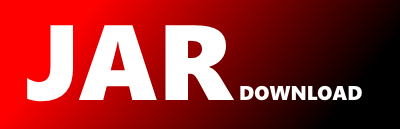
org.accidia.dbz.modules.MySQLStorageModule Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dbz Show documentation
Show all versions of dbz Show documentation
DBZ provides a simple interface to store/retrive key/value pairs on BerkeleyDB JE or MySQL
The newest version!
package org.accidia.dbz.modules;
import com.google.common.base.Strings;
import com.google.common.net.HostAndPort;
import com.google.inject.AbstractModule;
import com.google.inject.Singleton;
import com.mchange.v2.c3p0.ComboPooledDataSource;
import org.accidia.dbz.Constants;
import org.accidia.dbz.IDbz;
import org.accidia.dbz.impl.DbzOnMysqlImpl;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import javax.sql.DataSource;
import java.beans.PropertyVetoException;
import static com.google.common.base.Preconditions.checkArgument;
public class MySQLStorageModule extends AbstractModule {
private static final Logger logger = LoggerFactory.getLogger(MySQLStorageModule.class);
private final HostAndPort hostAndPort;
private final String database;
private final String username;
private final String password;
private MySQLStorageModule() {
logger.debug("MySQLStorageModule()");
throw new AssertionError();
}
public MySQLStorageModule(final String database) {
logger.debug("MySQLStorageModule(database)");
checkArgument(!Strings.isNullOrEmpty(database), "null/empty database");
this.hostAndPort = Constants.MySQLStorageModule.DEFAULT_HOST_AND_PORT;
this.database = database;
this.username = Constants.MySQLStorageModule.DEFAULT_USERNAME;
this.password = Constants.MySQLStorageModule.DEFAULT_PASSWORD;
}
public MySQLStorageModule(final HostAndPort hostAndPort,
final String database,
final String username,
final String password) {
logger.debug("MySQLStorageModule(hostAndPort,database,username,password)");
checkArgument(!Strings.isNullOrEmpty(database), "null/empty database");
this.hostAndPort = hostAndPort;
this.database = database;
this.username = username;
this.password = password;
}
@Override
protected void configure() {
logger.debug("configure()");
try {
final String url = String.format(
"jdbc:mysql://%s:%s/%s?useUnicode=true&characterEncoding=UTF-8",
this.hostAndPort.getHostText(), this.hostAndPort.getPort(),
this.database
);
final ComboPooledDataSource dataSource = new ComboPooledDataSource();
dataSource.setDriverClass("com.mysql.jdbc.Driver");
dataSource.setJdbcUrl(url);
dataSource.setUser(this.username);
dataSource.setPassword(this.password);
dataSource.setTestConnectionOnCheckout(true);
bind(DataSource.class).toInstance(dataSource);
bind(IDbz.class).to(DbzOnMysqlImpl.class).in(Singleton.class);
} catch (PropertyVetoException e) {
logger.error("veto exception: ", e);
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy