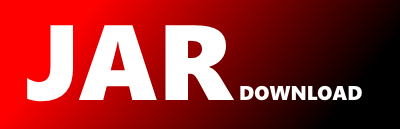
org.acegisecurity.domain.dao.EvictionUtils Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.domain.dao;
import org.acegisecurity.domain.PersistableEntity;
import org.springframework.util.Assert;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.Iterator;
/**
* Convenience methods that support eviction of PersistableEntity
s from those objects that implement
* {@link EvictionCapable}.
*
* @author Ben Alex
* @version $Id: EvictionUtils.java 1496 2006-05-23 13:38:33Z benalex $
*/
public class EvictionUtils {
//~ Methods ========================================================================================================
/**
* Evicts the PersistableEntity
using the passed Object
(provided that the passed
* Object
implements EvictionCapable
).
*
* @param daoOrServices the potential source for EvictionCapable
services (never null
)
* @param entity to evict (can be null
)
*/
public static void evictIfRequired(Object daoOrServices, PersistableEntity entity) {
EvictionCapable evictor = getEvictionCapable(daoOrServices);
if ((evictor != null) && (entity != null)) {
evictor.evict(entity);
}
}
/**
* Evicts each PersistableEntity
element of the passed Collection
using the
* passed Object
(provided that the passed Object
implements
* EvictionCapable
).
*
* @param daoOrServices the potential source for EvictionCapable
services (never null
)
* @param collection whose members to evict (never null
)
*/
public static void evictIfRequired(Object daoOrServices, Collection collection) {
Assert.notNull(collection, "Cannot evict a null Collection");
if (getEvictionCapable(daoOrServices) == null) {
// save expense of iterating collection
return;
}
Iterator iter = collection.iterator();
while (iter.hasNext()) {
Object obj = iter.next();
if (obj instanceof PersistableEntity) {
evictIfRequired(daoOrServices, (PersistableEntity) obj);
}
}
}
/**
* Evicts the PersistableEntity
using the passed Object
(provided that the passed
* Object
implements EvictionCapable
), along with expressly evicting every
* PersistableEntity
returned by the PersistableEntity
's getters.
*
* @param daoOrServices the potential source for EvictionCapable
services (never null
)
* @param entity to evict includnig its getter results (can be null
)
*/
public static void evictPopulatedIfRequired(Object daoOrServices, PersistableEntity entity) {
EvictionCapable evictor = getEvictionCapable(daoOrServices);
if ((evictor != null) && (entity != null)) {
evictor.evict(entity);
Method[] methods = entity.getClass().getMethods();
for (int i = 0; i < methods.length; i++) {
if (methods[i].getName().startsWith("get") && (methods[i].getParameterTypes().length == 0)) {
try {
Object result = methods[i].invoke(entity, new Object[] {});
if (result instanceof PersistableEntity) {
evictor.evict((PersistableEntity) result);
}
} catch (Exception ignored) {}
}
}
}
}
/**
* Obtain the EvictionCapable
from the passed argument, or null
.
*
* @param daoOrServices to check if provides eviction services
*
* @return the EvictionCapable
object or null
if the object does not provide eviction
* services
*/
private static EvictionCapable getEvictionCapable(Object daoOrServices) {
Assert.notNull(daoOrServices, "Cannot evict if the object that may provide EvictionCapable is null");
if (daoOrServices instanceof EvictionCapable) {
return (EvictionCapable) daoOrServices;
} else {
return null;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy