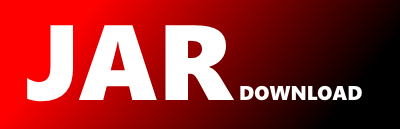
org.acegisecurity.domain.validation.ValidationManagerImpl Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.domain.validation;
import org.acegisecurity.domain.dao.DetachmentContextHolder;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.util.Assert;
import org.springframework.validation.BindException;
import org.springframework.validation.Errors;
import org.springframework.validation.Validator;
import java.util.Iterator;
import java.util.List;
import java.util.Vector;
/**
* Default implementation of {@link ValidationManager}.
*
* @author Ben Alex
* @author Matthew E. Porter
* @version $Id: ValidationManagerImpl.java 1496 2006-05-23 13:38:33Z benalex $
*/
public class ValidationManagerImpl implements InitializingBean, ValidationManager {
//~ Instance fields ================================================================================================
private IntrospectionManager introspectionManager;
protected final Log logger = LogFactory.getLog(getClass());
private ValidationRegistryManager validationRegistryManager = new ValidationRegistryManagerImpl();
private boolean strictValidation = true;
//~ Methods ========================================================================================================
public void afterPropertiesSet() throws Exception {
Assert.notNull(validationRegistryManager, "A ValidationRegistryManager is required");
Assert.notNull(introspectionManager, "An IntrospectionManager is required");
}
private Validator findValidator(Class clazz) throws ValidatorNotFoundException {
Assert.notNull(clazz, "Class cannot be null");
Validator validator = this.validationRegistryManager.findValidator(clazz);
if (validator == null) {
throw new ValidatorNotFoundException("No Validator found for class '" + clazz + "'");
}
return validator;
}
public IntrospectionManager getIntrospectionManager() {
return introspectionManager;
}
public ValidationRegistryManager getValidationRegistryManager() {
return validationRegistryManager;
}
public boolean isStrictValidation() {
return strictValidation;
}
/**
* Locates all immediate children of the passed parentObject
, adding each of those immediate
* children to the allObjects
list and then calling this same method for each of those immediate
* children.Does not add the passed parentObject
to the allObjects
* list. The caller of this method should ensure the parentObject
is added to the list instead.
*
* @param parentObject the object we wish to locate all children for
* @param allObjects the list to add the located children to
*/
private void obtainAllChildren(Object parentObject, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy