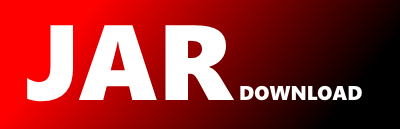
org.acegisecurity.annotation.SecurityAnnotationAttributes Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.annotation;
import org.acegisecurity.SecurityConfig;
import org.springframework.metadata.Attributes;
import org.springframework.util.ClassUtils;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
/**
* Java 5 Annotation Attributes
metadata implementation used for secure method interception.This
* Attributes
implementation will return security configuration for classes described using the
* Secured
Java 5 annotation.
* The SecurityAnnotationAttributes
implementation can be used to configure a
* MethodDefinitionAttributes
and MethodSecurityInterceptor
bean definition (see below).
* For example:
<bean id="attributes"
* class="org.acegisecurity.annotation.SecurityAnnotationAttributes"/><bean id="objectDefinitionSource"
* class="org.acegisecurity.intercept.method.MethodDefinitionAttributes"> <property name="attributes">
* <ref local="attributes"/> </property></bean><bean id="securityInterceptor"
* class="org.acegisecurity.intercept.method.aopalliance.MethodSecurityInterceptor"> . . .
* <property name="objectDefinitionSource"> <ref local="objectDefinitionSource"/> </property>
* </bean>
* These security annotations are similiar to the Commons Attributes approach, however they are using Java 5
* language-level metadata support.
* This class should be used with Spring 2.0 or above, as it relies upon utility classes in Spring 2.0 for
* correct introspection of annotations on bridge methods.
*
* @author Mark St.Godard
* @version $Id: SecurityAnnotationAttributes.java 1756 2006-11-17 02:17:45Z benalex $
*
* @see org.acegisecurity.annotation.Secured
*/
public class SecurityAnnotationAttributes implements Attributes {
//~ Methods ========================================================================================================
/**
* Get the Secured
attributes for a given target class.
*
* @param target The target method
*
* @return Collection of SecurityConfig
*
* @see Attributes#getAttributes
*/
public Collection getAttributes(Class target) {
Set attributes = new HashSet();
for (Annotation annotation : target.getAnnotations()) {
// check for Secured annotations
if (annotation instanceof Secured) {
Secured attr = (Secured) annotation;
for (String auth : attr.value()) {
attributes.add(new SecurityConfig(auth));
}
break;
}
}
return attributes;
}
public Collection getAttributes(Class clazz, Class filter) {
throw new UnsupportedOperationException("Unsupported operation");
}
/**
* Get the Secured
attributes for a given target method.
*
* @param method The target method
*
* @return Collection of SecurityConfig
*
* @see Attributes#getAttributes
*/
public Collection getAttributes(Method method) {
Set attributes = new HashSet();
Annotation[] annotations = null;
// Use AnnotationUtils if in classpath (ie Spring 1.2.9+ deployment)
try {
Class clazz = ClassUtils.forName("org.springframework.core.annotation.AnnotationUtils");
Method m = clazz.getMethod("getAnnotations", new Class[] {Method.class});
annotations = (Annotation[]) m.invoke(null, new Object[] {method});
} catch (Exception ex) {
// Fallback to manual retrieval if no AnnotationUtils available
annotations = method.getAnnotations();
}
for (Annotation annotation : annotations) {
// check for Secured annotations
if (annotation instanceof Secured) {
Secured attr = (Secured) annotation;
for (String auth : attr.value()) {
attributes.add(new SecurityConfig(auth));
}
break;
}
}
return attributes;
}
public Collection getAttributes(Method method, Class clazz) {
throw new UnsupportedOperationException("Unsupported operation");
}
public Collection getAttributes(Field field) {
throw new UnsupportedOperationException("Unsupported operation");
}
public Collection getAttributes(Field field, Class clazz) {
throw new UnsupportedOperationException("Unsupported operation");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy