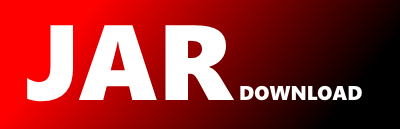
org.acegisecurity.Authentication Maven / Gradle / Ivy
/* Copyright 2004, 2005 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity;
import java.io.Serializable;
import java.security.Principal;
/**
* Represents an authentication request.
*
*
* An Authentication
object is not considered authenticated until
* it is processed by an {@link AuthenticationManager}.
*
*
*
* Stored in a request {@link
* org.acegisecurity.context.security.SecurityContext}.
*
*
* @author Ben Alex
* @version $Id: Authentication.java,v 1.11 2006/02/08 01:24:37 luke_t Exp $
*/
public interface Authentication extends Principal, Serializable {
//~ Methods ================================================================
/**
* See {@link #isAuthenticated()} for a full description.
*
*
* Implementations should always allow this method to be called with
* a false
parameter, as this is used by various classes to
* specify the authentication token should not be trusted. If an
* implementation wishes to reject an invocation with a true
* parameter (which would indicate the authentication token is trusted - a
* potential security risk) the implementation should throw an {@link
* IllegalArgumentException}.
*
*
* @param isAuthenticated true
if the token should be trusted
* (which may result in an exception) or false
if the
* token should not be trusted
*
* @throws IllegalArgumentException if an attempt to make the
* authentication token trusted (by passing true
as
* the argument) is rejected due to the implementation being
* immutable or implementing its own alternative approach to
* {@link #isAuthenticated()}
*/
public void setAuthenticated(boolean isAuthenticated)
throws IllegalArgumentException;
/**
* Used to indicate to AbstractSecurityInterceptor
whether it
* should present the authentication token to the
* AuthenticationManager
. Typically an
* AuthenticationManager
(or, more often, one of its
* AuthenticationProvider
s) will return an immutable
* authentication token after successful authentication, in which case
* that token can safely return true
to this method.
* Returning true
will improve performance, as calling the
* AuthenticationManager
for every request will no longer be
* necessary.
*
*
* For security reasons, implementations of this interface should be very
* careful about returning true
to this method unless they
* are either immutable, or have some way of ensuring the properties have
* not been changed since original creation.
*
*
* @return true if the token has been authenticated and the
* AbstractSecurityInterceptor
does not need to
* represent the token for re-authentication to the
* AuthenticationManager
*/
public boolean isAuthenticated();
/**
* Set by an AuthenticationManager
to indicate the authorities
* that the principal has been granted. Note that classes should not rely
* on this value as being valid unless it has been set by a trusted
* AuthenticationManager
.
*
* Implementations should ensure that modifications to the returned array
* do not affect the state of the Authentication object (e.g. by returning an
* array copy).
*
*
* @return the authorities granted to the principal, or null
* if authentication has not been completed
*/
public GrantedAuthority[] getAuthorities();
/**
* The credentials that prove the principal is correct. This is usually a
* password, but could be anything relevant to the
* AuthenticationManager
. Callers are expected to populate
* the credentials.
*
* @return the credentials that prove the identity of the
* Principal
*/
public Object getCredentials();
/**
* Stores additional details about the authentication request. These might
* be an IP address, certificate serial number etc.
*
* @return additional details about the authentication request, or
* null
if not used
*/
public Object getDetails();
/**
* The identity of the principal being authenticated. This is usually a
* username. Callers are expected to populate the principal.
*
* @return the Principal
being authenticated
*/
public Object getPrincipal();
}