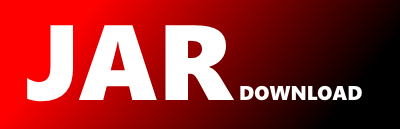
org.acegisecurity.providers.cas.CasAuthenticationToken Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.providers.cas;
import org.acegisecurity.GrantedAuthority;
import org.acegisecurity.providers.AbstractAuthenticationToken;
import org.acegisecurity.userdetails.UserDetails;
import java.io.Serializable;
import java.util.List;
/**
* Represents a successful CAS Authentication
.
*
* @author Ben Alex
* @version $Id: CasAuthenticationToken.java,v 1.13 2006/02/09 04:16:50 benalex Exp $
*/
public class CasAuthenticationToken extends AbstractAuthenticationToken
implements Serializable {
//~ Instance fields ========================================================
private List proxyList;
private Object credentials;
private Object principal;
private String proxyGrantingTicketIou;
private UserDetails userDetails;
private int keyHash;
//~ Constructors ===========================================================
/**
* Constructor.
*
* @param key to identify if this object made by a given {@link
* CasAuthenticationProvider}
* @param principal the username from CAS (cannot be null
)
* @param credentials the service/proxy ticket ID from CAS (cannot be
* null
)
* @param authorities the authorities granted to the user (from {@link
* CasAuthoritiesPopulator}) (cannot be null
)
* @param userDetails the user details (from {@link
* CasAuthoritiesPopulator}) (cannot be null
)
* @param proxyList the list of proxies from CAS (cannot be
* null
)
* @param proxyGrantingTicketIou the PGT-IOU ID from CAS (cannot be
* null
, but may be an empty String
if no
* PGT-IOU ID was provided)
*
* @throws IllegalArgumentException if a null
was passed
*/
public CasAuthenticationToken(String key, Object principal,
Object credentials, GrantedAuthority[] authorities,
UserDetails userDetails, List proxyList, String proxyGrantingTicketIou) {
super(authorities);
if ((key == null) || ("".equals(key)) || (principal == null)
|| "".equals(principal) || (credentials == null)
|| "".equals(credentials) || (authorities == null)
|| (userDetails == null) || (proxyList == null)
|| (proxyGrantingTicketIou == null)) {
throw new IllegalArgumentException(
"Cannot pass null or empty values to constructor");
}
this.keyHash = key.hashCode();
this.principal = principal;
this.credentials = credentials;
this.userDetails = userDetails;
this.proxyList = proxyList;
this.proxyGrantingTicketIou = proxyGrantingTicketIou;
setAuthenticated(true);
}
//~ Methods ================================================================
public boolean equals(Object obj) {
if (!super.equals(obj)) {
return false;
}
if (obj instanceof CasAuthenticationToken) {
CasAuthenticationToken test = (CasAuthenticationToken) obj;
// proxyGrantingTicketIou is never null due to constructor
if (!this.getProxyGrantingTicketIou()
.equals(test.getProxyGrantingTicketIou())) {
return false;
}
// proxyList is never null due to constructor
if (!this.getProxyList().equals(test.getProxyList())) {
return false;
}
if (this.getKeyHash() != test.getKeyHash()) {
return false;
}
return true;
}
return false;
}
public Object getCredentials() {
return this.credentials;
}
public int getKeyHash() {
return this.keyHash;
}
public Object getPrincipal() {
return this.principal;
}
/**
* Obtains the proxy granting ticket IOU.
*
* @return the PGT IOU-ID or an empty String
if no proxy
* callback was requested when validating the service ticket
*/
public String getProxyGrantingTicketIou() {
return proxyGrantingTicketIou;
}
public List getProxyList() {
return proxyList;
}
public UserDetails getUserDetails() {
return userDetails;
}
public String toString() {
StringBuffer sb = new StringBuffer();
sb.append(super.toString());
sb.append("; Credentials (Service/Proxy Ticket): ")
.append(this.credentials);
sb.append("; Proxy-Granting Ticket IOU: ")
.append(this.proxyGrantingTicketIou);
sb.append("; Proxy List: ").append(this.proxyList);
return (sb.toString());
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy