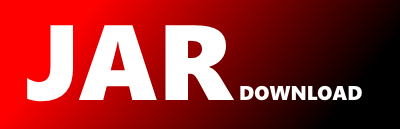
org.acegisecurity.providers.dao.DaoAuthenticationProvider Maven / Gradle / Ivy
/* Copyright 2004, 2005 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.providers.dao;
import org.acegisecurity.AuthenticationException;
import org.acegisecurity.AuthenticationServiceException;
import org.acegisecurity.BadCredentialsException;
import org.acegisecurity.providers.AuthenticationProvider;
import org.acegisecurity.providers.UsernamePasswordAuthenticationToken;
import org.acegisecurity.providers.encoding.PasswordEncoder;
import org.acegisecurity.providers.encoding.PlaintextPasswordEncoder;
import org.acegisecurity.userdetails.UserDetails;
import org.acegisecurity.userdetails.UserDetailsService;
import org.springframework.dao.DataAccessException;
import org.springframework.util.Assert;
/**
* An {@link AuthenticationProvider} implementation that retrieves user details
* from an {@link UserDetailsService}.
*
* @author Ben Alex
* @version $Id: DaoAuthenticationProvider.java,v 1.42 2005/12/24 10:03:18 benalex Exp $
*/
public class DaoAuthenticationProvider
extends AbstractUserDetailsAuthenticationProvider {
//~ Instance fields ========================================================
private UserDetailsService userDetailsService;
private PasswordEncoder passwordEncoder = new PlaintextPasswordEncoder();
private SaltSource saltSource;
//~ Methods ================================================================
protected void additionalAuthenticationChecks(UserDetails userDetails,
UsernamePasswordAuthenticationToken authentication)
throws AuthenticationException {
Object salt = null;
if (this.saltSource != null) {
salt = this.saltSource.getSalt(userDetails);
}
if (!passwordEncoder.isPasswordValid(userDetails.getPassword(),
authentication.getCredentials().toString(), salt)) {
throw new BadCredentialsException(messages.getMessage(
"AbstractUserDetailsAuthenticationProvider.badCredentials",
"Bad credentials"), userDetails);
}
}
protected void doAfterPropertiesSet() throws Exception {
Assert.notNull(this.userDetailsService,
"An Authentication DAO must be set");
}
public UserDetailsService getUserDetailsService() {
return userDetailsService;
}
public PasswordEncoder getPasswordEncoder() {
return passwordEncoder;
}
public SaltSource getSaltSource() {
return saltSource;
}
protected final UserDetails retrieveUser(String username,
UsernamePasswordAuthenticationToken authentication)
throws AuthenticationException {
UserDetails loadedUser;
try {
loadedUser = this.userDetailsService.loadUserByUsername(username);
} catch (DataAccessException repositoryProblem) {
throw new AuthenticationServiceException(
repositoryProblem.getMessage(), repositoryProblem );
}
if (loadedUser == null) {
throw new AuthenticationServiceException(
"AuthenticationDao returned null, which is an interface contract violation");
}
return loadedUser;
}
public void setUserDetailsService(UserDetailsService authenticationDao) {
this.userDetailsService = authenticationDao;
}
/**
* Sets the PasswordEncoder instance to be used to encode and validate
* passwords. If not set, {@link PlaintextPasswordEncoder} will be
* used by default.
*
* @param passwordEncoder The passwordEncoder to use
*/
public void setPasswordEncoder(PasswordEncoder passwordEncoder) {
this.passwordEncoder = passwordEncoder;
}
/**
* The source of salts to use when decoding passwords.
* null
is a valid value, meaning the
* DaoAuthenticationProvider
will present
* null
to the relevant PasswordEncoder
.
*
* @param saltSource to use when attempting to decode passwords via the
* PasswordEncoder
*/
public void setSaltSource(SaltSource saltSource) {
this.saltSource = saltSource;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy