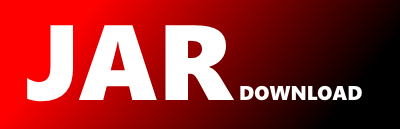
org.acegisecurity.userdetails.memory.UserMapEditor Maven / Gradle / Ivy
/* Copyright 2004, 2005 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.userdetails.memory;
import java.beans.PropertyEditorSupport;
import java.util.Iterator;
import java.util.Properties;
import org.acegisecurity.userdetails.User;
import org.acegisecurity.userdetails.UserDetails;
import org.springframework.beans.propertyeditors.PropertiesEditor;
/**
* Property editor to assist with the setup of a {@link UserMap}.
*
*
* The format of entries should be:
*
*
*
*
* username=password,grantedAuthority[,grantedAuthority][,enabled|disabled]
*
*
*
*
* The password
must always be the first entry after the equals.
* The enabled
or disabled
keyword can appear
* anywhere (apart from the first entry reserved for the password). If neither
* enabled
or disabled
appear, the default is
* enabled
. At least one granted authority must be listed.
*
*
*
* The username
represents the key and duplicates are handled the
* same was as duplicates would be in Java Properties
files.
*
*
*
* If the above requirements are not met, the invalid entry will be silently
* ignored.
*
*
*
* This editor always assumes each entry has a non-expired account and
* non-expired credentials. However, it does honour the user enabled/disabled
* flag as described above.
*
*
* @author Ben Alex
* @version $Id: UserMapEditor.java,v 1.10 2005/11/29 13:10:09 benalex Exp $
*/
public class UserMapEditor extends PropertyEditorSupport {
//~ Methods ================================================================
public void setAsText(String s) throws IllegalArgumentException {
UserMap userMap = new UserMap();
if ((s == null) || "".equals(s)) {
// Leave value in property editor null
} else {
// Use properties editor to tokenize the string
PropertiesEditor propertiesEditor = new PropertiesEditor();
propertiesEditor.setAsText(s);
Properties props = (Properties) propertiesEditor.getValue();
addUsersFromProperties(userMap, props);
}
setValue(userMap);
}
public static UserMap addUsersFromProperties(UserMap userMap,
Properties props) {
// Now we have properties, process each one individually
UserAttributeEditor configAttribEd = new UserAttributeEditor();
for (Iterator iter = props.keySet().iterator(); iter.hasNext();) {
String username = (String) iter.next();
String value = props.getProperty(username);
// Convert value to a password, enabled setting, and list of granted authorities
configAttribEd.setAsText(value);
UserAttribute attr = (UserAttribute) configAttribEd.getValue();
// Make a user object, assuming the properties were properly provided
if (attr != null) {
UserDetails user = new User(username, attr.getPassword(),
attr.isEnabled(), true, true, true,
attr.getAuthorities());
userMap.addUser(user);
}
}
return userMap;
}
}