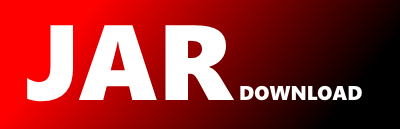
org.acegisecurity.vote.AbstractAclVoter Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.vote;
import org.acegisecurity.AuthorizationServiceException;
import org.acegisecurity.ConfigAttribute;
import org.acegisecurity.acl.AclEntry;
import org.acegisecurity.acl.AclManager;
import org.aopalliance.intercept.MethodInvocation;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.reflect.CodeSignature;
import org.springframework.util.Assert;
/**
*
* Given a domain object instance passed as a method argument, ensures the
* principal has appropriate permission as defined by the {@link AclManager}.
*
*
*
* The AclManager
is used to retrieve the access control list
* (ACL) permissions associated with a domain object instance for the current
* Authentication
object. This class is designed to process
* {@link AclEntry}s that are subclasses of {@link
* org.acegisecurity.acl.basic.BasicAclEntry} only. Generally these are
* obtained by using the {@link org.acegisecurity.acl.basic.BasicAclProvider}.
*
*
*
* The voter will vote if any {@link ConfigAttribute#getAttribute()} matches
* the {@link #processConfigAttribute}. The provider will then locate the
* first method argument of type {@link #processDomainObjectClass}. Assuming
* that method argument is non-null, the provider will then lookup the ACLs
* from the AclManager
and ensure the principal is {@link
* org.acegisecurity.acl.basic.BasicAclEntry#isPermitted(int)} for at least
* one of the {@link #requirePermission}s.
*
*
*
* If the method argument is null
, the voter will abstain from
* voting. If the method argument could not be found, an {@link
* org.acegisecurity.AuthorizationServiceException} will be thrown.
*
*
*
* In practical terms users will typically setup a number of
* BasicAclEntryVoter
s. Each will have a different {@link
* #processDomainObjectClass}, {@link #processConfigAttribute} and {@link
* #requirePermission} combination. For example, a small application might
* employ the following instances of BasicAclEntryVoter
:
*
*
* -
* Process domain object class
BankAccount
, configuration
* attribute VOTE_ACL_BANK_ACCONT_READ
, require permission
* SimpleAclEntry.READ
*
* -
* Process domain object class
BankAccount
, configuration
* attribute VOTE_ACL_BANK_ACCOUNT_WRITE
, require permission list
* SimpleAclEntry.WRITE
and SimpleAclEntry.CREATE
* (allowing the principal to have either of these two permissions
*
* -
* Process domain object class
Customer
, configuration attribute
* VOTE_ACL_CUSTOMER_READ
, require permission
* SimpleAclEntry.READ
*
* -
* Process domain object class
Customer
, configuration attribute
* VOTE_ACL_CUSTOMER_WRITE
, require permission list
* SimpleAclEntry.WRITE
and SimpleAclEntry.CREATE
*
*
*
* Alternatively, you could have used a common superclass or interface for the
* {@link #processDomainObjectClass} if both BankAccount
and
* Customer
had common parents.
*
*
*
* If the principal does not have sufficient permissions, the voter will vote
* to deny access.
*
*
*
* The AclManager
is allowed to return any implementations of
* AclEntry
it wishes. However, this provider will only be able
* to validate against AbstractBasicAclEntry
s, and thus a vote to
* deny access will be made if no AclEntry
is of type
* AbstractBasicAclEntry
.
*
*
*
* All comparisons and prefixes are case sensitive.
*
*
* @author Ben Alex
* @version $Id: AbstractAclVoter.java,v 1.4 2006/02/09 06:00:25 benalex Exp $
*/
public abstract class AbstractAclVoter implements AccessDecisionVoter {
//~ Instance fields ========================================================
private Class processDomainObjectClass;
//~ Methods ================================================================
protected Object getDomainObjectInstance(Object secureObject) {
Object[] args;
Class[] params;
if (secureObject instanceof MethodInvocation) {
MethodInvocation invocation = (MethodInvocation) secureObject;
params = invocation.getMethod().getParameterTypes();
args = invocation.getArguments();
} else {
JoinPoint jp = (JoinPoint) secureObject;
params = ((CodeSignature) jp.getStaticPart().getSignature())
.getParameterTypes();
args = jp.getArgs();
}
for (int i = 0; i < params.length; i++) {
if (processDomainObjectClass.isAssignableFrom(params[i])) {
return args[i];
}
}
throw new AuthorizationServiceException("Secure object: "
+ secureObject + " did not provide any argument of type: "
+ processDomainObjectClass);
}
public Class getProcessDomainObjectClass() {
return processDomainObjectClass;
}
public void setProcessDomainObjectClass(Class processDomainObjectClass) {
Assert.notNull(processDomainObjectClass,
"processDomainObjectClass cannot be set to null");
this.processDomainObjectClass = processDomainObjectClass;
}
/**
* This implementation supports only
* MethodSecurityInterceptor
, because it queries the
* presented MethodInvocation
.
*
* @param clazz the secure object
*
* @return true
if the secure object is
* MethodInvocation
, false
otherwise
*/
public boolean supports(Class clazz) {
if (MethodInvocation.class.isAssignableFrom(clazz)) {
return true;
} else if (JoinPoint.class.isAssignableFrom(clazz)) {
return true;
} else {
return false;
}
}
}