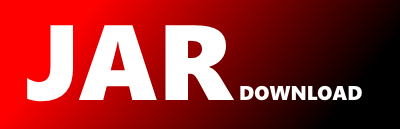
org.acegisecurity.ConfigAttributeDefinition Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity;
import java.io.Serializable;
import java.util.Iterator;
import java.util.List;
import java.util.Vector;
/**
* Holds a group of {@link ConfigAttribute}s that are associated with a given secure object target.All the
* ConfigAttributeDefinition
s associated with a given {@link
* org.acegisecurity.intercept.AbstractSecurityInterceptor} are stored in an {@link
* org.acegisecurity.intercept.ObjectDefinitionSource}.
*
* @author Ben Alex
* @version $Id: ConfigAttributeDefinition.java 1496 2006-05-23 13:38:33Z benalex $
*/
public class ConfigAttributeDefinition implements Serializable {
//~ Instance fields ================================================================================================
private List configAttributes = new Vector();
//~ Constructors ===================================================================================================
public ConfigAttributeDefinition() {
super();
}
//~ Methods ========================================================================================================
/**
* Adds a ConfigAttribute
that is related to the secure object method.
*
* @param newConfigAttribute the new configuration attribute to add
*/
public void addConfigAttribute(ConfigAttribute newConfigAttribute) {
this.configAttributes.add(newConfigAttribute);
}
/**
* Indicates whether the specified ConfigAttribute
is contained within this
* ConfigAttributeDefinition
.
*
* @param configAttribute the attribute to locate
*
* @return true
if the specified ConfigAttribute
is contained, false
* otherwise
*/
public boolean contains(ConfigAttribute configAttribute) {
return configAttributes.contains(configAttribute);
}
public boolean equals(Object obj) {
if (obj instanceof ConfigAttributeDefinition) {
ConfigAttributeDefinition test = (ConfigAttributeDefinition) obj;
List testAttrs = new Vector();
Iterator iter = test.getConfigAttributes();
while (iter.hasNext()) {
ConfigAttribute attr = (ConfigAttribute) iter.next();
testAttrs.add(attr);
}
if (this.configAttributes.size() != testAttrs.size()) {
return false;
}
for (int i = 0; i < this.configAttributes.size(); i++) {
if (!this.configAttributes.get(i).equals(testAttrs.get(i))) {
return false;
}
}
return true;
}
return false;
}
/**
* Returns an Iterator
over all the ConfigAttribute
s defined by this
* ConfigAttributeDefinition
.Allows AccessDecisionManager
s and other classes
* to loop through every configuration attribute associated with a target secure object.
*
* @return all the configuration attributes stored by the instance, or null
if an
* Iterator
is unavailable
*/
public Iterator getConfigAttributes() {
return this.configAttributes.iterator();
}
/**
* Returns the number of ConfigAttribute
s defined by this
* ConfigAttributeDefinition
.
*
* @return the number of ConfigAttribute
s contained
*/
public int size() {
return configAttributes.size();
}
public String toString() {
return this.configAttributes.toString();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy