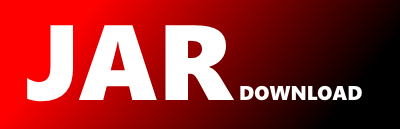
org.acegisecurity.vote.AbstractAclVoter Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.vote;
import org.acegisecurity.AuthorizationServiceException;
import org.acegisecurity.acl.AclManager;
import org.aopalliance.intercept.MethodInvocation;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.reflect.CodeSignature;
import org.springframework.util.Assert;
/**
* Given a domain object instance passed as a method argument, ensures the principal has appropriate permission
* as defined by the {@link AclManager}.
*
* @author Ben Alex
* @version $Id: AbstractAclVoter.java 1519 2006-05-29 15:06:32Z benalex $
*/
public abstract class AbstractAclVoter implements AccessDecisionVoter {
//~ Instance fields ================================================================================================
private Class processDomainObjectClass;
//~ Methods ========================================================================================================
protected Object getDomainObjectInstance(Object secureObject) {
Object[] args;
Class[] params;
if (secureObject instanceof MethodInvocation) {
MethodInvocation invocation = (MethodInvocation) secureObject;
params = invocation.getMethod().getParameterTypes();
args = invocation.getArguments();
} else {
JoinPoint jp = (JoinPoint) secureObject;
params = ((CodeSignature) jp.getStaticPart().getSignature()).getParameterTypes();
args = jp.getArgs();
}
for (int i = 0; i < params.length; i++) {
if (processDomainObjectClass.isAssignableFrom(params[i])) {
return args[i];
}
}
throw new AuthorizationServiceException("Secure object: " + secureObject
+ " did not provide any argument of type: " + processDomainObjectClass);
}
public Class getProcessDomainObjectClass() {
return processDomainObjectClass;
}
public void setProcessDomainObjectClass(Class processDomainObjectClass) {
Assert.notNull(processDomainObjectClass, "processDomainObjectClass cannot be set to null");
this.processDomainObjectClass = processDomainObjectClass;
}
/**
* This implementation supports only MethodSecurityInterceptor
, because it queries the
* presented MethodInvocation
.
*
* @param clazz the secure object
*
* @return true
if the secure object is MethodInvocation
, false
otherwise
*/
public boolean supports(Class clazz) {
if (MethodInvocation.class.isAssignableFrom(clazz)) {
return true;
} else if (JoinPoint.class.isAssignableFrom(clazz)) {
return true;
} else {
return false;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy