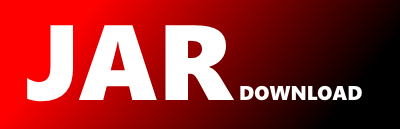
org.acegisecurity.vote.AccessDecisionVoter Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.vote;
import org.acegisecurity.Authentication;
import org.acegisecurity.ConfigAttribute;
import org.acegisecurity.ConfigAttributeDefinition;
/**
* Indicates a class is responsible for voting on authorization decisions.
*
*
* The coordination of voting (ie polling AccessDecisionVoter
s,
* tallying their responses, and making the final authorization decision) is
* performed by an {@link org.acegisecurity.AccessDecisionManager}.
*
*
* @author Ben Alex
* @version $Id: AccessDecisionVoter.java 1496 2006-05-23 13:38:33Z benalex $
*/
public interface AccessDecisionVoter {
//~ Static fields/initializers =====================================================================================
public static final int ACCESS_GRANTED = 1;
public static final int ACCESS_ABSTAIN = 0;
public static final int ACCESS_DENIED = -1;
//~ Methods ========================================================================================================
/**
* Indicates whether this AccessDecisionVoter
is able to vote on the passed
* ConfigAttribute
.This allows the AbstractSecurityInterceptor
to check every
* configuration attribute can be consumed by the configured AccessDecisionManager
and/or
* RunAsManager
and/or AfterInvocationManager
.
*
* @param attribute a configuration attribute that has been configured against the
* AbstractSecurityInterceptor
*
* @return true if this AccessDecisionVoter
can support the passed configuration attribute
*/
public boolean supports(ConfigAttribute attribute);
/**
* Indicates whether the AccessDecisionVoter
implementation is able to provide access control
* votes for the indicated secured object type.
*
* @param clazz the class that is being queried
*
* @return true if the implementation can process the indicated class
*/
public boolean supports(Class clazz);
/**
* Indicates whether or not access is granted.The decision must be affirmative
* (ACCESS_GRANTED
), negative (ACCESS_DENIED
) or the AccessDecisionVoter
* can abstain (ACCESS_ABSTAIN
) from voting. Under no circumstances should implementing classes
* return any other value. If a weighting of results is desired, this should be handled in a custom {@link
* org.acegisecurity.AccessDecisionManager} instead.
* Unless an AccessDecisionVoter
is specifically intended to vote on an access control
* decision due to a passed method invocation or configuration attribute parameter, it must return
* ACCESS_ABSTAIN
. This prevents the coordinating AccessDecisionManager
from counting
* votes from those AccessDecisionVoter
s without a legitimate interest in the access control
* decision.
* Whilst the method invocation is passed as a parameter to maximise flexibility in making access
* control decisions, implementing classes must never modify the behaviour of the method invocation (such as
* calling MethodInvocation.proceed()
).
*
* @param authentication the caller invoking the method
* @param object the secured object
* @param config the configuration attributes associated with the method being invoked
*
* @return either {@link #ACCESS_GRANTED}, {@link #ACCESS_ABSTAIN} or {@link #ACCESS_DENIED}
*/
public int vote(Authentication authentication, Object object, ConfigAttributeDefinition config);
}