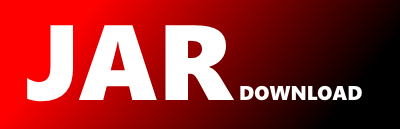
org.acegisecurity.acls.Acl Maven / Gradle / Ivy
/* Copyright 2004, 2005, 2006 Acegi Technology Pty Limited
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.acegisecurity.acls;
import org.acegisecurity.acls.objectidentity.ObjectIdentity;
import org.acegisecurity.acls.sid.Sid;
import java.io.Serializable;
/**
* Represents an access control list (ACL) for a domain object.
*
*
* An Acl
represents all ACL entries for a given domain object. In
* order to avoid needing references to the domain object itself, this
* interface handles indirection between a domain object and an ACL object
* identity via the {@link
* org.acegisecurity.acls.objectidentity.ObjectIdentity} interface.
*
*
*
* An implementation represents the {@link org.acegisecurity.acls.Permission}
* list applicable for some or all {@link org.acegisecurity.acls.sid.Sid}
* instances.
*
*
* @author Ben Alex
* @version $Id: Acl.java 1784 2007-02-24 21:00:24Z luke_t $
*/
public interface Acl extends Serializable {
//~ Methods ========================================================================================================
/**
* Returns all of the entries represented by the present Acl
(not parents).This method is
* typically used for administrative purposes.
* The order that entries appear in the array is unspecified. However, if implementations use
* particular ordering logic in authorization decisions, the entries returned by this method MUST be
* ordered in that manner.
* Do NOT use this method for making authorization decisions. Instead use {@link
* #isGranted(Permission[], Sid[], boolean)}.
* This method must operate correctly even if the Acl
only represents a subset of
* Sid
s. The caller is responsible for correctly handling the result if only a subset of
* Sid
s is represented.
*
* @return the list of entries represented by the Acl
*/
AccessControlEntry[] getEntries();
/**
* Obtains the domain object this Acl
provides entries for. This is immutable once an
* Acl
is created.
*
* @return the object identity
*/
ObjectIdentity getObjectIdentity();
/**
* Determines the owner of the Acl
. The meaning of ownership varies by implementation and is
* unspecified.
*
* @return the owner (may be null if the implementation does not use ownership concepts)
*/
Sid getOwner();
/**
* A domain object may have a parent for the purpose of ACL inheritance. If there is a parent, its ACL can
* be accessed via this method. In turn, the parent's parent (grandparent) can be accessed and so on.This
* method solely represents the presence of a navigation hierarchy between the parent Acl
and this
* Acl
. For actual inheritance to take place, the {@link #isEntriesInheriting()} must also be
* true
.
* This method must operate correctly even if the Acl
only represents a subset of
* Sid
s. The caller is responsible for correctly handling the result if only a subset of
* Sid
s is represented.
*
* @return the parent Acl
*/
Acl getParentAcl();
/**
* Indicates whether the ACL entries from the {@link #getParentAcl()} should flow down into the current
* Acl
.The mere link between an Acl
and a parent Acl
on its own
* is insufficient to cause ACL entries to inherit down. This is because a domain object may wish to have entirely
* independent entries, but maintain the link with the parent for navigation purposes. Thus, this method denotes
* whether or not the navigation relationship also extends to the actual inheritence of entries.
*
* @return true
if parent ACL entries inherit into the current Acl
*/
boolean isEntriesInheriting();
/**
* This is the actual authorization logic method, and must be used whenever ACL authorization decisions are
* required.An array of Sid
s are presented, representing security identifies of the current
* principal. In addition, an array of Permission
s is presented which will have one or more bits set
* in order to indicate the permissions needed for an affirmative authorization decision. An array is presented
* because holding any of the Permission
s inside the array will be sufficient for an
* affirmative authorization.
* The actual approach used to make authorization decisions is left to the implementation and is not
* specified by this interface. For example, an implementation MAY search the current ACL in the order
* the ACL entries have been stored. If a single entry is found that has the same active bits as are shown in a
* passed Permission
, that entry's grant or deny state may determine the authorization decision. If
* the case of a deny state, the deny decision will only be relevant if all other Permission
s passed
* in the array have also been unsuccessfully searched. If no entry is found that match the bits in the current
* ACL, provided that {@link #isEntriesInheriting()} is true
, the authorization decision may be
* passed to the parent ACL. If there is no matching entry, the implementation MAY throw an exception, or make a
* predefined authorization decision.
* This method must operate correctly even if the Acl
only represents a subset of
* Sid
s.
*
* @param permission the permission or permissions required
* @param sids the security identities held by the principal
* @param administrativeMode if true
denotes the query is for administrative purposes and no logging
* or auditing (if supported by the implementation) should be undertaken
*
* @return true
is authorization is granted
*
* @throws NotFoundException MUST be thrown if an implementation cannot make an authoritative authorization
* decision, usually because there is no ACL information for this particular permission and/or SID
* @throws UnloadedSidException thrown if the Acl
does not have details for one or more of the
* Sid
s passed as arguments
*/
boolean isGranted(Permission[] permission, Sid[] sids, boolean administrativeMode)
throws NotFoundException, UnloadedSidException;
/**
* For efficiency reasons an Acl
may be loaded and not contain entries for every
* Sid
in the system. If an Acl
has been loaded and does not represent every
* Sid
, all methods of the Sid
can only be used within the limited scope of the
* Sid
instances it actually represents.
*
* It is normal to load an Acl
for only particular Sid
s if read-only authorization
* decisions are being made. However, if user interface reporting or modification of Acl
s are
* desired, an Acl
should be loaded with all Sid
s. This method denotes whether or
* not the specified Sid
s have been loaded or not.
*
*
* @param sids one or more security identities the caller is interest in knowing whether this Sid
* supports
*
* @return true
if every passed Sid
is represented by this Acl
instance
*/
boolean isSidLoaded(Sid[] sids);
}