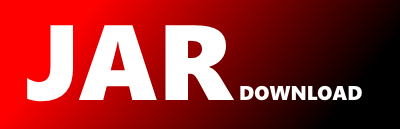
jadex.micro.philosophers.AbstractTableGui Maven / Gradle / Ivy
The newest version!
package jadex.micro.philosophers;
import java.awt.BasicStroke;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.FlowLayout;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Image;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.io.IOException;
import java.net.URL;
import java.util.function.Function;
import javax.imageio.ImageIO;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import jadex.core.IExternalAccess;
import jadex.providedservice.IProvidedServiceFeature;
public abstract class AbstractTableGui
{
protected PaintData paintdata;
public AbstractTableGui(int n)
{
paintdata = readData(n);
Image think = loadImage("think.png");
Image eat = loadImage("eat.png");
SwingUtilities.invokeLater(new Runnable()
{
public void run()
{
JFrame f = new JFrame();
JPanel p = new JPanel(new BorderLayout())
{
Rectangle[] rects = new Rectangle[n];
{
addMouseListener(new MouseAdapter()
{
public void mouseClicked(MouseEvent e)
{
// System.out.println("mouse clicked: "+e.getX()+" "+e.getY());
int x = e.getX();
int y = e.getY();
for(int i=0; i {ser.notifyPhilosopher(0); return null;});
break;
}
}
}
}
});
}
public void paint(Graphics g)
{
super.paint(g);
int w = getWidth();
int h = getHeight();
g.setColor(Color.WHITE);
g.fillOval(0, 0, w, h);
double step = 2*Math.PI/n;
for(int i=0; i ser.isWaitForClicks().get());
boolean wait = isWaitForClicks();
final JButton modeb = new JButton(wait? "Run Mode": "Click Mode");
modeb.addActionListener(new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
invertWaitForClicks();
//executeOnTable(ser -> {ser.invertWaitForClicks(); return null;});
//boolean wfc = (Boolean)executeOnTable(ser -> ser.isWaitForClicks());
boolean wfc = isWaitForClicks();
SwingUtilities.invokeLater(() ->
{
if(wfc)
{
modeb.setText("Run Mode");
}
else
{
notifyAllPhilosophers();
//executeOnTable(ser -> {ser.notifyAllPhilosophers(); return null;});
modeb.setText("Click Mode");
}
});
}
});
JPanel sp = new JPanel(new FlowLayout(FlowLayout.RIGHT));
sp.add(modeb);
f.getContentPane().add(p, BorderLayout.CENTER);
f.getContentPane().add(sp, BorderLayout.SOUTH);
f.setSize(300, 350);
f.setLocationRelativeTo(null);
f.setVisible(true);
Timer t = new Timer(100, new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
paintdata = readData(n);
p.repaint();
}
});
t.start();
}
});
}
public Image loadImage(String name)
{
Image ret = null;
try
{
URL imageUrl = AbstractTableGui.class.getResource(name);
ret = ImageIO.read(imageUrl);
}
catch (IOException e)
{
e.printStackTrace();
}
return ret;
}
public abstract PaintData readData(int n);
public abstract void notifyPhilosopher(int no, long time);
public abstract boolean isWaitForClicks();
public abstract void invertWaitForClicks();
public abstract void notifyAllPhilosophers();
public record PaintData(PhilosopherState[] state, Integer[] eatcnt, Integer[] stickowner)
{
public PaintData(int n)
{
this(new PhilosopherState[n], new Integer[n], new Integer[n]);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy