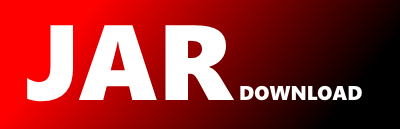
jadex.micro.philosophers.agents.PhilosophAgent Maven / Gradle / Ivy
The newest version!
package jadex.micro.philosophers.agents;
import java.util.Random;
import jadex.core.IComponent;
import jadex.execution.IExecutionFeature;
import jadex.future.Future;
import jadex.future.IFuture;
import jadex.micro.annotation.Agent;
import jadex.micro.philosophers.PhilosopherState;
import jadex.providedservice.annotation.Service;
import jadex.requiredservice.annotation.OnService;
@Agent
@Service
public class PhilosophAgent implements IPhilosopherService
{
@Agent
protected IComponent agent;
protected PhilosopherState state;
protected Random r = new Random();
protected int no;
protected ITableService t;
protected int eatcnt;
protected Future wait;
public PhilosophAgent(int no)
{
this.no = no;
this.state = PhilosopherState.THINKING;
}
@OnService
protected void foundTable(ITableService table)
{
this.t = table;
t.addPhilosopher(no);
run();
}
public void run()
{
while(true)
{
// implement the lifecycle of the philosoph here
// set the philosophers state and wait when thinking and eating
// additionally you can wait before trying to fetch the second stick
doSleepRandom();
setState(PhilosopherState.WAITING_FOR_LEFT);
t.getLeftStick(no).get();
setState(PhilosopherState.WAITING_FOR_RIGHT);
doSleepRandom();
t.getRightStick(no).get();
setState(PhilosopherState.EATING);
doSleepRandom();
t.releaseLeftStick(no);
t.releaseRightStick(no);
setState(PhilosopherState.THINKING);
eatcnt++;
}
}
public IFuture getState()
{
return new Future(state);
}
public void setState(PhilosopherState state)
{
this.state = state;
}
public IFuture getEatCnt()
{
return new Future(eatcnt);
}
public IFuture getNo()
{
return new Future(no);
}
protected void doSleepRandom()
{
doSleep((int)(Math.random()*2000));
}
protected void doSleep(long time)
{
if(!t.isWaitForClicks().get())
{
// implement time wait here
agent.getFeature(IExecutionFeature.class).waitForDelay((long)(Math.random()*2000)).get();
}
else
{
this.wait = new Future();
wait.get();
}
}
public void notifyPhilosopher(long time)
{
if(time>0)
doSleep(time);
if(wait!=null)
{
Future tmp = wait;
wait = null;
tmp.setResult(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy