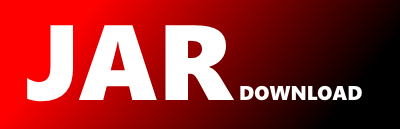
jadex.bdi.planlib.DefaultBDIVisionProcessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-applications-applib-bdi Show documentation
Show all versions of jadex-applications-applib-bdi Show documentation
The Jadex applib BDI package contains ready to use functionalities for BDI agents mostly in form of modules called capabilities.
The newest version!
package jadex.bdi.planlib;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import jadex.bdiv3x.features.IBDIXAgentFeature;
import jadex.bridge.IComponentStep;
import jadex.bridge.IExternalAccess;
import jadex.bridge.IInternalAccess;
import jadex.bridge.service.types.cms.IComponentDescription;
import jadex.commons.IValueFetcher;
import jadex.commons.SUtil;
import jadex.commons.SimplePropertyObject;
import jadex.commons.future.IFuture;
import jadex.commons.future.IResultListener;
import jadex.commons.transformation.annotations.Classname;
import jadex.extension.envsupport.environment.IEnvironmentSpace;
import jadex.extension.envsupport.environment.IPerceptProcessor;
import jadex.extension.envsupport.environment.ISpaceObject;
import jadex.extension.envsupport.environment.space2d.Space2D;
import jadex.extension.envsupport.math.IVector1;
import jadex.extension.envsupport.math.IVector2;
import jadex.extension.envsupport.math.Vector1Double;
import jadex.javaparser.IParsedExpression;
import jadex.javaparser.SimpleValueFetcher;
/**
* Default bdi agent vision processor.
* Updates the agent's beliefsets according to the percepts of new/disappeared waste.
*/
public class DefaultBDIVisionProcessor extends SimplePropertyObject implements IPerceptProcessor
{
//-------- constants --------
/** The percept types property. */
public static final String PROPERTY_PERCEPTTYPES = "percepttypes";
/** The add action. */
public static final String ADD = "add";
/** The remove action. */
public static final String REMOVE = "remove";
/** The remove_outdated action (checks all entries in the belief set, if they should be seen, but are no longer there). */
public static final String REMOVE_OUTDATED = "remove_outdated";
/** The set action. */
public static final String SET = "set";
/** The unset action (sets a belief fact to null). */
public static final String UNSET = "unset";
/** The maxrange property. */
public static final String PROPERTY_MAXRANGE = "range";
/** The maxrange property. */
public static final String PROPERTY_RANGE = "range_property";
//-------- attributes --------
/** The percepttypes infos. */
protected Map percepttypes;
//-------- methods --------
/**
* Process a new percept.
* @param space The space.
* @param type The type.
* @param percept The percept.
* @param agent The agent identifier.
* @param agent The avatar of the agent (if any).
*/
public void processPercept(final IEnvironmentSpace space, final String type, final Object percept, final IComponentDescription agent, final ISpaceObject avatar)
{
boolean invoke = false;
final String[][] metainfos = getMetaInfos(type);
for(int i=0; !invoke && metainfos!=null && i fut = space.getExternalAccess().getExternalAccessAsync(agent.getName());
fut.addResultListener(new IResultListener()
{
public void exceptionOccurred(Exception exception)
{
// Happens when component already removed
// exception.printStackTrace();
}
public void resultAvailable(IExternalAccess result)
{
final IExternalAccess exta = (IExternalAccess)result;
for(int i=0; i()
{
@Classname("add")
public IFuture execute(IInternalAccess ia)
{
IBDIXAgentFeature bdif = ia.getFeature(IBDIXAgentFeature.class);
Object[] facts = bdif.getBeliefbase().getBeliefSet(name).getFacts();
if(cond!=null)
fetcher.setValue("$facts", facts);
if(!SUtil.arrayContains(facts, percept) && (cond==null || evaluate(cond, fetcher)))
{
bdif.getBeliefbase().getBeliefSet(name).addFact(percept);
// System.out.println("added: "+percept+" to: "+belset);
}
return IFuture.DONE;
}
});
}
else if(REMOVE.equals(metainfos[i][0]))
{
exta.scheduleStep(new IComponentStep()
{
@Classname("remove")
public IFuture execute(IInternalAccess ia)
{
IBDIXAgentFeature bdif = ia.getFeature(IBDIXAgentFeature.class);
Object[] facts = bdif.getBeliefbase().getBeliefSet(name).getFacts();
if(cond!=null)
fetcher.setValue("$facts", facts);
if(SUtil.arrayContains(facts, percept) && (cond==null || evaluate(cond, fetcher)))
{
bdif.getBeliefbase().getBeliefSet(name).removeFact(percept);
// System.out.println("removed: "+percept+" from: "+belset);
}
return IFuture.DONE;
}
});
}
else if(SET.equals(metainfos[i][0]))
{
exta.scheduleStep(new IComponentStep()
{
@Classname("set")
public IFuture execute(IInternalAccess ia)
{
IBDIXAgentFeature bdif = ia.getFeature(IBDIXAgentFeature.class);
Object fact = bdif.getBeliefbase().getBelief(name).getFact();
if(cond!=null)
fetcher.setValue("$fact", fact);
if(cond==null || evaluate(cond, fetcher))
{
bdif.getBeliefbase().getBelief(name).setFact(percept);
// System.out.println("set: "+percept+" on: "+belset);
}
return IFuture.DONE;
}
});
}
else if(UNSET.equals(metainfos[i][0]))
{
exta.scheduleStep(new IComponentStep()
{
@Classname("unset")
public IFuture execute(IInternalAccess ia)
{
IBDIXAgentFeature bdif = ia.getFeature(IBDIXAgentFeature.class);
Object fact = bdif.getBeliefbase().getBelief(name).getFact();
if(cond!=null)
fetcher.setValue("$fact", fact);
if(cond==null || evaluate(cond, fetcher))
{
bdif.getBeliefbase().getBelief(name).setFact(null);
// System.out.println("unset: "+percept+" on: "+belset);
}
return IFuture.DONE;
}
});
}
else if(REMOVE_OUTDATED.equals(metainfos[i][0]) && percept.equals(avatar))
{
exta.scheduleStep(new IComponentStep()
{
@Classname("removeoutdated")
public IFuture execute(IInternalAccess ia)
{
IBDIXAgentFeature bdif = ia.getFeature(IBDIXAgentFeature.class);
Object[] facts = bdif.getBeliefbase().getBeliefSet(name).getFacts();
if(cond!=null)
fetcher.setValue("$facts", facts);
if(cond==null || evaluate(cond, fetcher))
{
IVector1 vision = getRange(avatar);
Space2D space2d = (Space2D)space;
IVector2 mypos = (IVector2)avatar.getProperty(Space2D.PROPERTY_POSITION);
ISpaceObject[] known = (ISpaceObject[])facts;
Set seen = space2d.getNearObjects(mypos, vision);
for(int j=0; j
© 2015 - 2025 Weber Informatics LLC | Privacy Policy