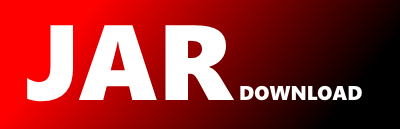
jadex.commons.gui.ClassSearchPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-commons-gui Show documentation
Show all versions of jadex-commons-gui Show documentation
Common GUI support classes.
package jadex.commons.gui;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.FlowLayout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.io.File;
import java.lang.reflect.Modifier;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.StringTokenizer;
import java.util.jar.JarEntry;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.swing.AbstractAction;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JComponent;
import javax.swing.JDialog;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextField;
import javax.swing.KeyStroke;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
import javax.swing.UIDefaults;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.DefaultTableCellRenderer;
import jadex.commons.IFilter;
import jadex.commons.SReflect;
import jadex.commons.SUtil;
import jadex.commons.concurrent.IThreadPool;
import jadex.commons.concurrent.ThreadPool;
import jadex.commons.future.IIntermediateResultListener;
import jadex.commons.future.ISubscriptionIntermediateFuture;
import jadex.commons.future.ITerminationCommand;
import jadex.commons.future.TerminableFuture;
import jadex.commons.gui.future.SwingIntermediateResultListener;
/**
* Panel that allows for searching artifacts from maven repositories.
*/
public class ClassSearchPanel extends JPanel
{
/** The icons. */
protected static final UIDefaults icons = new UIDefaults(new Object[]
{
"class", SGUI.makeIcon(ClassSearchPanel.class, "/jadex/commons/gui/images/class.png"),
"abstractclass", SGUI.makeIcon(ClassSearchPanel.class, "/jadex/commons/gui/images/abstractclass.png"),
"interface", SGUI.makeIcon(ClassSearchPanel.class, "/jadex/commons/gui/images/interface.png")
});
//-------- attributes --------
/** The results table. */
protected JTable results;
protected ClassTableModel ctm;
/** The status. */
protected JLabel status;
/** The thread pool. */
protected IThreadPool tp;
/** The classloader. */
protected ClassLoader cl;
/** The current search query text. */
protected String curquery;
/** The checkbox for interfaces. */
protected JCheckBox cbif;
protected JCheckBox cbac;
protected JCheckBox cbc;
protected JCheckBox cbic;
//-------- constructors --------
/**
* Create a new search panel.
*/
public ClassSearchPanel(ClassLoader cl, IThreadPool tp)
{
this(cl, tp, true, true, true, false);
}
/**
* Create a new search panel.
*/
public ClassSearchPanel(ClassLoader cl, IThreadPool tp,
boolean interfaces, boolean absclasses, boolean classes, boolean innerclasses)
{
this.tp = tp==null? new ThreadPool(): tp;
final JTextField tfsearch = new JTextField();
tfsearch.addKeyListener(new KeyListener()
{
protected boolean dirty = false;
protected Timer t;
{
// Swing timer
t = new Timer(500, new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
if(dirty)
{
dirty = false;
}
else
{
t.stop();
performSearch(tfsearch.getText());
}
}
});
}
public void keyTyped(KeyEvent e)
{
if(!t.isRunning())
{
t.start();
}
else
{
dirty = true;
}
}
public void keyReleased(KeyEvent e)
{
}
public void keyPressed(KeyEvent e)
{
}
});
status = new JLabel("idle");
ctm = new ClassTableModel();
results = new JTable(ctm);
results.setDefaultRenderer(ClassInfo.class, new ClassCellRenderer());
results.setTableHeader(null);
cbif = new JCheckBox("Interfaces", interfaces);
cbac = new JCheckBox("Abstract Classes", absclasses);
cbc = new JCheckBox("Classes", classes);
cbic = new JCheckBox("Inner Classes", innerclasses);
ActionListener al = new ActionListener()
{
public void actionPerformed(ActionEvent e)
{
performSearch(tfsearch.getText());
}
};
cbif.addActionListener(al);
cbac.addActionListener(al);
cbc.addActionListener(al);
cbic.addActionListener(al);
JPanel cbp = new JPanel(new FlowLayout(FlowLayout.LEFT));
cbp.add(cbif);
cbp.add(cbac);
cbp.add(cbc);
cbp.add(cbic);
setLayout(new GridBagLayout());
int y=0;
add(new JLabel("Enter type name prefix:"), new GridBagConstraints(0,y++,
2,1,0,0,GridBagConstraints.NORTHWEST, GridBagConstraints.VERTICAL, new Insets(2,2,2,2),0,0));
add(tfsearch, new GridBagConstraints(0,y++,2,1,1,0,
GridBagConstraints.NORTH, GridBagConstraints.BOTH, new Insets(2,2,2,2),0,0));
add(cbp, new GridBagConstraints(0,y++,
2,1,0,0,GridBagConstraints.NORTHWEST, GridBagConstraints.HORIZONTAL, new Insets(2,2,2,2),0,0));
add(new JLabel("Search Results:"), new GridBagConstraints(0,y++,
2,1,0,0,GridBagConstraints.NORTHWEST, GridBagConstraints.VERTICAL, new Insets(2,2,2,2),0,0));
add(new JScrollPane(results), new GridBagConstraints(0,y++,
2,1,1,1,GridBagConstraints.NORTHWEST, GridBagConstraints.BOTH, new Insets(2,2,2,2),0,0));
add(status, new GridBagConstraints(0,y++,
2,1,1,0,GridBagConstraints.NORTHWEST, GridBagConstraints.BOTH, new Insets(2,2,2,2),0,0));
performSearch(null);
// tp.execute(new Runnable()
// {
// public void run()
// {
// while(true)
// {
// try
// {
// Thread.sleep(1000);
// getSelectedArtifactInfo();
// }
// catch(Exception e)
// {
// e.printStackTrace();
// }
// }
// }
// });
}
//-------- methods --------
/**
* Get the thread pool.
* @return The thread pool.
*/
public IThreadPool getThreadPool()
{
return tp;
}
/**
* Set the current query string.
* Used to abort old searches.
* @param curquery The current query.
*/
protected synchronized void setCurrentQuery(String curquery)
{
this.curquery = curquery;
}
/**
* Test if the search is still the current one.
* @param curquery The current search text.
*/
protected synchronized boolean isCurrentQuery(String curquery)
{
return SUtil.equals(this.curquery, curquery);
}
protected TerminableFuture lastsearch;
/**
* Perform a search using a search expression.
*/
public void performSearch(final String exp)
{
assert SwingUtilities.isEventDispatchThread();
final ISubscriptionIntermediateFuture>[] fut = new ISubscriptionIntermediateFuture[1];
if(lastsearch!=null)
lastsearch.terminate();
final TerminableFuture ret = new TerminableFuture(new ITerminationCommand()
{
public void terminated(Exception reason)
{
// System.out.println("terminating: "+exp);
if(fut[0]!=null)
fut[0].terminate();
}
public boolean checkTermination(Exception reason)
{
return true;
}
});
lastsearch = ret;
if(exp==null || exp.length()==0)
{
status.setText("idle");
lastsearch.setResultIfUndone(null);
return;
}
final Pattern pat = SUtil.createRegexFromGlob(exp+"*");
setCurrentQuery(exp);
// System.out.println("perform search: "+exp);
// ctm.clear();
if(exp!=null && exp.length()>0)
status.setText("searching '"+exp+"'");
getThreadPool().execute(new Runnable()
{
public void run()
{
IFilter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy