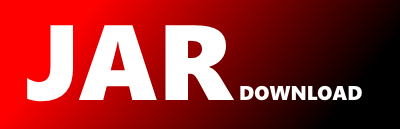
jadex.commons.gui.EditableList Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-commons-gui Show documentation
Show all versions of jadex-commons-gui Show documentation
Common GUI support classes.
package jadex.commons.gui;
import java.awt.Component;
import java.awt.Insets;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import javax.swing.AbstractCellEditor;
import javax.swing.JButton;
import javax.swing.JTable;
import javax.swing.UIDefaults;
import javax.swing.event.TableModelEvent;
import javax.swing.table.AbstractTableModel;
import javax.swing.table.TableCellEditor;
import javax.swing.table.TableCellRenderer;
import jadex.commons.SUtil;
/**
* A editable list with x / + symbols at each row.
*/
public class EditableList extends JTable
{
//-------- static part --------
/** The image icons. */
protected static final UIDefaults icons = new UIDefaults(new Object[]
{
"add", SGUI.makeIcon(EditableList.class, "/jadex/commons/gui/images/add_small.png"),
"delete", SGUI.makeIcon(EditableList.class, "/jadex/commons/gui/images/delete_small.png"),
});
//-------- attributes --------
/** The editable flag. */
protected boolean editable;
/** The entries. */
protected java.util.List entries;
/** Allow duplicate entries. */
protected boolean allowduplicates;
/** The list title. */
protected String title;
/** Show the numbers of entries in title. */
protected boolean showcnt;
//-------- constructors --------
/**
* Create a new editable list.
*/
public EditableList(final String title)
{
this(title, false);
}
/**
* Create a new editable list.
*/
public EditableList(final String title, boolean showcnt)
{
this.title = title;
this.entries = new ArrayList();
this.editable = true;
this.allowduplicates = false;
this.showcnt = showcnt;
setModel(new AbstractTableModel()
{
public int getColumnCount()
{
return editable ? 2 : 1;
}
public String getColumnName(int column)
{
return editable && column==0 ? " " : getTitle();
}
public Class getColumnClass(int columnIndex)
{
return editable && columnIndex==0 ? JButton.class : String.class;
}
public int getRowCount()
{
return getEntries().length + (editable ? 1 : 0);
}
public Object getValueAt(int rowIndex, int columnIndex)
{
if((!editable || columnIndex!=0) && rowIndex=entries.size())
entries.add("");
entries.set(rowIndex, (String)newadr);
fireTableCellUpdated(rowIndex, columnIndex);
}
}
});
// Hack!!! Set header preferred size and afterwards set title text to "" (bug in JDK1.5).
getTableHeader().setPreferredSize(getTableHeader().getPreferredSize());
getColumnModel().getColumn(0).setHeaderValue("");
// Hack!!! Stupid JTable behaviour, see bug #4709394.
putClientProperty("terminateEditOnFocusLost", Boolean.TRUE);
setDefaultRenderer(JButton.class, new ButtonCellManager());
setDefaultEditor(JButton.class, new ButtonCellManager());
JButton but = new JButton(icons.getIcon("delete"));
but.setMargin(new Insets(0,0,0,0));
getColumnModel().getColumn(0).setMaxWidth(but.getPreferredSize().width);
//teststable.setPreferredScrollableViewportSize(new Dimension(tfname.getPreferredSize().width, tfname.getPreferredSize().height*3));
// Add resizable header.
/*ResizeableTableHeader header = new ResizeableTableHeader();
header.setColumnModel(getColumnModel());
header.setAutoResizingEnabled(true); //default
header.setIncludeHeaderWidth(false); //default
setTableHeader(header);
setAutoResizeMode(JTable.AUTO_RESIZE_OFF);*/
}
/**
* Test if duplicates are allowed.
* @return the allowduplicates.
*/
public boolean isAllowDuplicates()
{
return allowduplicates;
}
/**
* Set if duplicates are allowed.
* @param allowduplicates the allowduplicates to set.
*/
public void setAllowDuplicates(boolean allowduplicates)
{
this.allowduplicates = allowduplicates;
if(!allowduplicates)
removeDuplicates();
}
/**
* Remove all duplicates from the list.
*/
protected void removeDuplicates()
{
for(int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy