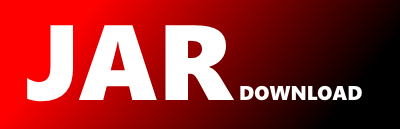
jadex.bdiv3.features.impl.BDIProvidedServicesComponentFeature Maven / Gradle / Ivy
package jadex.bdiv3.features.impl;
import java.lang.reflect.Proxy;
import java.util.HashSet;
import java.util.Set;
import jadex.bdiv3.IBDIAgent;
import jadex.bdiv3.features.IBDIAgentFeature;
import jadex.bdiv3.model.MElement;
import jadex.bdiv3.runtime.impl.BDIServiceInvocationHandler;
import jadex.bdiv3.runtime.impl.CapabilityPojoWrapper;
import jadex.bridge.IInternalAccess;
import jadex.bridge.component.ComponentCreationInfo;
import jadex.bridge.component.IPojoComponentFeature;
import jadex.bridge.service.ProvidedServiceImplementation;
import jadex.bridge.service.ProvidedServiceInfo;
import jadex.bridge.service.component.ProvidedServicesComponentFeature;
import jadex.commons.IParameterGuesser;
import jadex.commons.IValueFetcher;
import jadex.commons.SimpleParameterGuesser;
import jadex.javaparser.SimpleValueFetcher;
/**
* Overriden to allow for service implementations to be directly mapped to plans.
*/
public class BDIProvidedServicesComponentFeature extends ProvidedServicesComponentFeature
{
//-------- constructors --------
/**
* Factory method constructor for instance level.
*/
public BDIProvidedServicesComponentFeature(IInternalAccess component, ComponentCreationInfo cinfo)
{
super(component, cinfo);
}
/**
* Init a service.
* Overriden to allow for service implementations as BPMN processes using signal events.
*/
protected Object createServiceImplementation(ProvidedServiceInfo info, IValueFetcher fetcher) throws Exception
{
// todo: cleanup this HACK!!!
if(getComponent().getComponentFeature0(IPojoComponentFeature.class)!=null)
{
int i = info.getName()!=null ? info.getName().indexOf(MElement.CAPABILITY_SEPARATOR) : -1;
Object ocapa = getComponent().getComponentFeature(IPojoComponentFeature.class).getPojoAgent();
String capa = null;
if(i!=-1)
{
capa = info.getName().substring(0, i);
SimpleValueFetcher fet = new SimpleValueFetcher(fetcher);
ocapa = ((BDIAgentFeature)getComponent().getComponentFeature(IBDIAgentFeature.class)).getCapabilityObject(capa);
fet.setValue("$pojocapa", ocapa);
fetcher = fet;
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy