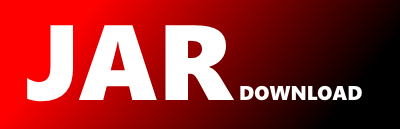
jadex.bdiv3.runtime.IPlan Maven / Gradle / Ivy
package jadex.bdiv3.runtime;
import jadex.bdiv3x.runtime.IFinishableElement;
import jadex.bdiv3x.runtime.IParameterElement;
import jadex.commons.IFilter;
import jadex.commons.future.IFuture;
import jadex.rules.eca.ChangeInfo;
import jadex.rules.eca.ICondition;
/**
* User interface for plans.
*/
public interface IPlan extends IParameterElement, IFinishableElement
© 2015 - 2024 Weber Informatics LLC | Privacy Policy