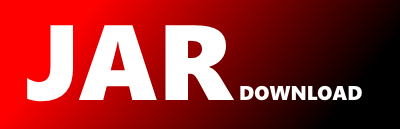
jadex.bdiv3.runtime.impl.ServiceCallPlan Maven / Gradle / Ivy
package jadex.bdiv3.runtime.impl;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import jadex.bdiv3.annotation.Plan;
import jadex.bdiv3.annotation.PlanBody;
import jadex.bdiv3.annotation.PlanReason;
import jadex.bdiv3.features.impl.BDIAgentFeature;
import jadex.bridge.IInternalAccess;
import jadex.bridge.service.RequiredServiceInfo;
import jadex.bridge.service.component.IRequiredServicesFeature;
import jadex.commons.SReflect;
import jadex.commons.future.Future;
import jadex.commons.future.IFuture;
import jadex.commons.future.IIntermediateFuture;
import jadex.commons.future.IIntermediateResultListener;
import jadex.commons.future.IResultListener;
/**
* Default plan for realizing a service call.
* Finds and calls a service.
*/
@Plan
public class ServiceCallPlan
{
@PlanReason
protected Object reason;
// @PlanCapability
protected IInternalAccess agent;
/** The service name. */
protected String service;
/** The method. */
protected String method;
/** The parameter service mapper. */
protected IServiceParameterMapper
© 2015 - 2024 Weber Informatics LLC | Privacy Policy