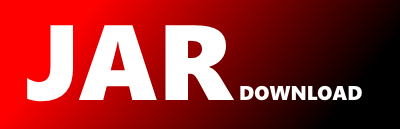
jadex.micro.MicroModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-kernel-micro Show documentation
Show all versions of jadex-kernel-micro Show documentation
The Jadex micro kernel provides a lightweight and very efficient kernel for minimal agents with an extremely low memory footprint. Micro agents have a simple internal architecture that is similar to active objects. They are programmed as Java objects overriding a framework class, which has predefined methods for the setup, termination, execution logic as well as message receipt.
package jadex.micro;
import java.lang.annotation.Annotation;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import jadex.bridge.ClassInfo;
import jadex.bridge.ServiceCallInfo;
import jadex.bridge.modelinfo.IModelInfo;
import jadex.commons.FieldInfo;
import jadex.commons.MethodInfo;
import jadex.commons.SUtil;
import jadex.commons.Tuple2;
import jadex.commons.Tuple3;
import jadex.commons.collection.MultiCollection;
import jadex.kernelbase.CacheableKernelModel;
/**
* The micro agent model.
*/
public class MicroModel extends CacheableKernelModel
{
/** The micro agent class. */
protected ClassInfo pojoclass;
/** The agent injection targets. */
protected List agentinjections;
/** The parent injection targets. */
protected List parentinjections;
/** The argument injection targets. */
protected MultiCollection> argumentinjections;
/** The result injection targets. */
protected Map> resultinjections;
/** The service injection targets. */
protected MultiCollection serviceinjections;
/** The feature injection targets. */
protected List featureinjections;
/** The class loader. */
protected ClassLoader classloader;
/** The agent methods for given annotations (if any). */
protected Map, MethodInfo> agentmethods;
/** The service value calls. */
protected List servicecalls;
/**
* Create a new model.
*/
public MicroModel(IModelInfo modelinfo)
{
super(modelinfo);
}
/**
* Add an injection field.
* @param field The field.
*/
public void addAgentInjection(FieldInfo field)
{
if(agentinjections==null)
agentinjections = new ArrayList();
agentinjections.add(field);
}
/**
* Get the agent injection fields.
* @return The fields.
*/
public FieldInfo[] getAgentInjections()
{
return agentinjections==null? new FieldInfo[0]: (FieldInfo[])agentinjections.toArray(new FieldInfo[agentinjections.size()]);
}
/**
* Add an injection field.
* @param field The field.
*/
public void addParentInjection(FieldInfo field)
{
if(parentinjections==null)
parentinjections = new ArrayList();
parentinjections.add(field);
}
/**
* Get the parentinjections fields.
* @return The fields.
*/
public FieldInfo[] getParentInjections()
{
return parentinjections==null? new FieldInfo[0]: (FieldInfo[])parentinjections.toArray(new FieldInfo[parentinjections.size()]);
}
/**
* Add an injection field.
* @param name The name.
* @param field The field.
*/
public void addArgumentInjection(String name, FieldInfo field, String convert)
{
if(argumentinjections==null)
argumentinjections = new MultiCollection>();
argumentinjections.add(name, new Tuple2(field, convert!=null && convert.length()==0? null: convert));
}
/**
* Get the argument injection fields.
* @return The fields.
*/
public Tuple2[] getArgumentInjections(String name)
{
Collection col = argumentinjections==null? null: (Collection)argumentinjections.get(name);
return col==null? new Tuple2[0]: (Tuple2[])col.toArray(new Tuple2[col.size()]);
}
/**
* Get the argument injection names.
* @return The names.
*/
public String[] getArgumentInjectionNames()
{
return argumentinjections==null? SUtil.EMPTY_STRING_ARRAY:
(String[])argumentinjections.keySet().toArray(new String[argumentinjections.size()]);
}
/**
* Add an injection field.
* @param name The name.
* @param field The field.
*/
public void addResultInjection(String name, FieldInfo field, String convert, String convback)
{
if(resultinjections==null)
resultinjections = new HashMap>();
resultinjections.put(name, new Tuple3(field,
convert!=null && convert.length()==0? null: convert,
convback!=null && convback.length()==0? null: convback));
}
/**
* Get the result injection field.
* @return The fields.
*/
public Tuple3 getResultInjection(String name)
{
return resultinjections==null? null: (Tuple3)resultinjections.get(name);
}
/**
* Get the Result injection names.
* @return The names.
*/
public String[] getResultInjectionNames()
{
return resultinjections==null? SUtil.EMPTY_STRING_ARRAY:
(String[])resultinjections.keySet().toArray(new String[resultinjections.size()]);
}
/**
* Add an injection field.
* @param name The name.
* @param field The field.
*/
public void addServiceInjection(String name, FieldInfo field)
{
if(serviceinjections==null)
serviceinjections = new MultiCollection();
serviceinjections.add(name, field);
}
/**
* Add an injection field.
* @param name The name.
* @param method The method.
*/
public void addServiceInjection(String name, MethodInfo method)
{
if(serviceinjections==null)
serviceinjections = new MultiCollection();
serviceinjections.add(name, method);
}
/**
* Get the service injection fields.
* @return The field or method infos.
*/
public Object[] getServiceInjections(String name)
{
Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy