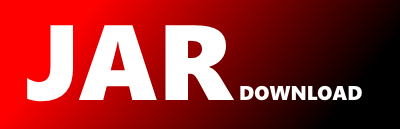
jadex.base.relay.JadexRelayExample Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-platform-extension-relay Show documentation
Show all versions of jadex-platform-extension-relay Show documentation
Base classes for Jadex relay server.
package jadex.base.relay;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.io.UnsupportedEncodingException;
import java.net.HttpURLConnection;
import java.net.URL;
import java.net.URLEncoder;
import java.util.Random;
/**
* Example showing how to connect to Jadex relay server using pure Java only
* (no Jadex jars required).
* It is recommended using the helper methods of RelayConnectionManager instead.
*/
public class JadexRelayExample
{
//-------- example code below --------
/**
* Main method for testing.
*/
public static void main(String[] args) throws Exception
{
// Create a random id, which is used for sending message to.
String id = "RelayExample_"+new Random().nextInt(1000);
// Connect to server (starts receiver thread in background).
connect(id);
// Send a message to self.
send(id, "Hello Relay World!".getBytes("UTF-8"));
send(id, "some more testing...".getBytes("UTF-8"));
// Wait while received message is printed on receiver thread.
Thread.sleep(1000);
// Exit
System.exit(0);
}
/**
* Called whenever a message is received.
*/
public static void deliverMessage(byte[] rawmsg)
{
try
{
System.out.println("Message received: "+new String(rawmsg, "UTF-8"));
}
catch (UnsupportedEncodingException e)
{
throw new RuntimeException(e);
}
}
//-------- helper code below (need not be touched) --------
/** The relay server address. */
public static final String ADDRESS = "http://www.activecomponents.org/relay/";
/** The default message type: followed by length (int as 4 bytes) and arbitrary message content from some sender. */
public static final byte MSGTYPE_DEFAULT = 1;
/** The ping message type sent by server to verify if connection is alive (just the type byte and no content). */
public static final byte MSGTYPE_PING = 2;
/**
* Start a new thread and connect to the relay server.
*/
public static void connect(String id) throws Exception
{
// Connect to server.
URL url = new URL(ADDRESS+"?id="+URLEncoder.encode(id, "UTF-8"));
HttpURLConnection con = (HttpURLConnection)url.openConnection();
con.setUseCaches(false);
final InputStream in = con.getInputStream();
int read;
while((read=in.read())!=-1 && read!=MSGTYPE_PING)
{
// wait for first ping.
System.out.println(read);
}
// Start receiver thread.
new Thread(new Runnable()
{
public void run()
{
try
{
while(true)
{
// Read message type.
int b = in.read();
if(b==-1)
{
throw new IOException("Stream closed");
}
else if(b==MSGTYPE_PING)
{
System.out.println("Received server ping");
}
else if(b==MSGTYPE_DEFAULT)
{
byte[] rawmsg = null;
// Read message header (size)
int msg_size;
byte[] asize = new byte[4];
for(int i=0; i0)
{
rawmsg = new byte[msg_size];
int count = 0;
while(count>> 24) & 0xFF);
buffer[1] = (byte)((val >>> 16) & 0xFF);
buffer[2] = (byte)((val >>> 8) & 0xFF);
buffer[3] = (byte)(val & 0xFF);
return buffer;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy