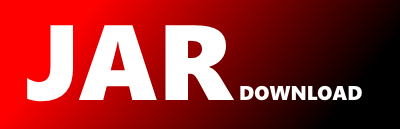
org.activecomponents.webservice.SWebSocket Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-platform-webservice-websocket Show documentation
Show all versions of jadex-platform-webservice-websocket Show documentation
Jadex websocket webservice implementation
package org.activecomponents.webservice;
import java.util.Enumeration;
import java.util.Map;
import javax.servlet.ServletContext;
import jadex.base.IPlatformConfiguration;
import jadex.base.PlatformConfigurationHandler;
import jadex.base.Starter;
import jadex.bridge.IComponentIdentifier;
import jadex.bridge.IExternalAccess;
import jadex.bridge.service.types.cms.CreationInfo;
import jadex.commons.future.ExceptionDelegationResultListener;
import jadex.commons.future.Future;
import jadex.commons.future.FutureBarrier;
import jadex.commons.future.IFuture;
import jadex.commons.future.IResultListener;
/**
* Static helper methods for Jadex websocket integration.
*/
public class SWebSocket
{
//-------- constants --------
/** Attribute name for platform in context. */
public static final String ATTR_PLATFORM = "org.activecomponents.webservice.platform";
//-------- methods --------
/**
* Initialize a context. Should be called only once per context at startup.
* Creates platform and initial agents, if necessary.
* @param context The servlet context.
*/
public static IFuture initContext(ServletContext context)
{
System.out.println("Init context for: "+context.getContextPath());
FutureBarrier agents = new FutureBarrier();
// Create components specified in web.xml
Enumeration pnames = context.getInitParameterNames();
if(pnames!=null)
{
while(pnames.hasMoreElements())
{
String pname = pnames.nextElement();
if(pname!=null && pname.startsWith("ws_component"))
{
final String model = context.getInitParameter(pname);
IFuture fut = createAgent(context, model);
agents.addFuture(fut);
fut.addResultListener(new IResultListener()
{
public void resultAvailable(IComponentIdentifier result)
{
}
public void exceptionOccurred(Exception exception)
{
System.out.println("Exception in component start: "+model+" "+exception);
}
});
}
}
}
return agents.waitForIgnoreFailures(null);
}
/**
* Cleanup a context. Should be called only once per context at shutdown.
* Removes platform with all agents, if necessary.
* @param context The servlet context.
*/
public static IFuture cleanupContext(ServletContext context)
{
// Terminates the platform of the application/context
IFuture fut = (IFuture)context.getAttribute(ATTR_PLATFORM);
if(fut!=null)
{
final Future ret = new Future();
fut.addResultListener(new IResultListener()
{
@Override
public void resultAvailable(IExternalAccess platform)
{
platform.killComponent().addResultListener(new IResultListener
© 2015 - 2024 Weber Informatics LLC | Privacy Policy