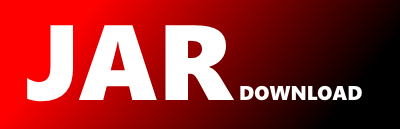
jadex.rules.tools.stateviewer.CopyState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-rules-tools Show documentation
Show all versions of jadex-rules-tools Show documentation
The Jadex rules tools package contains tools for the Jadex rule engine.
package jadex.rules.tools.stateviewer;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.IdentityHashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import jadex.commons.collection.IdentityHashSet;
import jadex.commons.concurrent.ISynchronizator;
import jadex.rules.state.IOAVState;
import jadex.rules.state.IOAVStateListener;
import jadex.rules.state.IProfiler;
import jadex.rules.state.OAVAttributeType;
import jadex.rules.state.OAVJavaType;
import jadex.rules.state.OAVObjectType;
import jadex.rules.state.OAVTypeModel;
/**
* A copy state allows to decouple a state
* from the user (e.g. a local or remote gui tool).
* Changes to the original state will be represented
* in the copy state, but synchronized to a user
* environment (e.g. swing thread).
* Therefore, a synchronizator needs to be set.
*/
public class CopyState implements IOAVState
{
//-------- attributes --------
/** The state copy. */
protected Map copy;
/** The typemodel. */
protected OAVTypeModel tmodel;
/** The root objects. */
protected Set rootobjects;
/** The synchronizator (e.g. swing sync.). */
protected ISynchronizator synchronizator;
/** The original state. */
protected IOAVState state;
/** The listener on the original state. */
protected IOAVStateListener listener;
/** The listeners. */
protected List listeners;
//-------- constructors --------
/**
* Create a local copy state.
* @param state The original state.
* @param synchronizator The synchronizator used to reflect changes in the copy state.
*/
public CopyState(final IOAVState state, ISynchronizator synchronizator)
{
this.state = state;
this.copy = state.isJavaIdentity() ? (Map)new IdentityHashMap() : new HashMap();
this.rootobjects = state.isJavaIdentity() ? (Set)new IdentityHashSet() : new HashSet();
setSynchronizator(synchronizator);
// Copy initial objects.
this.tmodel = state.getTypeModel();
for(Iterator it=state.getDeepObjects(); it.hasNext(); )
{
Object id = it.next();
OAVObjectType type = state.getType(id);
if(!(type instanceof OAVJavaType))
{
Map obj = copyObject(state, id, type);
copy.put(id, obj);
}
else
{
copy.put(id, id);
}
}
for(Iterator it=state.getRootObjects(); it.hasNext(); )
{
rootobjects.add(it.next());
}
// Add listener for updating on changes.
listener = new IOAVStateListener()
{
public void objectModified(final Object id, final OAVObjectType type,
final OAVAttributeType attr, final Object oldvalue, final Object newvalue)
{
if(!(type instanceof OAVJavaType))
{
// For multiplicity always copy complete collection (hack???)
Object val = newvalue;
if(!OAVAttributeType.NONE.equals(attr.getMultiplicity()))
{
Collection coll = state.getAttributeValues(id, attr);
if(coll!=null)
{
coll = new ArrayList(coll);
}
val = coll;
}
final Object newval = val;
CopyState.this.synchronizator.invokeLater(new Runnable()
{
public void run()
{
Map obj = (Map)copy.get(id);
assert obj!=null : id;
obj.put(attr, newval);
if(listeners!=null)
{
IOAVStateListener[] alist = (IOAVStateListener[])listeners.toArray(new IOAVStateListener[listeners.size()]);
for(int i=0; i
© 2015 - 2024 Weber Informatics LLC | Privacy Policy