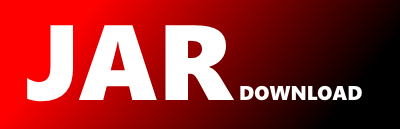
jadex.base.gui.JAutoPositionDialog Maven / Gradle / Ivy
Show all versions of jadex-tools-base-swing Show documentation
package jadex.base.gui;
import java.awt.Dialog;
import java.awt.Frame;
import java.awt.GraphicsConfiguration;
import java.awt.HeadlessException;
import javax.swing.JComponent;
import javax.swing.JDialog;
import jadex.commons.gui.SGUI;
/**
* Dialog that positions itself in the middle of the screen.
*/
public class JAutoPositionDialog extends JDialog
{
/**
* Creates a non-modal dialog without a title and without a specified
* Frame
owner. A shared, hidden frame will be
* set as the owner of the dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog() throws HeadlessException
{
super();
}
/**
* Creates a non-modal dialog without a title with the
* specified Frame
as its owner. If owner
* is null
, a shared, hidden frame will be set as the
* owner of the dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Frame
from which the dialog is displayed
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Frame owner) throws HeadlessException
{
super(owner, false);
}
/**
* Creates a modal or non-modal dialog without a title and
* with the specified owner Frame
. If owner
* is null
, a shared, hidden frame will be set as the
* owner of the dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Frame
from which the dialog is displayed
* @param modal true for a modal dialog, false for one that allows
* others windows to be active at the same time
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Frame owner, boolean modal) throws HeadlessException
{
super(owner, null, modal);
}
/**
* Creates a non-modal dialog with the specified title and
* with the specified owner frame. If owner
* is null
, a shared, hidden frame will be set as the
* owner of the dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Frame
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Frame owner, String title) throws HeadlessException
{
super(owner, title, false);
}
/**
* Creates a modal or non-modal dialog with the specified title
* and the specified owner Frame
. If owner
* is null
, a shared, hidden frame will be set as the
* owner of this dialog. All constructors defer to this one.
*
* NOTE: Any popup components (JComboBox
,
* JPopupMenu
, JMenuBar
)
* created within a modal dialog will be forced to be lightweight.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Frame
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @param modal true for a modal dialog, false for one that allows
* other windows to be active at the same time
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Frame owner, String title, boolean modal)
throws HeadlessException
{
super(owner, title, modal);
}
/**
* Creates a modal or non-modal dialog with the specified title,
* owner Frame
, and GraphicsConfiguration
.
*
*
* NOTE: Any popup components (JComboBox
,
* JPopupMenu
, JMenuBar
)
* created within a modal dialog will be forced to be lightweight.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Frame
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @param modal true for a modal dialog, false for one that allows
* other windows to be active at the same time
* @param gc the GraphicsConfiguration
* of the target screen device. If gc
is
* null
, the same
* GraphicsConfiguration
as the owning Frame is used.
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
* @since 1.4
*/
public JAutoPositionDialog(Frame owner, String title, boolean modal,
GraphicsConfiguration gc)
{
super(owner, title, modal, gc);
}
/**
* Creates a non-modal dialog without a title with the
* specified Dialog
as its owner.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the non-null Dialog
from which the dialog is displayed
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Dialog owner) throws HeadlessException
{
super(owner, false);
}
/**
* Creates a modal or non-modal dialog without a title and
* with the specified owner dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the non-null Dialog
from which the dialog is displayed
* @param modal true for a modal dialog, false for one that allows
* other windows to be active at the same time
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Dialog owner, boolean modal) throws HeadlessException
{
super(owner, null, modal);
}
/**
* Creates a non-modal dialog with the specified title and
* with the specified owner dialog.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the non-null Dialog
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Dialog owner, String title) throws HeadlessException
{
super(owner, title, false);
}
/**
* Creates a modal or non-modal dialog with the specified title
* and the specified owner frame.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the non-null Dialog
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @param modal true for a modal dialog, false for one that allows
* other windows to be active at the same time
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* returns true.
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
*/
public JAutoPositionDialog(Dialog owner, String title, boolean modal)
throws HeadlessException
{
super(owner, title, modal);
}
/**
* Creates a modal or non-modal dialog with the specified title,
* owner Dialog
, and GraphicsConfiguration
.
*
*
* NOTE: Any popup components (JComboBox
,
* JPopupMenu
, JMenuBar
)
* created within a modal dialog will be forced to be lightweight.
*
* This constructor sets the component's locale property to the value
* returned by JComponent.getDefaultLocale
.
*
* @param owner the Dialog
from which the dialog is displayed
* @param title the String
to display in the dialog's
* title bar
* @param modal true for a modal dialog, false for one that allows
* other windows to be active at the same time
* @param gc the GraphicsConfiguration
* of the target screen device. If gc
is
* null
, the same
* GraphicsConfiguration
as the owning Dialog is used.
* @exception HeadlessException if GraphicsEnvironment.isHeadless()
* @see java.awt.GraphicsEnvironment#isHeadless
* @see JComponent#getDefaultLocale
* returns true.
* @since 1.4
*/
public JAutoPositionDialog(Dialog owner, String title, boolean modal,
GraphicsConfiguration gc) throws HeadlessException
{
super(owner, title, modal, gc);
}
/**
* Overrides normal setVisible to auto-adjust the
* dialogs position to the center of the owner frame.
* @param show True, for showing.
*/
public void setVisible(boolean show)
{
if(show)
{
setLocation(SGUI.calculateMiddlePosition(this.getOwner(), this));
super.setVisible(show);
}
else
{
super.setVisible(show);
}
}
}