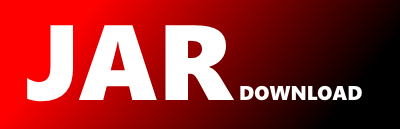
jadex.bytecode.vmhacks.ClassStore Maven / Gradle / Ivy
package jadex.bytecode.vmhacks;
import java.lang.ref.WeakReference;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.WeakHashMap;
/**
* Class used to store injected classes globally.
*
*/
public class ClassStore implements Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy