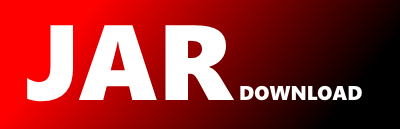
jadex.bytecode.vmhacks.SecurityProviderStore Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jadex-util-bytecode Show documentation
Show all versions of jadex-util-bytecode Show documentation
Jadex bytecode tools contains helper stuff for asm bytecode generation.
package jadex.bytecode.vmhacks;
import java.security.Provider;
import java.security.Security;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.logging.Logger;
/**
* This class abuses the java.security.* API to establish a
* VM-wide object store.
*
*/
class SecurityProviderStore extends Provider
{
/**
* ID
*/
public static final int ID = 23070273;
/** Flag if injected. */
public static boolean INJECTED = false;
/** The delegate list. */
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy