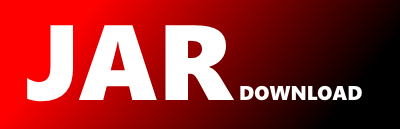
jadex.javaparser.javaccimpl.ParserImpl Maven / Gradle / Ivy
/* ParserImpl.java */
/* Generated By:JJTree&JavaCC: Do not edit this line. ParserImpl.java */
package jadex.javaparser.javaccimpl;
import jadex.commons.SReflect;
import java.util.Map;
import java.util.ArrayList;
/**
* The Jadex parser parses all types of expressions in ADF and queries.
*/
class ParserImpl/*@bgen(jjtree)*/implements ParserImplTreeConstants, ParserImplConstants {/*@bgen(jjtree)*/
protected JJTParserImplState jjtree = new JJTParserImplState();//-------- constants --------
/** The class not found identifier. */
protected static Object NOTFOUND = new Object();
//-------- attributes --------
/** The imports. */
protected String[] imports;
/** The local parameters (name->type). */
protected Map params;
/** The class loader. */
protected ClassLoader classloader;
//-------- attribute accessors --------
/**
* Set the imports (comma separated packages).
* @param imports The imports.
*/
protected void setImports(String[] imports)
{
this.imports = imports;
}
/**
* Get the imports (comma separated packages).
* @return The imports.
*/
protected String[] getImports()
{
return this.imports;
}
/**
* Set the parameters.
*/
protected void setParameters(Map params)
{
this.params = params;
}
/**
* Set the classloader.
* @param classloader The classloader.
*/
protected void setClassLoader(ClassLoader classloader)
{
this.classloader = classloader;
}
/**
* Get the classloader.
* @return The classloader.
*/
protected ClassLoader getClassLoader()
{
return this.classloader;
}
//-------- helper methods --------
/**
* Find a class using imports.
* @param name The class name.
* @return The class, or null if not found.
*/
protected Class findClass0(String name)
{
return SReflect.findClass0(name, imports, classloader);
}
/**
* Find a class using imports.
* @param name The class name.
* @return The class.
* @throws ClassNotFoundException when the class is not found in the imports.
*/
protected Class findClass(String name) throws ClassNotFoundException
{
return SReflect.findClass(name, imports, classloader);
}
/**
* Check if an inner class exists.
* @param outer The outer class.
* @param inner The name of the inner class.
* @return The inner class, or null if not found.
*/
protected Class getInnerClass(Class outer, String inner)
{
return SReflect.classForName0(outer.getName()+"$"+inner, classloader);
}
/**
* Check if a token is an identifier.
* Needed, because the extension keywords
* (like SELECT, ALL etc.) may occur as identifier
* (e.g. java.util.logging.Level.ALL).
*/
protected boolean isIdentifier(Token token)
{
return token.kind==IDENTIFIER
|| token.kind==SELECT
|| token.kind==ALL
|| token.kind==ANY
|| token.kind==ONE
|| token.kind==IOTA
|| token.kind==FROM
|| token.kind==IN
|| token.kind==WHERE
|| token.kind==ORDER
|| token.kind==BY
|| token.kind==ASC
|| token.kind==DESC;
}
/**
* Unescape a string.
*/
protected String unescape(String str)
{
StringBuffer buf = new StringBuffer(str);
int idx = buf.indexOf("\\");
while(idx!=-1 && buf.length()>idx+1)
{
if(buf.charAt(idx+1)=='b')
buf.replace(idx, idx+2, "\b");
else if(buf.charAt(idx+1)=='t')
buf.replace(idx, idx+2, "\t");
else if(buf.charAt(idx+1)=='n')
buf.replace(idx, idx+2, "\n");
else if(buf.charAt(idx+1)=='f')
buf.replace(idx, idx+2, "\f");
else if(buf.charAt(idx+1)=='r')
buf.replace(idx, idx+2, "\r");
else if(buf.charAt(idx+1)=='"')
buf.replace(idx, idx+2, "\"");
else if(buf.charAt(idx+1)=='\'')
buf.replace(idx, idx+2, "'");
else if(buf.charAt(idx+1)=='\\')
buf.replace(idx, idx+2, "\\");
idx = buf.indexOf("\\", idx+1);
}
// Todo: escape octal codes.
return buf.toString();
}
/**
* Peek to some non-top node.
*/
public Node peekNode(JJTParserImplState jjtree, int i)
{
Node ret;
if(i>0)
{
Node[] stack = new Node[i];
for(int s=i-1; s>=0; s--)
stack[s] = jjtree.popNode();
ret = jjtree.peekNode();
for(Node n: stack)
jjtree.pushNode(n);
}
else if(i==0)
{
ret = jjtree.peekNode();
}
else
{
throw new IndexOutOfBoundsException(""+i);
}
return ret;
}
/*
TOKEN : /* OPERATORS * /
{
< GT: ">" >
| < LT: "<" >
| < BANG: "!" >
| < TILDE: "~" >
| < HOOK: "?" >
| < COLON: ":" >
| < EQ: "==" >
| < LE: "<=" >
| < GE: ">=" >
| < NE: "!=" >
| < SC_OR: "||" >
| < SC_AND: "&&" >
| < INCR: "++" >
| < DECR: "--" >
| < PLUS: "+" >
| < MINUS: "-" >
| < STAR: "*" >
| < SLASH: "/" >
| < BIT_AND: "&" >
| < BIT_OR: "|" >
| < XOR: "^" >
| < REM: "%" >
| < LSHIFT: "<<" >
| < RSIGNEDSHIFT: ">>" >
| < RUNSIGNEDSHIFT: ">>>" >
}
*/
//-------- Grammer rules --------
/**
* Parse the supplied expression.
* @return The root node of the generated tree.
*/
final public ExpressionNode parseExpression() throws ParseException {
Expression();
jj_consume_token(0);
// Return the root of the generated tree.
{if ("" != null) return (ExpressionNode)jjtree.rootNode();}
throw new Error("Missing return statement in function");
}
/**
* Parse the supplied type expression.
* @return The class given by the type expression.
*/
final public Class parseType() throws ParseException {
Type();
jj_consume_token(0);
// Return the value of the type expression.
{if ("" != null) return (Class)((TypeNode)jjtree.rootNode()).getValue(null);}
throw new Error("Missing return statement in function");
}
/**
* The start rule.
* All other rules are invoked by this rule,
* and may recursively reinvoke this rule.
*/
final public void Expression() throws ParseException {
if (jj_2_1(2147483647) && (getToken(2).kind != DOT)) {
SelectExpression();
} else if (jj_2_2(1)) {
ConditionalExpression();
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
/**
* The conditional expression (bool ? exp1 : exp2).
* Creates ConditionalNode if present (not yet implemented).
*/
final public void SelectExpression() throws ParseException {// Declare local variables.
Token t1 = null;
String t2 = null;
ArrayList vars = new ArrayList();
Token t3 = null;
Token t4 = null;
Token t5 = null;
SelectNode jjtn002 = new SelectNode(this, JJTSELECTNODE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jj_consume_token(SELECT);
if (jj_2_7(1)) {
if (jj_2_3(2147483647) && (getToken(2).kind != DOT)) {
t1 = jj_consume_token(ALL);
} else if (jj_2_4(2147483647) && (getToken(2).kind != DOT)) {
t1 = jj_consume_token(ANY);
} else if (jj_2_5(2147483647) && (getToken(2).kind != DOT)) {
t1 = jj_consume_token(ONE);
} else if (jj_2_6(2147483647) && (getToken(2).kind != DOT)) {
t1 = jj_consume_token(IOTA);
} else {
jj_consume_token(-1);
throw new ParseException();
}
} else {
;
}
if (jj_2_12(2147483647)) {
ConditionalExpression();
jj_consume_token(FROM);
if (jj_2_8(2147483647)) {
Type();
t2 = Identifier();
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case SELECT:
case ALL:
case ANY:
case IOTA:
case FROM:
case IN:
case WHERE:
case ORDER:
case BY:
case ASC:
case DESC:
case IDENTIFIER:{
t2 = Identifier();
break;
}
default:
jj_la1[0] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
jj_consume_token(IN);
ConditionalExpression();
vars.add(t2);
label_1:
while (true) {
if (jj_2_9(2147483647)) {
;
} else {
break label_1;
}
jj_consume_token(COMMA);
if (jj_2_10(1)) {
Type();
} else {
;
}
t2 = Identifier();
jj_consume_token(IN);
ConditionalExpression();
vars.add(t2);
}
} else if (jj_2_13(1)) {
ParameterNode jjtn001 = new ParameterNode(this, JJTPARAMETERNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
if (jj_2_11(2147483647)) {
Type();
t2 = Identifier();
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case SELECT:
case ALL:
case ANY:
case IOTA:
case FROM:
case IN:
case WHERE:
case ORDER:
case BY:
case ASC:
case DESC:
case IDENTIFIER:{
t2 = Identifier();
break;
}
default:
jj_la1[1] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, true);
}
}
((ExpressionNode)jjtree.peekNode()).setText(t2);
vars.add(t2);
jj_consume_token(FROM);
ConditionalExpression();
} else {
jj_consume_token(-1);
throw new ParseException();
}
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case WHERE:{
t3 = jj_consume_token(WHERE);
ConditionalExpression();
break;
}
default:
jj_la1[2] = jj_gen;
;
}
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case ORDER:{
t4 = jj_consume_token(ORDER);
jj_consume_token(BY);
ConditionalExpression();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case ASC:
case DESC:{
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case ASC:{
t5 = jj_consume_token(ASC);
break;
}
case DESC:{
t5 = jj_consume_token(DESC);
break;
}
default:
jj_la1[3] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
break;
}
default:
jj_la1[4] = jj_gen;
;
}
break;
}
default:
jj_la1[5] = jj_gen;
;
}
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, jjtree.nodeArity() > 1);
}
}
// Set node properties.
if(t1!=null) ((SelectNode)jjtree.peekNode()).setText(t1.kind==ONE ? "any" : t1.image);
((SelectNode)jjtree.peekNode()).setVariables((String[])vars.toArray(new String[vars.size()]));
if(t3!=null) ((SelectNode)jjtree.peekNode()).setWhere(true);
if(t4!=null) ((SelectNode)jjtree.peekNode()).setOrderBy(true);
if(t5!=null) ((SelectNode)jjtree.peekNode()).setOrder(t5.image);
}
/**
* The conditional expression (bool ? exp1 : exp2).
* Creates ConditionalNode if present (not yet implemented).
*/
final public void ConditionalExpression() throws ParseException {
ConditionalNode jjtn001 = new ConditionalNode(this, JJTCONDITIONALNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
ConditionalOrExpression();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case QUESTIONMARK:{
jj_consume_token(QUESTIONMARK);
ConditionalExpression();
jj_consume_token(54);
ConditionalExpression();
break;
}
default:
jj_la1[6] = jj_gen;
;
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, jjtree.nodeArity() > 1);
}
}
}
/**
* Or expression.
* If present, creates BooleanNode with arbitrary number of subnodes.
*/
final public void ConditionalOrExpression() throws ParseException {Token t = null;
BooleanNode jjtn001 = new BooleanNode(this, JJTBOOLEANNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
ConditionalAndExpression();
label_2:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 55:{
;
break;
}
default:
jj_la1[7] = jj_gen;
break label_2;
}
t = jj_consume_token(55);
ConditionalAndExpression();
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, jjtree.nodeArity() > 1);
}
}
// When token is set, node was created.
// Set node text to specify boolean operation.
if(t!=null) ((ExpressionNode)jjtree.peekNode()).setText("||");
}
/**
* And expression.
* If present, creates BooleanNode with arbitrary number of subnodes.
*/
final public void ConditionalAndExpression() throws ParseException {Token t = null;
BooleanNode jjtn001 = new BooleanNode(this, JJTBOOLEANNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
InclusiveOrExpression();
label_3:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 56:{
;
break;
}
default:
jj_la1[8] = jj_gen;
break label_3;
}
t = jj_consume_token(56);
InclusiveOrExpression();
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, jjtree.nodeArity() > 1);
}
}
// When token is set, node was created.
// Set node text to specify boolean operation.
if(t!=null) ((ExpressionNode)jjtree.peekNode()).setText("&&");
}
/**
* Bitwise Or expression (|).
* If present, creates MathNode(s) with two subnodes.
*/
final public void InclusiveOrExpression() throws ParseException {
ExclusiveOrExpression();
label_4:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 57:{
;
break;
}
default:
jj_la1[9] = jj_gen;
break label_4;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(57);
ExclusiveOrExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText("|");
}
}
/**
* Bitwise XOr expression (^).
* If present, creates MathNode(s) with two subnodes.
*/
final public void ExclusiveOrExpression() throws ParseException {
AndExpression();
label_5:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 58:{
;
break;
}
default:
jj_la1[10] = jj_gen;
break label_5;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(58);
AndExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText("^");
}
}
/**
* Bitwise And expression (&).
* If present, creates MathNode(s) with two subnodes.
*/
final public void AndExpression() throws ParseException {
EqualityExpression();
label_6:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 59:{
;
break;
}
default:
jj_la1[11] = jj_gen;
break label_6;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(59);
EqualityExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText("&");
}
}
/**
* Test for (un)equality.
* If present, creates CompareNode(s) with two subnodes.
*/
final public void EqualityExpression() throws ParseException {Token t;
InstanceOfExpression();
label_7:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 60:
case 61:{
;
break;
}
default:
jj_la1[12] = jj_gen;
break label_7;
}
CompareNode jjtn001 = new CompareNode(this, JJTCOMPARENODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 60:{
t = jj_consume_token(60);
break;
}
case 61:{
t = jj_consume_token(61);
break;
}
default:
jj_la1[13] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
InstanceOfExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify compare operation.
((ExpressionNode)jjtree.peekNode()).setText(t.image);
}
}
/**
* Instance of operator.
* If present, creates CompareNode with two subnodes.
*/
final public void InstanceOfExpression() throws ParseException {
RelationalExpression();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case INSTANCEOF:{
CompareNode jjtn001 = new CompareNode(this, JJTCOMPARENODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(INSTANCEOF);
Type();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify compare operation.
((ExpressionNode)jjtree.peekNode()).setText("instanceof");
break;
}
default:
jj_la1[14] = jj_gen;
;
}
}
/**
* Comparison expression
* If present, creates CompareNode(s) with two subnodes.
*/
final public void RelationalExpression() throws ParseException {Token t;
ShiftExpression();
label_8:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:
case 63:
case 64:
case 65:{
;
break;
}
default:
jj_la1[15] = jj_gen;
break label_8;
}
CompareNode jjtn001 = new CompareNode(this, JJTCOMPARENODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:{
t = jj_consume_token(62);
break;
}
case 63:{
t = jj_consume_token(63);
break;
}
case 64:{
t = jj_consume_token(64);
break;
}
case 65:{
t = jj_consume_token(65);
break;
}
default:
jj_la1[16] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
ShiftExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify compare operation.
((ExpressionNode)jjtree.peekNode()).setText(t.image);
}
}
/**
* Shift operation.
* If present, creates MathNode(s) with two subnodes.
*/
final public void ShiftExpression() throws ParseException {String exp;
AdditiveExpression();
label_9:
while (true) {
if (jj_2_14(2)) {
;
} else {
break label_9;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 66:{
jj_consume_token(66);
exp="<<";
break;
}
default:
jj_la1[17] = jj_gen;
if (jj_2_15(3)) {
RUNSIGNEDSHIFT();
exp=">>>";
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 63:{
RSIGNEDSHIFT();
exp=">>";
break;
}
default:
jj_la1[18] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
AdditiveExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText(exp);
}
}
/* We use productions to match >>>, >> and > so that we can keep the
* type declaration syntax with generics clean
*/
final public void RUNSIGNEDSHIFT() throws ParseException {
jj_consume_token(63);
jj_consume_token(63);
jj_consume_token(63);
}
final public void RSIGNEDSHIFT() throws ParseException {
jj_consume_token(63);
jj_consume_token(63);
}
/**
* Additive operation.
* If present, creates MathNode(s) with two subnodes.
*/
final public void AdditiveExpression() throws ParseException {Token t;
MultiplicativeExpression();
label_10:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 67:
case 68:{
;
break;
}
default:
jj_la1[19] = jj_gen;
break label_10;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 67:{
t = jj_consume_token(67);
break;
}
case 68:{
t = jj_consume_token(68);
break;
}
default:
jj_la1[20] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
MultiplicativeExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText(t.image);
}
}
/**
* Multiplicative operation.
* If present, creates MathNode(s) with two subnodes.
*/
final public void MultiplicativeExpression() throws ParseException {Token t;
UnaryExpression();
label_11:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 69:
case 70:
case 71:{
;
break;
}
default:
jj_la1[21] = jj_gen;
break label_11;
}
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 69:{
t = jj_consume_token(69);
break;
}
case 70:{
t = jj_consume_token(70);
break;
}
case 71:{
t = jj_consume_token(71);
break;
}
default:
jj_la1[22] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
UnaryExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
// Set node text to specify math operation.
((ExpressionNode)jjtree.peekNode()).setText(t.image);
}
}
/**
* Unary math operation.
* If present, creates MathNode with single subnode.
*/
final public void UnaryExpression() throws ParseException {Token t;
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 67:
case 68:{
MathNode jjtn001 = new MathNode(this, JJTMATHNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 67:{
t = jj_consume_token(67);
break;
}
case 68:{
t = jj_consume_token(68);
break;
}
default:
jj_la1[23] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
UnaryExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 1);
}
}
// Set node text to specify compare operation.
((ExpressionNode)jjtree.peekNode()).setText(t.image);
break;
}
default:
jj_la1[24] = jj_gen;
if (jj_2_16(1)) {
UnaryExpressionNotPlusMinus();
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
}
/**
* Logical or bitwise Not operator.
* If present, creates BooleanNode or MathNode with single subnode.
* Otherwise goes on with cast or primary expression.
*/
final public void UnaryExpressionNotPlusMinus() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 72:{
BooleanNode jjtn001 = new BooleanNode(this, JJTBOOLEANNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(72);
UnaryExpression();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 1);
}
}
// Set node text to specify boolean operation.
((ExpressionNode)jjtree.peekNode()).setText("!");
break;
}
case 73:{
MathNode jjtn002 = new MathNode(this, JJTMATHNODE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jj_consume_token(73);
UnaryExpression();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 1);
}
}
// Set node text to specify matz operation.
((ExpressionNode)jjtree.peekNode()).setText("~");
break;
}
default:
jj_la1[25] = jj_gen;
if (jj_2_17(2147483647)) {
CastExpression();
} else if (jj_2_18(1)) {
PrimaryExpression();
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
}
/**
* A cast node changes the static type of an unary expression,
* but does not affect the value.
* Creates a cast node having as child the type node and the unary expression.
*/
final public void CastExpression() throws ParseException {/*@bgen(jjtree) CastNode */
CastNode jjtn000 = new CastNode(this, JJTCASTNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LPAREN);
Type();
jj_consume_token(RPAREN);
UnaryExpression();
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* The basic form of an object expression.
* Consists of statement for initial object value (primary prefix)
* e.g., a constructor invocation.
* The prefix may be followed by an arbitrary number
* of subsequent operations (primary suffix)
* e.g., a method invocation or field access.
*/
final public void PrimaryExpression() throws ParseException {
PrimaryPrefix();
label_12:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case LBRACE:
case LBRACKET:
case DOT:{
;
break;
}
default:
jj_la1[26] = jj_gen;
break label_12;
}
PrimarySuffix();
}
}
/**
* The expression for initial values i.e.,
* the leaves of every expression.
* Creates a single node representing the value,
* which may be a literal, parameter, constructor or static method invocation,
* field access, or array creation.
* For parsing braces, expressions like "(" expression ")" are also
* represented as primary prefix.
*/
final public void PrimaryPrefix() throws ParseException {String t=null; Token lit=null;
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case TRUE:
case FALSE:
case NULL:
case INTEGER_LITERAL:
case FLOATING_POINT_LITERAL:
case CHARACTER_LITERAL:
case STRING_LITERAL:{
Literal();
break;
}
case LPAREN:{
jj_consume_token(LPAREN);
Expression();
jj_consume_token(RPAREN);
break;
}
default:
jj_la1[28] = jj_gen;
if (jj_2_19(2147483647)) {
ReflectNode jjtn001 = new ReflectNode(this, JJTREFLECTNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(NEW);
Name();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:{
TypeArguments();
break;
}
default:
jj_la1[27] = jj_gen;
;
}
Arguments();
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
((ReflectNode)jjtree.peekNode()).setType(ReflectNode.CONSTRUCTOR);
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case NEW:{
ArrayExpression();
break;
}
default:
jj_la1[29] = jj_gen;
if (lookaheadStaticMethod()) {
ReflectNode jjtn002 = new ReflectNode(this, JJTREFLECTNODE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
Name();
jj_consume_token(DOT);
t = Identifier();
Arguments();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
((ReflectNode)jjtree.peekNode()).setText(t);
((ReflectNode)jjtree.peekNode()).setType(ReflectNode.STATIC_METHOD);
} else if (jj_2_20(2147483647)) {
ReflectNode jjtn003 = new ReflectNode(this, JJTREFLECTNODE);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
Type();
jj_consume_token(DOT);
t = Identifier();
} catch (Throwable jjte003) {
if (jjtc003) {
jjtree.clearNodeScope(jjtn003);
jjtc003 = false;
} else {
jjtree.popNode();
}
if (jjte003 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte003;}
}
if (jjte003 instanceof ParseException) {
{if (true) throw (ParseException)jjte003;}
}
{if (true) throw (Error)jjte003;}
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 1);
}
}
((ReflectNode)jjtree.peekNode()).setText(t);
((ReflectNode)jjtree.peekNode()).setType(ReflectNode.STATIC_FIELD);
} else if (jj_2_21(4)) {
ParameterNode jjtn004 = new ParameterNode(this, JJTPARAMETERNODE);
boolean jjtc004 = true;
jjtree.openNodeScope(jjtn004);
try {
t = Identifier();
jj_consume_token(LBRACKET);
jj_consume_token(68);
lit = jj_consume_token(INTEGER_LITERAL);
jj_consume_token(RBRACKET);
} catch (Throwable jjte004) {
if (jjtc004) {
jjtree.clearNodeScope(jjtn004);
jjtc004 = false;
} else {
jjtree.popNode();
}
if (jjte004 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte004;}
}
if (jjte004 instanceof ParseException) {
{if (true) throw (ParseException)jjte004;}
}
{if (true) throw (Error)jjte004;}
} finally {
if (jjtc004) {
jjtree.closeNodeScope(jjtn004, true);
}
}
((ExpressionNode)jjtree.peekNode()).setText(t+"[-"+lit+"]");
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case SELECT:
case ALL:
case ANY:
case IOTA:
case FROM:
case IN:
case WHERE:
case ORDER:
case BY:
case ASC:
case DESC:
case IDENTIFIER:{
ParameterNode jjtn005 = new ParameterNode(this, JJTPARAMETERNODE);
boolean jjtc005 = true;
jjtree.openNodeScope(jjtn005);
try {
t = Identifier();
} catch (Throwable jjte005) {
if (jjtc005) {
jjtree.clearNodeScope(jjtn005);
jjtc005 = false;
} else {
jjtree.popNode();
}
if (jjte005 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte005;}
}
if (jjte005 instanceof ParseException) {
{if (true) throw (ParseException)jjte005;}
}
{if (true) throw (Error)jjte005;}
} finally {
if (jjtc005) {
jjtree.closeNodeScope(jjtn005, true);
}
}
((ExpressionNode)jjtree.peekNode()).setText(t);
break;
}
default:
jj_la1[30] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
}
}
}
/**
* Method to lookahead for a class name.
*/
final public boolean classname() throws ParseException {
// Starts with name.
String name = null;
int next = 1;
boolean havename = false;
if(isIdentifier(getToken(next)))
{
name = getToken(next).image;
havename = findClass0(name)!=null;
next++;
}
else
{
{if ("" != null) return false;}
}
// Append "." while available and class not found.
while(!havename && getToken(next).kind==DOT && isIdentifier(getToken(next+1)))
{
name += "." + getToken(next+1).image;
havename = findClass0(name)!=null;
next += 2;
}
{if ("" != null) return havename;}
throw new Error("Missing return statement in function");
}
/**
* Method to lookahead for static methods.
* Expects Name() "." Identifier() "(".
* Syntactic lookahead doesn't work correctly in this case
* (don't ask me why).
*/
final public boolean lookaheadStaticMethod() throws ParseException {
// Starts with name.
String name = null;
int next = 1;
boolean havename = false;
if(isIdentifier(getToken(next)))
{
name = getToken(next).image;
havename = findClass0(name)!=null;
next++;
}
else
{
{if ("" != null) return false;}
}
// Append "." while available and class not found.
while(!havename && getToken(next).kind==DOT && isIdentifier(getToken(next+1)))
{
name += "." + getToken(next+1).image;
havename = findClass0(name)!=null;
next += 2;
}
if(!havename)
{
{if ("" != null) return false;}
}
// Found name, now search for "." "(".
{if ("" != null) return getToken(next).kind==DOT && isIdentifier(getToken(next+1))
&& getToken(next+2).kind==LPAREN;}
throw new Error("Missing return statement in function");
}
/**
* Subsequent operations to perform on an initial value.
* Creates nodes for selection from array, method invocation, field access,
* or filling in of collection values.
*/
final public void PrimarySuffix() throws ParseException {String identifier;
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case LBRACKET:{
SelectionNode jjtn001 = new SelectionNode(this, JJTSELECTIONNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
jj_consume_token(LBRACKET);
Expression();
jj_consume_token(RBRACKET);
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, 2);
}
}
break;
}
default:
jj_la1[31] = jj_gen;
if (jj_2_22(2147483647)) {
ReflectNode jjtn002 = new ReflectNode(this, JJTREFLECTNODE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jj_consume_token(DOT);
identifier = Identifier();
Arguments();
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, 2);
}
}
((ReflectNode)jjtree.peekNode()).setText(identifier);
((ReflectNode)jjtree.peekNode()).setType(ReflectNode.METHOD);
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case DOT:{
ReflectNode jjtn003 = new ReflectNode(this, JJTREFLECTNODE);
boolean jjtc003 = true;
jjtree.openNodeScope(jjtn003);
try {
jj_consume_token(DOT);
identifier = Identifier();
} catch (Throwable jjte003) {
if (jjtc003) {
jjtree.clearNodeScope(jjtn003);
jjtc003 = false;
} else {
jjtree.popNode();
}
if (jjte003 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte003;}
}
if (jjte003 instanceof ParseException) {
{if (true) throw (ParseException)jjte003;}
}
{if (true) throw (Error)jjte003;}
} finally {
if (jjtc003) {
jjtree.closeNodeScope(jjtn003, 1);
}
}
((ReflectNode)jjtree.peekNode()).setText(identifier);
((ReflectNode)jjtree.peekNode()).setType(ReflectNode.FIELD);
break;
}
case LBRACE:{
CollectionNode jjtn004 = new CollectionNode(this, JJTCOLLECTIONNODE);
boolean jjtc004 = true;
jjtree.openNodeScope(jjtn004);
try {
CollectionContent();
} catch (Throwable jjte004) {
if (jjtc004) {
jjtree.clearNodeScope(jjtn004);
jjtc004 = false;
} else {
jjtree.popNode();
}
if (jjte004 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte004;}
}
if (jjte004 instanceof ParseException) {
{if (true) throw (ParseException)jjte004;}
}
{if (true) throw (Error)jjte004;}
} finally {
if (jjtc004) {
jjtree.closeNodeScope(jjtn004, 2);
}
}
break;
}
default:
jj_la1[32] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
}
/**
* A literal value.
* Creates a constant node, with the value filled in.
*/
// Todo: Support long, float, hex, exponents, escapes in characters, strings...
final public void Literal() throws ParseException {/*@bgen(jjtree) ConstantNode */
ConstantNode jjtn000 = new ConstantNode(this, JJTCONSTANTNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);Token t=null;
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case INTEGER_LITERAL:{
t = jj_consume_token(INTEGER_LITERAL);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
String string = t.image;
boolean l = false;
int radix = 10;
if(string.endsWith("l") || string.endsWith("L"))
{
l = true;
string = string.substring(0, string.length()-1);
}
if(string.startsWith("0x"))
{
radix = 16;
string = string.substring(2);
}
else if(string.length()>1 && string.startsWith("0"))
{
radix = 8;
string = string.substring(1);
}
if(l)
jjtn000.setValue(Long.valueOf(string, radix));
else
jjtn000.setValue(Integer.valueOf(string, radix));
break;
}
case FLOATING_POINT_LITERAL:{
t = jj_consume_token(FLOATING_POINT_LITERAL);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
if(t.image.endsWith("f") || t.image.endsWith("F"))
jjtn000.setValue(Float.valueOf(t.image.substring(0, t.image.length()-1)));
else if(t.image.endsWith("d") || t.image.endsWith("D"))
jjtn000.setValue(Double.valueOf(t.image.substring(0, t.image.length()-1)));
else
jjtn000.setValue(Double.valueOf(t.image));
break;
}
case CHARACTER_LITERAL:{
t = jj_consume_token(CHARACTER_LITERAL);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
// Get unescaped character between ''.
jjtn000.setValue(Character.valueOf(unescape(
t.image.substring(1, t.image.length()-1)).charAt(0)));
break;
}
case STRING_LITERAL:{
t = jj_consume_token(STRING_LITERAL);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
// Get string between "".
jjtn000.setValue(unescape(t.image.substring(1, t.image.length()-1)));
break;
}
case TRUE:{
jj_consume_token(TRUE);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setValue(Boolean.TRUE);
break;
}
case FALSE:{
jj_consume_token(FALSE);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setValue(Boolean.FALSE);
break;
}
case NULL:{
jj_consume_token(NULL);
break;
}
default:
jj_la1[33] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Arguments of a method call or constructor invocation.
* Creates a single arguments node, which contains subnodes for
* all argument expressions.
*/
final public void Arguments() throws ParseException {/*@bgen(jjtree) ArgumentsNode */
ArgumentsNode jjtn000 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LPAREN);
if (jj_2_23(1)) {
Expression();
label_13:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case COMMA:{
;
break;
}
default:
jj_la1[34] = jj_gen;
break label_13;
}
jj_consume_token(COMMA);
Expression();
}
} else {
;
}
jj_consume_token(RPAREN);
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* For creating arrays with or without content.
* Creates an array node, with two subnodes
* for array type and arguments.
*/
final public void ArrayExpression() throws ParseException {/*@bgen(jjtree) ArrayNode */
ArrayNode jjtn000 = new ArrayNode(this, JJTARRAYNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
if (jj_2_25(2147483647)) {
jj_consume_token(NEW);
Type();
ArrayContent();
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setType(ArrayNode.ARRAY);
} else if (jj_2_26(2147483647)) {
jj_consume_token(NEW);
if (jj_2_24(1)) {
Name();
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case BOOLEAN:
case DOUBLE:
case FLOAT:
case LONG:
case INT:
case SHORT:
case BYTE:
case CHAR:{
PrimitiveType();
break;
}
default:
jj_la1[35] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:{
TypeArguments();
break;
}
default:
jj_la1[36] = jj_gen;
;
}
ArrayDimensions();
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setType(ArrayNode.ARRAY_DIMENSION);
} else {
jj_consume_token(-1);
throw new ParseException();
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Inline content specification of an array.
* Creates a single arguments node, which contains subnodes for
* all content values.
*/
final public void ArrayContent() throws ParseException {/*@bgen(jjtree) ArgumentsNode */
ArgumentsNode jjtn000 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LBRACE);
if (jj_2_27(1)) {
Expression();
label_14:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case COMMA:{
;
break;
}
default:
jj_la1[37] = jj_gen;
break label_14;
}
jj_consume_token(COMMA);
Expression();
}
} else {
;
}
jj_consume_token(RBRACE);
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Array size specification.
* Creates a single arguments node, which contains subnodes for
* all dimension values.
*/
final public void ArrayDimensions() throws ParseException {/*@bgen(jjtree) ArgumentsNode */
ArgumentsNode jjtn000 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);int i=0;
try {
label_15:
while (true) {
jj_consume_token(LBRACKET);
Expression();
jj_consume_token(RBRACKET);
i++;
// Append array dimension to type node.
// System.out.println("node is: "+peekNode(jjtree, i));
((TypeNode)peekNode(jjtree, i)).appendText("[]");
if (jj_2_28(2)) {
;
} else {
break label_15;
}
}
label_16:
while (true) {
if (jj_2_29(2)) {
;
} else {
break label_16;
}
jj_consume_token(LBRACKET);
jj_consume_token(RBRACKET);
// Append array dimension to type node.
// System.out.println("und hier: "+peekNode(jjtree, i));
((TypeNode)peekNode(jjtree, i)).appendText("[]");
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Inline content specification of a collection.
* Creates a single arguments node, which contains subnodes for
* all content values. Map content values are represented in
* turn by an arguments node with two subnodes for key and value.
*/
final public void CollectionContent() throws ParseException {/*@bgen(jjtree) ArgumentsNode */
ArgumentsNode jjtn000 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
jj_consume_token(LBRACE);
ArgumentsNode jjtn001 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc001 = true;
jjtree.openNodeScope(jjtn001);
try {
if (jj_2_30(1)) {
Expression();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 74:{
jj_consume_token(74);
Expression();
break;
}
default:
jj_la1[38] = jj_gen;
;
}
} else {
;
}
} catch (Throwable jjte001) {
if (jjtc001) {
jjtree.clearNodeScope(jjtn001);
jjtc001 = false;
} else {
jjtree.popNode();
}
if (jjte001 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte001;}
}
if (jjte001 instanceof ParseException) {
{if (true) throw (ParseException)jjte001;}
}
{if (true) throw (Error)jjte001;}
} finally {
if (jjtc001) {
jjtree.closeNodeScope(jjtn001, jjtree.nodeArity() > 1);
}
}
label_17:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case COMMA:{
;
break;
}
default:
jj_la1[39] = jj_gen;
break label_17;
}
ArgumentsNode jjtn002 = new ArgumentsNode(this, JJTARGUMENTSNODE);
boolean jjtc002 = true;
jjtree.openNodeScope(jjtn002);
try {
jj_consume_token(COMMA);
Expression();
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 74:{
jj_consume_token(74);
Expression();
break;
}
default:
jj_la1[40] = jj_gen;
;
}
} catch (Throwable jjte002) {
if (jjtc002) {
jjtree.clearNodeScope(jjtn002);
jjtc002 = false;
} else {
jjtree.popNode();
}
if (jjte002 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte002;}
}
if (jjte002 instanceof ParseException) {
{if (true) throw (ParseException)jjte002;}
}
{if (true) throw (Error)jjte002;}
} finally {
if (jjtc002) {
jjtree.closeNodeScope(jjtn002, jjtree.nodeArity() > 1);
}
}
}
jj_consume_token(RBRACE);
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Parse Java type (class or basic type,
* with or without array brackets).
* Creates TypeNode.
*/
final public void Type() throws ParseException {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case BOOLEAN:
case DOUBLE:
case FLOAT:
case LONG:
case INT:
case SHORT:
case BYTE:
case CHAR:{
PrimitiveType();
break;
}
default:
jj_la1[41] = jj_gen;
if (jj_2_31(1)) {
Name();
} else {
jj_consume_token(-1);
throw new ParseException();
}
}
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:{
TypeArguments();
break;
}
default:
jj_la1[42] = jj_gen;
;
}
label_18:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case LBRACKET:{
;
break;
}
default:
jj_la1[43] = jj_gen;
break label_18;
}
jj_consume_token(LBRACKET);
jj_consume_token(RBRACKET);
((ExpressionNode)jjtree.peekNode()).appendText("[]");
}
}
/**
* Parse primitive type.
* Creates type node.
*/
final public void PrimitiveType() throws ParseException {/*@bgen(jjtree) TypeNode */
TypeNode jjtn000 = new TypeNode(this, JJTTYPENODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);
try {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case BOOLEAN:{
jj_consume_token(BOOLEAN);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("boolean");
jjtn000.setConstantValue(boolean.class);
break;
}
case CHAR:{
jj_consume_token(CHAR);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("char");
jjtn000.setConstantValue(char.class);
break;
}
case BYTE:{
jj_consume_token(BYTE);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("byte");
jjtn000.setConstantValue(byte.class);
break;
}
case SHORT:{
jj_consume_token(SHORT);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("short");
jjtn000.setConstantValue(short.class);
break;
}
case INT:{
jj_consume_token(INT);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("int");
jjtn000.setConstantValue(int.class);
break;
}
case LONG:{
jj_consume_token(LONG);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("long");
jjtn000.setConstantValue(long.class);
break;
}
case FLOAT:{
jj_consume_token(FLOAT);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("float");
jjtn000.setConstantValue(float.class);
break;
}
case DOUBLE:{
jj_consume_token(DOUBLE);
jjtree.closeNodeScope(jjtn000, true);
jjtc000 = false;
jjtn000.setText("double");
jjtn000.setConstantValue(double.class);
break;
}
default:
jj_la1[44] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Parse class name (with package) into given type node.
* Use SReflect.findClass, to assure that class exists.
*/
final public void Name() throws ParseException {/*@bgen(jjtree) TypeNode */
TypeNode jjtn000 = new TypeNode(this, JJTTYPENODE);
boolean jjtc000 = true;
jjtree.openNodeScope(jjtn000);String identifier; Class type;
try {
if (classname()) {
} else {
jj_consume_token(-1);
throw new ParseException();
}
identifier = Identifier();
// Set node text to identifier using token image.
jjtn000.setText(identifier);
// Set type.
type = findClass0(jjtn000.getText());
label_19:
while (true) {
if (jj_2_32(2147483647) && (type==null
|| getInnerClass(type, getToken(2).image)!=null)) {
;
} else {
break label_19;
}
jj_consume_token(DOT);
identifier = Identifier();
if(type==null)
{
// Append identifier to node text using token image.
jjtn000.appendText(".");
jjtn000.appendText(identifier);
// Set type.
type = findClass0(jjtn000.getText());
}
else
{
// Append identifier to node text using token image.
jjtn000.appendText("$");
jjtn000.appendText(identifier);
// Set type.
type = getInnerClass(type, identifier);
}
}
} catch (Throwable jjte000) {
if (jjtc000) {
jjtree.clearNodeScope(jjtn000);
jjtc000 = false;
} else {
jjtree.popNode();
}
if (jjte000 instanceof RuntimeException) {
{if (true) throw (RuntimeException)jjte000;}
}
if (jjte000 instanceof ParseException) {
{if (true) throw (ParseException)jjte000;}
}
{if (true) throw (Error)jjte000;}
} finally {
if (jjtc000) {
jjtree.closeNodeScope(jjtn000, true);
}
}
}
/**
* Parse identifier and return token string.
*/
final public String Identifier() throws ParseException {Token t;
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case IDENTIFIER:{
t = jj_consume_token(IDENTIFIER);
break;
}
case SELECT:{
t = jj_consume_token(SELECT);
break;
}
case ALL:{
t = jj_consume_token(ALL);
break;
}
case ANY:{
t = jj_consume_token(ANY);
break;
}
case IOTA:{
t = jj_consume_token(IOTA);
break;
}
case FROM:{
t = jj_consume_token(FROM);
break;
}
case IN:{
t = jj_consume_token(IN);
break;
}
case WHERE:{
t = jj_consume_token(WHERE);
break;
}
case ORDER:{
t = jj_consume_token(ORDER);
break;
}
case BY:{
t = jj_consume_token(BY);
break;
}
case ASC:{
t = jj_consume_token(ASC);
break;
}
case DESC:{
t = jj_consume_token(DESC);
break;
}
default:
jj_la1[45] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
{if ("" != null) return t.image;}
throw new Error("Missing return statement in function");
}
/**
* Generic type arguments.
* https://github.com/pmd/pmd/blob/master/pmd/etc/grammar/Java.jjt
*/
final public void TypeArguments() throws ParseException {
if (jj_2_33(2)) {
jj_consume_token(62);
TypeArgument();
label_20:
while (true) {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case COMMA:{
;
break;
}
default:
jj_la1[46] = jj_gen;
break label_20;
}
jj_consume_token(COMMA);
TypeArgument();
}
jj_consume_token(63);
} else {
switch ((jj_ntk==-1)?jj_ntk_f():jj_ntk) {
case 62:{
jj_consume_token(62);
jj_consume_token(63);
break;
}
default:
jj_la1[47] = jj_gen;
jj_consume_token(-1);
throw new ParseException();
}
}
}
/**
* A generic type argument.
*/
final public void TypeArgument() throws ParseException {
Type();
jjtree.popNode(); /* hack!!! */
}
private boolean jj_2_1(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_1(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(0, xla); }
}
private boolean jj_2_2(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_2(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(1, xla); }
}
private boolean jj_2_3(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_3(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(2, xla); }
}
private boolean jj_2_4(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_4(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(3, xla); }
}
private boolean jj_2_5(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_5(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(4, xla); }
}
private boolean jj_2_6(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_6(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(5, xla); }
}
private boolean jj_2_7(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_7(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(6, xla); }
}
private boolean jj_2_8(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_8(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(7, xla); }
}
private boolean jj_2_9(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_9(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(8, xla); }
}
private boolean jj_2_10(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_10(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(9, xla); }
}
private boolean jj_2_11(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_11(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(10, xla); }
}
private boolean jj_2_12(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_12(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(11, xla); }
}
private boolean jj_2_13(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_13(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(12, xla); }
}
private boolean jj_2_14(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_14(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(13, xla); }
}
private boolean jj_2_15(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_15(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(14, xla); }
}
private boolean jj_2_16(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_16(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(15, xla); }
}
private boolean jj_2_17(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_17(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(16, xla); }
}
private boolean jj_2_18(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_18(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(17, xla); }
}
private boolean jj_2_19(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_19(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(18, xla); }
}
private boolean jj_2_20(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_20(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(19, xla); }
}
private boolean jj_2_21(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_21(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(20, xla); }
}
private boolean jj_2_22(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_22(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(21, xla); }
}
private boolean jj_2_23(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_23(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(22, xla); }
}
private boolean jj_2_24(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_24(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(23, xla); }
}
private boolean jj_2_25(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_25(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(24, xla); }
}
private boolean jj_2_26(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_26(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(25, xla); }
}
private boolean jj_2_27(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_27(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(26, xla); }
}
private boolean jj_2_28(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_28(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(27, xla); }
}
private boolean jj_2_29(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_29(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(28, xla); }
}
private boolean jj_2_30(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_30(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(29, xla); }
}
private boolean jj_2_31(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_31(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(30, xla); }
}
private boolean jj_2_32(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_32(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(31, xla); }
}
private boolean jj_2_33(int xla)
{
jj_la = xla; jj_lastpos = jj_scanpos = token;
try { return !jj_3_33(); }
catch(LookaheadSuccess ls) { return true; }
finally { jj_save(32, xla); }
}
private boolean jj_3R_49()
{
if (jj_scan_token(63)) return true;
if (jj_scan_token(63)) return true;
return false;
}
private boolean jj_3R_95()
{
if (jj_scan_token(NEW)) return true;
if (jj_3R_22()) return true;
if (jj_3R_119()) return true;
return false;
}
private boolean jj_3R_85()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_95()) {
jj_scanpos = xsp;
if (jj_3R_96()) return true;
}
return false;
}
private boolean jj_3R_31()
{
if (jj_scan_token(63)) return true;
if (jj_scan_token(63)) return true;
if (jj_scan_token(63)) return true;
return false;
}
private boolean jj_3_23()
{
if (jj_3R_36()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_118()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_28()
{
if (jj_scan_token(66)) return true;
return false;
}
private boolean jj_3_14()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_28()) {
jj_scanpos = xsp;
if (jj_3_15()) {
jj_scanpos = xsp;
if (jj_3R_29()) return true;
}
}
if (jj_3R_30()) return true;
return false;
}
private boolean jj_3R_112()
{
if (jj_scan_token(LPAREN)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_23()) jj_scanpos = xsp;
if (jj_scan_token(RPAREN)) return true;
return false;
}
private boolean jj_3R_105()
{
if (jj_3R_30()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3_14()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_106()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(62)) {
jj_scanpos = xsp;
if (jj_scan_token(63)) {
jj_scanpos = xsp;
if (jj_scan_token(64)) {
jj_scanpos = xsp;
if (jj_scan_token(65)) return true;
}
}
}
if (jj_3R_105()) return true;
return false;
}
private boolean jj_3R_94()
{
if (jj_scan_token(FALSE)) return true;
return false;
}
private boolean jj_3R_93()
{
if (jj_scan_token(TRUE)) return true;
return false;
}
private boolean jj_3R_92()
{
if (jj_scan_token(STRING_LITERAL)) return true;
return false;
}
private boolean jj_3R_103()
{
if (jj_3R_105()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_106()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_91()
{
if (jj_scan_token(CHARACTER_LITERAL)) return true;
return false;
}
private boolean jj_3R_104()
{
if (jj_scan_token(INSTANCEOF)) return true;
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3R_90()
{
if (jj_scan_token(FLOATING_POINT_LITERAL)) return true;
return false;
}
private boolean jj_3R_101()
{
if (jj_3R_103()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_104()) jj_scanpos = xsp;
return false;
}
private boolean jj_3R_102()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(60)) {
jj_scanpos = xsp;
if (jj_scan_token(61)) return true;
}
if (jj_3R_101()) return true;
return false;
}
private boolean jj_3R_99()
{
if (jj_3R_101()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_102()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_100()
{
if (jj_scan_token(59)) return true;
if (jj_3R_99()) return true;
return false;
}
private boolean jj_3R_84()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_89()) {
jj_scanpos = xsp;
if (jj_3R_90()) {
jj_scanpos = xsp;
if (jj_3R_91()) {
jj_scanpos = xsp;
if (jj_3R_92()) {
jj_scanpos = xsp;
if (jj_3R_93()) {
jj_scanpos = xsp;
if (jj_3R_94()) {
jj_scanpos = xsp;
if (jj_scan_token(32)) return true;
}
}
}
}
}
}
return false;
}
private boolean jj_3R_89()
{
if (jj_scan_token(INTEGER_LITERAL)) return true;
return false;
}
private boolean jj_3R_97()
{
if (jj_3R_99()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_100()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_116()
{
if (jj_3R_117()) return true;
return false;
}
private boolean jj_3R_98()
{
if (jj_scan_token(58)) return true;
if (jj_3R_97()) return true;
return false;
}
private boolean jj_3_22()
{
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
if (jj_scan_token(LPAREN)) return true;
return false;
}
private boolean jj_3R_115()
{
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3R_87()
{
if (jj_3R_97()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_98()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_114()
{
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
if (jj_3R_112()) return true;
return false;
}
private boolean jj_3R_82()
{
if (jj_scan_token(56)) return true;
if (jj_3R_81()) return true;
return false;
}
private boolean jj_3R_110()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_113()) {
jj_scanpos = xsp;
if (jj_3R_114()) {
jj_scanpos = xsp;
if (jj_3R_115()) {
jj_scanpos = xsp;
if (jj_3R_116()) return true;
}
}
}
return false;
}
private boolean jj_3R_113()
{
if (jj_scan_token(LBRACKET)) return true;
if (jj_3R_36()) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3R_88()
{
if (jj_scan_token(57)) return true;
if (jj_3R_87()) return true;
return false;
}
private boolean jj_3R_27()
{
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3R_130()
{
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3R_81()
{
if (jj_3R_87()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_88()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_86()
{
if (jj_scan_token(COMMA)) return true;
if (jj_3R_43()) return true;
return false;
}
private boolean jj_3R_61()
{
if (jj_scan_token(55)) return true;
if (jj_3R_60()) return true;
return false;
}
private boolean jj_3R_132()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(20)) {
jj_scanpos = xsp;
if (jj_scan_token(21)) return true;
}
return false;
}
private boolean jj_3R_48()
{
if (jj_scan_token(QUESTIONMARK)) return true;
if (jj_3R_21()) return true;
if (jj_scan_token(54)) return true;
if (jj_3R_21()) return true;
return false;
}
private boolean jj_3R_60()
{
if (jj_3R_81()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_82()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_43()
{
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3R_71()
{
if (jj_scan_token(62)) return true;
if (jj_scan_token(63)) return true;
return false;
}
private boolean jj_3R_44()
{
if (jj_3R_60()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_61()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_25()
{
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3R_57()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3_33()) {
jj_scanpos = xsp;
if (jj_3R_71()) return true;
}
return false;
}
private boolean jj_3_33()
{
if (jj_scan_token(62)) return true;
if (jj_3R_43()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_86()) { jj_scanpos = xsp; break; }
}
if (jj_scan_token(63)) return true;
return false;
}
private boolean jj_3R_24()
{
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3_9()
{
if (jj_scan_token(COMMA)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_24()) jj_scanpos = xsp;
if (jj_3R_23()) return true;
if (jj_scan_token(IN)) return true;
return false;
}
private boolean jj_3R_21()
{
if (jj_3R_44()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_48()) jj_scanpos = xsp;
return false;
}
private boolean jj_3_10()
{
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3_8()
{
if (jj_3R_22()) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3_11()
{
if (jj_3R_22()) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3R_131()
{
if (jj_scan_token(COMMA)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_10()) jj_scanpos = xsp;
if (jj_3R_23()) return true;
if (jj_scan_token(IN)) return true;
if (jj_3R_21()) return true;
return false;
}
private boolean jj_3R_126()
{
if (jj_scan_token(ORDER)) return true;
if (jj_scan_token(BY)) return true;
if (jj_3R_21()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_132()) jj_scanpos = xsp;
return false;
}
private boolean jj_3R_125()
{
if (jj_scan_token(WHERE)) return true;
if (jj_3R_21()) return true;
return false;
}
private boolean jj_3R_129()
{
if (jj_3R_22()) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3_6()
{
if (jj_scan_token(IOTA)) return true;
return false;
}
private boolean jj_3R_26()
{
if (jj_3R_22()) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3_5()
{
if (jj_scan_token(ONE)) return true;
return false;
}
private boolean jj_3_4()
{
if (jj_scan_token(ANY)) return true;
return false;
}
private boolean jj_3R_23()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(42)) {
jj_scanpos = xsp;
if (jj_scan_token(10)) {
jj_scanpos = xsp;
if (jj_scan_token(11)) {
jj_scanpos = xsp;
if (jj_scan_token(12)) {
jj_scanpos = xsp;
if (jj_scan_token(14)) {
jj_scanpos = xsp;
if (jj_scan_token(15)) {
jj_scanpos = xsp;
if (jj_scan_token(16)) {
jj_scanpos = xsp;
if (jj_scan_token(17)) {
jj_scanpos = xsp;
if (jj_scan_token(18)) {
jj_scanpos = xsp;
if (jj_scan_token(19)) {
jj_scanpos = xsp;
if (jj_scan_token(20)) {
jj_scanpos = xsp;
if (jj_scan_token(21)) return true;
}
}
}
}
}
}
}
}
}
}
}
return false;
}
private boolean jj_3_3()
{
if (jj_scan_token(ALL)) return true;
return false;
}
private boolean jj_3_13()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_26()) {
jj_scanpos = xsp;
if (jj_3R_27()) return true;
}
if (jj_scan_token(FROM)) return true;
if (jj_3R_21()) return true;
return false;
}
private boolean jj_3_12()
{
if (jj_3R_21()) return true;
if (jj_scan_token(FROM)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_25()) jj_scanpos = xsp;
if (jj_3R_23()) return true;
if (jj_scan_token(IN)) return true;
return false;
}
private boolean jj_3_7()
{
Token xsp;
xsp = jj_scanpos;
jj_lookingAhead = true;
jj_semLA = getToken(2).kind != DOT;
jj_lookingAhead = false;
if (!jj_semLA || jj_scan_token(11)) {
jj_scanpos = xsp;
jj_lookingAhead = true;
jj_semLA = getToken(2).kind != DOT;
jj_lookingAhead = false;
if (!jj_semLA || jj_scan_token(12)) {
jj_scanpos = xsp;
jj_lookingAhead = true;
jj_semLA = getToken(2).kind != DOT;
jj_lookingAhead = false;
if (!jj_semLA || jj_scan_token(13)) {
jj_scanpos = xsp;
jj_lookingAhead = true;
jj_semLA = getToken(2).kind != DOT;
jj_lookingAhead = false;
if (!jj_semLA || jj_scan_token(14)) return true;
}
}
}
return false;
}
private boolean jj_3_32()
{
if (jj_scan_token(DOT)) return true;
return false;
}
private boolean jj_3R_124()
{
if (jj_3R_21()) return true;
if (jj_scan_token(FROM)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_129()) {
jj_scanpos = xsp;
if (jj_3R_130()) return true;
}
if (jj_scan_token(IN)) return true;
if (jj_3R_21()) return true;
while (true) {
xsp = jj_scanpos;
if (jj_3R_131()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_70()
{
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3R_56()
{
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3_20()
{
if (jj_3R_22()) return true;
return false;
}
private boolean jj_3_21()
{
if (jj_3R_23()) return true;
if (jj_scan_token(LBRACKET)) return true;
if (jj_scan_token(68)) return true;
if (jj_scan_token(INTEGER_LITERAL)) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3R_35()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_72()
{
if (jj_scan_token(SELECT)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_7()) jj_scanpos = xsp;
xsp = jj_scanpos;
if (jj_3R_124()) {
jj_scanpos = xsp;
if (jj_3_13()) return true;
}
xsp = jj_scanpos;
if (jj_3R_125()) jj_scanpos = xsp;
xsp = jj_scanpos;
if (jj_3R_126()) jj_scanpos = xsp;
return false;
}
private boolean jj_3R_55()
{
return false;
}
private boolean jj_3R_111()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_69()
{
if (jj_3R_22()) return true;
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
return false;
}
private boolean jj_3_1()
{
if (jj_scan_token(SELECT)) return true;
return false;
}
private boolean jj_3R_34()
{
jj_lookingAhead = true;
jj_semLA = classname();
jj_lookingAhead = false;
if (!jj_semLA || jj_3R_55()) return true;
if (jj_3R_23()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_56()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_19()
{
if (jj_scan_token(NEW)) return true;
if (jj_3R_34()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_35()) jj_scanpos = xsp;
if (jj_scan_token(LPAREN)) return true;
return false;
}
private boolean jj_3R_68()
{
if (jj_3R_34()) return true;
if (jj_scan_token(DOT)) return true;
if (jj_3R_23()) return true;
if (jj_3R_112()) return true;
return false;
}
private boolean jj_3R_67()
{
if (jj_3R_85()) return true;
return false;
}
private boolean jj_3_2()
{
if (jj_3R_21()) return true;
return false;
}
private boolean jj_3R_58()
{
if (jj_3R_72()) return true;
return false;
}
private boolean jj_3R_36()
{
Token xsp;
xsp = jj_scanpos;
jj_lookingAhead = true;
jj_semLA = getToken(2).kind != DOT;
jj_lookingAhead = false;
if (!jj_semLA || jj_3R_58()) {
jj_scanpos = xsp;
if (jj_3_2()) return true;
}
return false;
}
private boolean jj_3R_66()
{
if (jj_scan_token(NEW)) return true;
if (jj_3R_34()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_111()) jj_scanpos = xsp;
if (jj_3R_112()) return true;
return false;
}
private boolean jj_3R_80()
{
if (jj_scan_token(DOUBLE)) return true;
return false;
}
private boolean jj_3R_79()
{
if (jj_scan_token(FLOAT)) return true;
return false;
}
private boolean jj_3R_78()
{
if (jj_scan_token(LONG)) return true;
return false;
}
private boolean jj_3R_109()
{
if (jj_3R_110()) return true;
return false;
}
private boolean jj_3R_77()
{
if (jj_scan_token(INT)) return true;
return false;
}
private boolean jj_3R_65()
{
if (jj_scan_token(LPAREN)) return true;
if (jj_3R_36()) return true;
if (jj_scan_token(RPAREN)) return true;
return false;
}
private boolean jj_3R_54()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_64()) {
jj_scanpos = xsp;
if (jj_3R_65()) {
jj_scanpos = xsp;
if (jj_3R_66()) {
jj_scanpos = xsp;
if (jj_3R_67()) {
jj_scanpos = xsp;
jj_lookingAhead = true;
jj_semLA = lookaheadStaticMethod();
jj_lookingAhead = false;
if (!jj_semLA || jj_3R_68()) {
jj_scanpos = xsp;
if (jj_3R_69()) {
jj_scanpos = xsp;
if (jj_3_21()) {
jj_scanpos = xsp;
if (jj_3R_70()) return true;
}
}
}
}
}
}
}
return false;
}
private boolean jj_3R_64()
{
if (jj_3R_84()) return true;
return false;
}
private boolean jj_3R_76()
{
if (jj_scan_token(SHORT)) return true;
return false;
}
private boolean jj_3R_46()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_75()
{
if (jj_scan_token(BYTE)) return true;
return false;
}
private boolean jj_3R_128()
{
if (jj_scan_token(74)) return true;
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3R_74()
{
if (jj_scan_token(CHAR)) return true;
return false;
}
private boolean jj_3R_33()
{
if (jj_3R_54()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_109()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_31()
{
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3R_73()
{
if (jj_scan_token(BOOLEAN)) return true;
return false;
}
private boolean jj_3R_59()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_73()) {
jj_scanpos = xsp;
if (jj_3R_74()) {
jj_scanpos = xsp;
if (jj_3R_75()) {
jj_scanpos = xsp;
if (jj_3R_76()) {
jj_scanpos = xsp;
if (jj_3R_77()) {
jj_scanpos = xsp;
if (jj_3R_78()) {
jj_scanpos = xsp;
if (jj_3R_79()) {
jj_scanpos = xsp;
if (jj_3R_80()) return true;
}
}
}
}
}
}
}
return false;
}
private boolean jj_3R_47()
{
if (jj_scan_token(LBRACKET)) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3R_127()
{
if (jj_scan_token(74)) return true;
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3R_63()
{
if (jj_scan_token(LPAREN)) return true;
if (jj_3R_22()) return true;
if (jj_scan_token(RPAREN)) return true;
if (jj_3R_62()) return true;
return false;
}
private boolean jj_3_17()
{
if (jj_scan_token(LPAREN)) return true;
if (jj_3R_22()) return true;
if (jj_scan_token(RPAREN)) return true;
return false;
}
private boolean jj_3R_123()
{
if (jj_scan_token(COMMA)) return true;
if (jj_3R_36()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_128()) jj_scanpos = xsp;
return false;
}
private boolean jj_3R_45()
{
if (jj_3R_59()) return true;
return false;
}
private boolean jj_3R_22()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_45()) {
jj_scanpos = xsp;
if (jj_3_31()) return true;
}
xsp = jj_scanpos;
if (jj_3R_46()) jj_scanpos = xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_47()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_18()
{
if (jj_3R_33()) return true;
return false;
}
private boolean jj_3R_53()
{
if (jj_3R_63()) return true;
return false;
}
private boolean jj_3_30()
{
if (jj_3R_36()) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_127()) jj_scanpos = xsp;
return false;
}
private boolean jj_3R_52()
{
if (jj_scan_token(73)) return true;
if (jj_3R_62()) return true;
return false;
}
private boolean jj_3R_117()
{
if (jj_scan_token(LBRACE)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_30()) jj_scanpos = xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_123()) { jj_scanpos = xsp; break; }
}
if (jj_scan_token(RBRACE)) return true;
return false;
}
private boolean jj_3R_51()
{
if (jj_scan_token(72)) return true;
if (jj_3R_62()) return true;
return false;
}
private boolean jj_3R_32()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_51()) {
jj_scanpos = xsp;
if (jj_3R_52()) {
jj_scanpos = xsp;
if (jj_3R_53()) {
jj_scanpos = xsp;
if (jj_3_18()) return true;
}
}
}
return false;
}
private boolean jj_3R_29()
{
if (jj_3R_49()) return true;
return false;
}
private boolean jj_3_29()
{
if (jj_scan_token(LBRACKET)) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3_16()
{
if (jj_3R_32()) return true;
return false;
}
private boolean jj_3R_42()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_62()
{
Token xsp;
xsp = jj_scanpos;
if (jj_3R_83()) {
jj_scanpos = xsp;
if (jj_3_16()) return true;
}
return false;
}
private boolean jj_3R_83()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(67)) {
jj_scanpos = xsp;
if (jj_scan_token(68)) return true;
}
if (jj_3R_62()) return true;
return false;
}
private boolean jj_3R_121()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_39()
{
if (jj_3R_57()) return true;
return false;
}
private boolean jj_3R_133()
{
if (jj_scan_token(COMMA)) return true;
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3_28()
{
if (jj_scan_token(LBRACKET)) return true;
if (jj_3R_36()) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3R_108()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(69)) {
jj_scanpos = xsp;
if (jj_scan_token(70)) {
jj_scanpos = xsp;
if (jj_scan_token(71)) return true;
}
}
if (jj_3R_62()) return true;
return false;
}
private boolean jj_3R_41()
{
if (jj_3R_59()) return true;
return false;
}
private boolean jj_3R_122()
{
Token xsp;
if (jj_3_28()) return true;
while (true) {
xsp = jj_scanpos;
if (jj_3_28()) { jj_scanpos = xsp; break; }
}
while (true) {
xsp = jj_scanpos;
if (jj_3_29()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_50()
{
if (jj_3R_62()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_108()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_38()
{
if (jj_3R_59()) return true;
return false;
}
private boolean jj_3R_120()
{
if (jj_3R_59()) return true;
return false;
}
private boolean jj_3_27()
{
if (jj_3R_36()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_133()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3R_40()
{
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3R_107()
{
Token xsp;
xsp = jj_scanpos;
if (jj_scan_token(67)) {
jj_scanpos = xsp;
if (jj_scan_token(68)) return true;
}
if (jj_3R_50()) return true;
return false;
}
private boolean jj_3R_119()
{
if (jj_scan_token(LBRACE)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_27()) jj_scanpos = xsp;
if (jj_scan_token(RBRACE)) return true;
return false;
}
private boolean jj_3R_37()
{
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3_24()
{
if (jj_3R_34()) return true;
return false;
}
private boolean jj_3_26()
{
if (jj_scan_token(NEW)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_40()) {
jj_scanpos = xsp;
if (jj_3R_41()) return true;
}
xsp = jj_scanpos;
if (jj_3R_42()) jj_scanpos = xsp;
if (jj_scan_token(LBRACKET)) return true;
return false;
}
private boolean jj_3_15()
{
if (jj_3R_31()) return true;
return false;
}
private boolean jj_3R_118()
{
if (jj_scan_token(COMMA)) return true;
if (jj_3R_36()) return true;
return false;
}
private boolean jj_3R_30()
{
if (jj_3R_50()) return true;
Token xsp;
while (true) {
xsp = jj_scanpos;
if (jj_3R_107()) { jj_scanpos = xsp; break; }
}
return false;
}
private boolean jj_3_25()
{
if (jj_scan_token(NEW)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3R_37()) {
jj_scanpos = xsp;
if (jj_3R_38()) return true;
}
xsp = jj_scanpos;
if (jj_3R_39()) jj_scanpos = xsp;
if (jj_scan_token(LBRACKET)) return true;
if (jj_scan_token(RBRACKET)) return true;
return false;
}
private boolean jj_3R_96()
{
if (jj_scan_token(NEW)) return true;
Token xsp;
xsp = jj_scanpos;
if (jj_3_24()) {
jj_scanpos = xsp;
if (jj_3R_120()) return true;
}
xsp = jj_scanpos;
if (jj_3R_121()) jj_scanpos = xsp;
if (jj_3R_122()) return true;
return false;
}
/** Generated Token Manager. */
public ParserImplTokenManager token_source;
JavaCharStream jj_input_stream;
/** Current token. */
public Token token;
/** Next token. */
public Token jj_nt;
private int jj_ntk;
private Token jj_scanpos, jj_lastpos;
private int jj_la;
/** Whether we are looking ahead. */
private boolean jj_lookingAhead = false;
private boolean jj_semLA;
private int jj_gen;
final private int[] jj_la1 = new int[48];
static private int[] jj_la1_0;
static private int[] jj_la1_1;
static private int[] jj_la1_2;
static {
jj_la1_init_0();
jj_la1_init_1();
jj_la1_init_2();
}
private static void jj_la1_init_0() {
jj_la1_0 = new int[] {0x3fdc00,0x3fdc00,0x20000,0x300000,0x300000,0x40000,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x200,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0xc0000000,0x100,0x3fdc00,0x0,0x0,0xc0000000,0x0,0x3fc00000,0x0,0x0,0x0,0x0,0x0,0x3fc00000,0x0,0x0,0x3fc00000,0x3fdc00,0x0,0x0,};
}
private static void jj_la1_init_1() {
jj_la1_1 = new int[] {0x400,0x400,0x0,0x0,0x0,0x0,0x200,0x800000,0x1000000,0x2000000,0x4000000,0x8000000,0x30000000,0x30000000,0x0,0xc0000000,0xc0000000,0x0,0x80000000,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x228000,0x40000000,0x21a3,0x0,0x400,0x20000,0x208000,0x1a3,0x100000,0x0,0x40000000,0x100000,0x0,0x100000,0x0,0x0,0x40000000,0x20000,0x0,0x400,0x100000,0x40000000,};
}
private static void jj_la1_init_2() {
jj_la1_2 = new int[] {0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x3,0x3,0x4,0x0,0x18,0x18,0xe0,0xe0,0x18,0x18,0x300,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x400,0x0,0x400,0x0,0x0,0x0,0x0,0x0,0x0,0x0,};
}
final private JJCalls[] jj_2_rtns = new JJCalls[33];
private boolean jj_rescan = false;
private int jj_gc = 0;
/** Constructor with InputStream. */
public ParserImpl(java.io.InputStream stream) {
this(stream, null);
}
/** Constructor with InputStream and supplied encoding */
public ParserImpl(java.io.InputStream stream, String encoding) {
try { jj_input_stream = new JavaCharStream(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source = new ParserImplTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream) {
ReInit(stream, null);
}
/** Reinitialise. */
public void ReInit(java.io.InputStream stream, String encoding) {
try { jj_input_stream.ReInit(stream, encoding, 1, 1); } catch(java.io.UnsupportedEncodingException e) { throw new RuntimeException(e); }
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Constructor. */
public ParserImpl(java.io.Reader stream) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
token_source = new ParserImplTokenManager(jj_input_stream);
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(java.io.Reader stream) {
if (jj_input_stream == null) {
jj_input_stream = new JavaCharStream(stream, 1, 1);
} else {
jj_input_stream.ReInit(stream, 1, 1);
}
if (token_source == null) {
token_source = new ParserImplTokenManager(jj_input_stream);
}
token_source.ReInit(jj_input_stream);
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Constructor with generated Token Manager. */
public ParserImpl(ParserImplTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
/** Reinitialise. */
public void ReInit(ParserImplTokenManager tm) {
token_source = tm;
token = new Token();
jj_ntk = -1;
jjtree.reset();
jj_gen = 0;
for (int i = 0; i < 48; i++) jj_la1[i] = -1;
for (int i = 0; i < jj_2_rtns.length; i++) jj_2_rtns[i] = new JJCalls();
}
private Token jj_consume_token(int kind) throws ParseException {
Token oldToken;
if ((oldToken = token).next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
if (token.kind == kind) {
jj_gen++;
if (++jj_gc > 100) {
jj_gc = 0;
for (int i = 0; i < jj_2_rtns.length; i++) {
JJCalls c = jj_2_rtns[i];
while (c != null) {
if (c.gen < jj_gen) c.first = null;
c = c.next;
}
}
}
return token;
}
token = oldToken;
jj_kind = kind;
throw generateParseException();
}
@SuppressWarnings("serial")
static private final class LookaheadSuccess extends java.lang.Error { }
final private LookaheadSuccess jj_ls = new LookaheadSuccess();
private boolean jj_scan_token(int kind) {
if (jj_scanpos == jj_lastpos) {
jj_la--;
if (jj_scanpos.next == null) {
jj_lastpos = jj_scanpos = jj_scanpos.next = token_source.getNextToken();
} else {
jj_lastpos = jj_scanpos = jj_scanpos.next;
}
} else {
jj_scanpos = jj_scanpos.next;
}
if (jj_rescan) {
int i = 0; Token tok = token;
while (tok != null && tok != jj_scanpos) { i++; tok = tok.next; }
if (tok != null) jj_add_error_token(kind, i);
}
if (jj_scanpos.kind != kind) return true;
if (jj_la == 0 && jj_scanpos == jj_lastpos) throw jj_ls;
return false;
}
/** Get the next Token. */
final public Token getNextToken() {
if (token.next != null) token = token.next;
else token = token.next = token_source.getNextToken();
jj_ntk = -1;
jj_gen++;
return token;
}
/** Get the specific Token. */
final public Token getToken(int index) {
Token t = jj_lookingAhead ? jj_scanpos : token;
for (int i = 0; i < index; i++) {
if (t.next != null) t = t.next;
else t = t.next = token_source.getNextToken();
}
return t;
}
private int jj_ntk_f() {
if ((jj_nt=token.next) == null)
return (jj_ntk = (token.next=token_source.getNextToken()).kind);
else
return (jj_ntk = jj_nt.kind);
}
private java.util.List jj_expentries = new java.util.ArrayList();
private int[] jj_expentry;
private int jj_kind = -1;
private int[] jj_lasttokens = new int[100];
private int jj_endpos;
private void jj_add_error_token(int kind, int pos) {
if (pos >= 100) {
return;
}
if (pos == jj_endpos + 1) {
jj_lasttokens[jj_endpos++] = kind;
} else if (jj_endpos != 0) {
jj_expentry = new int[jj_endpos];
for (int i = 0; i < jj_endpos; i++) {
jj_expentry[i] = jj_lasttokens[i];
}
for (int[] oldentry : jj_expentries) {
if (oldentry.length == jj_expentry.length) {
boolean isMatched = true;
for (int i = 0; i < jj_expentry.length; i++) {
if (oldentry[i] != jj_expentry[i]) {
isMatched = false;
break;
}
}
if (isMatched) {
jj_expentries.add(jj_expentry);
break;
}
}
}
if (pos != 0) {
jj_lasttokens[(jj_endpos = pos) - 1] = kind;
}
}
}
/** Generate ParseException. */
public ParseException generateParseException() {
jj_expentries.clear();
boolean[] la1tokens = new boolean[75];
if (jj_kind >= 0) {
la1tokens[jj_kind] = true;
jj_kind = -1;
}
for (int i = 0; i < 48; i++) {
if (jj_la1[i] == jj_gen) {
for (int j = 0; j < 32; j++) {
if ((jj_la1_0[i] & (1< jj_gen) {
jj_la = p.arg; jj_lastpos = jj_scanpos = p.first;
switch (i) {
case 0: jj_3_1(); break;
case 1: jj_3_2(); break;
case 2: jj_3_3(); break;
case 3: jj_3_4(); break;
case 4: jj_3_5(); break;
case 5: jj_3_6(); break;
case 6: jj_3_7(); break;
case 7: jj_3_8(); break;
case 8: jj_3_9(); break;
case 9: jj_3_10(); break;
case 10: jj_3_11(); break;
case 11: jj_3_12(); break;
case 12: jj_3_13(); break;
case 13: jj_3_14(); break;
case 14: jj_3_15(); break;
case 15: jj_3_16(); break;
case 16: jj_3_17(); break;
case 17: jj_3_18(); break;
case 18: jj_3_19(); break;
case 19: jj_3_20(); break;
case 20: jj_3_21(); break;
case 21: jj_3_22(); break;
case 22: jj_3_23(); break;
case 23: jj_3_24(); break;
case 24: jj_3_25(); break;
case 25: jj_3_26(); break;
case 26: jj_3_27(); break;
case 27: jj_3_28(); break;
case 28: jj_3_29(); break;
case 29: jj_3_30(); break;
case 30: jj_3_31(); break;
case 31: jj_3_32(); break;
case 32: jj_3_33(); break;
}
}
p = p.next;
} while (p != null);
} catch(LookaheadSuccess ls) { }
}
jj_rescan = false;
}
private void jj_save(int index, int xla) {
JJCalls p = jj_2_rtns[index];
while (p.gen > jj_gen) {
if (p.next == null) { p = p.next = new JJCalls(); break; }
p = p.next;
}
p.gen = jj_gen + xla - jj_la;
p.first = token;
p.arg = xla;
}
static final class JJCalls {
int gen;
Token first;
int arg;
JJCalls next;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy