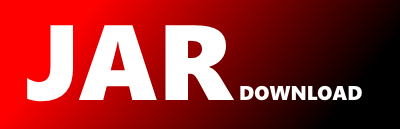
org.adeptnet.jmx.addons.kannel.xml.Gateway Maven / Gradle / Ivy
The newest version!
package org.adeptnet.jmx.addons.kannel.xml;
import java.io.Serializable;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
/**
* Java class for gateway complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="gateway">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <all>
* <element name="version" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="status" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="wdp" type="{urn:ietf:params:xml:ns:kannel-1.0}stats" minOccurs="0"/>
* <element name="sms" type="{urn:ietf:params:xml:ns:kannel-1.0}stats" minOccurs="0"/>
* <element name="dlr" type="{urn:ietf:params:xml:ns:kannel-1.0}dlr" minOccurs="0"/>
* <element name="boxes" type="{urn:ietf:params:xml:ns:kannel-1.0}boxes" minOccurs="0"/>
* <element name="smscs" type="{urn:ietf:params:xml:ns:kannel-1.0}smscs" minOccurs="0"/>
* </all>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "gateway", namespace = "urn:ietf:params:xml:ns:kannel-1.0", propOrder = {
})
@XmlRootElement(name = "gateway", namespace = "urn:ietf:params:xml:ns:kannel-1.0")
public class Gateway
implements Serializable, ToString
{
private final static long serialVersionUID = 201401010001L;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected String version;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected String status;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected Stats wdp;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected Stats sms;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected Dlr dlr;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected Boxes boxes;
@XmlElement(namespace = "urn:ietf:params:xml:ns:kannel-1.0")
protected Smscs smscs;
/**
* Gets the value of the version property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getVersion() {
return version;
}
/**
* Sets the value of the version property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setVersion(String value) {
this.version = value;
}
public boolean isSetVersion() {
return (this.version!= null);
}
/**
* Gets the value of the status property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStatus() {
return status;
}
/**
* Sets the value of the status property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStatus(String value) {
this.status = value;
}
public boolean isSetStatus() {
return (this.status!= null);
}
/**
* Gets the value of the wdp property.
*
* @return
* possible object is
* {@link Stats }
*
*/
public Stats getWdp() {
return wdp;
}
/**
* Sets the value of the wdp property.
*
* @param value
* allowed object is
* {@link Stats }
*
*/
public void setWdp(Stats value) {
this.wdp = value;
}
public boolean isSetWdp() {
return (this.wdp!= null);
}
/**
* Gets the value of the sms property.
*
* @return
* possible object is
* {@link Stats }
*
*/
public Stats getSms() {
return sms;
}
/**
* Sets the value of the sms property.
*
* @param value
* allowed object is
* {@link Stats }
*
*/
public void setSms(Stats value) {
this.sms = value;
}
public boolean isSetSms() {
return (this.sms!= null);
}
/**
* Gets the value of the dlr property.
*
* @return
* possible object is
* {@link Dlr }
*
*/
public Dlr getDlr() {
return dlr;
}
/**
* Sets the value of the dlr property.
*
* @param value
* allowed object is
* {@link Dlr }
*
*/
public void setDlr(Dlr value) {
this.dlr = value;
}
public boolean isSetDlr() {
return (this.dlr!= null);
}
/**
* Gets the value of the boxes property.
*
* @return
* possible object is
* {@link Boxes }
*
*/
public Boxes getBoxes() {
return boxes;
}
/**
* Sets the value of the boxes property.
*
* @param value
* allowed object is
* {@link Boxes }
*
*/
public void setBoxes(Boxes value) {
this.boxes = value;
}
public boolean isSetBoxes() {
return (this.boxes!= null);
}
/**
* Gets the value of the smscs property.
*
* @return
* possible object is
* {@link Smscs }
*
*/
public Smscs getSmscs() {
return smscs;
}
/**
* Sets the value of the smscs property.
*
* @param value
* allowed object is
* {@link Smscs }
*
*/
public void setSmscs(Smscs value) {
this.smscs = value;
}
public boolean isSetSmscs() {
return (this.smscs!= null);
}
public String toString() {
final ToStringStrategy strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy strategy) {
{
String theVersion;
theVersion = this.getVersion();
strategy.appendField(locator, this, "version", buffer, theVersion);
}
{
String theStatus;
theStatus = this.getStatus();
strategy.appendField(locator, this, "status", buffer, theStatus);
}
{
Stats theWdp;
theWdp = this.getWdp();
strategy.appendField(locator, this, "wdp", buffer, theWdp);
}
{
Stats theSms;
theSms = this.getSms();
strategy.appendField(locator, this, "sms", buffer, theSms);
}
{
Dlr theDlr;
theDlr = this.getDlr();
strategy.appendField(locator, this, "dlr", buffer, theDlr);
}
{
Boxes theBoxes;
theBoxes = this.getBoxes();
strategy.appendField(locator, this, "boxes", buffer, theBoxes);
}
{
Smscs theSmscs;
theSmscs = this.getSmscs();
strategy.appendField(locator, this, "smscs", buffer, theSmscs);
}
return buffer;
}
public Gateway withVersion(String value) {
setVersion(value);
return this;
}
public Gateway withStatus(String value) {
setStatus(value);
return this;
}
public Gateway withWdp(Stats value) {
setWdp(value);
return this;
}
public Gateway withSms(Stats value) {
setSms(value);
return this;
}
public Gateway withDlr(Dlr value) {
setDlr(value);
return this;
}
public Gateway withBoxes(Boxes value) {
setBoxes(value);
return this;
}
public Gateway withSmscs(Smscs value) {
setSmscs(value);
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy