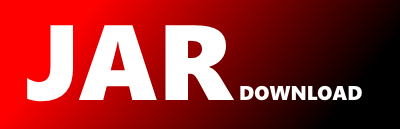
org.adeptnet.jmx.addons.mikrotik.Bean Maven / Gradle / Ivy
/*
* Copyright 2015 Francois Steyn - Adept Internet (PTY) LTD ([email protected]).
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.adeptnet.jmx.addons.mikrotik;
import java.io.IOException;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import javax.net.SocketFactory;
import javax.net.ssl.SSLSocketFactory;
import me.legrange.mikrotik.ApiConnection;
import me.legrange.mikrotik.MikrotikApiException;
/**
*
* @author Francois Steyn - Adept Internet (PTY) LTD ([email protected])
*/
public class Bean implements BeanInterface {
private static final Logger LOG = Logger.getLogger(Bean.class.getName());
private static final BeanData datas = new BeanData();
private final MapThread mapThread;
private final Thread thread;
public static class MapThread implements Runnable {
private boolean running;
public boolean isRunning() {
return running;
}
public void setRunning(final boolean running) {
this.running = running;
}
@Override
public void run() {
long current;
long lastUsed;
BeanData.Record record;
try {
while (isRunning()) {
synchronized (datas) {
current = System.currentTimeMillis();
LOG.log(Level.FINE, "mapThread Running: {0}", datas.getMap().size());
for (Iterator keys = datas.getMap().keySet().iterator(); keys.hasNext();) {
final String key = keys.next();
record = datas.getMap().get(key);
lastUsed = current - record.getLastUsed();
//LOG.info(String.format("%s: %d", key, lastUsed));
if (lastUsed > 30 * 60 * 1000) {
LOG.log(Level.INFO, "Removing old Reference: {0}", key);
keys.remove();
}
}
}
Thread.sleep(60 * 1000);
}
} catch (InterruptedException ex) {
if (isRunning()) {
LOG.log(Level.SEVERE, "mapThread", ex);
}
}
}
}
public Bean() {
mapThread = new MapThread();
thread = new Thread(mapThread);
thread.setDaemon(true);
mapThread.setRunning(true);
}
public void start() {
thread.start();
}
public void stop() {
mapThread.setRunning(false);
thread.interrupt();
}
private BeanData.Record getRecord(final String reference) {
synchronized (datas) {
if (!datas.hasRecord(reference)) {
throw new java.lang.NullPointerException("record is NULL, call loadFromAPI()");
}
return datas.getRecord(reference);
}
}
private void setRecord(final String reference, final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy