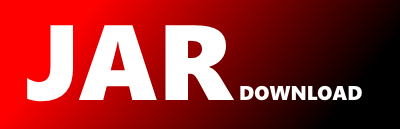
org.miniclient.mock.MockRestApiUserClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of miniclient-core-mock Show documentation
Show all versions of miniclient-core-mock Show documentation
Universal REST API client library in Java. Core-Mock Module
package org.miniclient.mock;
import java.io.IOException;
import java.io.Serializable;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.miniclient.RestApiException;
import org.miniclient.RestApiServiceClient;
import org.miniclient.RestApiUserClient;
import org.miniclient.impl.AbstractRestApiUserClient;
import org.miniclient.proxy.DecoratedRestApiUserClient;
// "Mock" object.
// We have a very strange "dual" implementation.
// We use either inheritance or decoration, based on how the object is constructed.
public class MockRestApiUserClient extends AbstractRestApiUserClient implements DecoratedRestApiUserClient, Serializable
{
private static final Logger log = Logger.getLogger(MockRestApiUserClient.class.getName());
private static final long serialVersionUID = 1L;
private final RestApiUserClient decoratedClient;
// Based on the use of a particular ctor,
// we use either inheritance or decoration.
public MockRestApiUserClient(RestApiServiceClient restApiServiceClient)
{
this(null, restApiServiceClient);
}
public MockRestApiUserClient(RestApiUserClient decoratedClient)
{
this(decoratedClient, null);
}
private MockRestApiUserClient(RestApiUserClient decoratedClient, RestApiServiceClient restApiServiceClient)
{
super(restApiServiceClient);
this.decoratedClient = decoratedClient;
}
// Override methods.
// Note the unusual "dual" delegation.
@Override
public Map get(String id,
Map params) throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.get(): id = " + id + "; params = " + params);
if(decoratedClient != null) {
return decoratedClient.get(id, params);
} else {
return super.get(id, params);
}
}
@Override
public Map post(Object inputData)
throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.post(): inputData = " + inputData);
if(decoratedClient != null) {
return decoratedClient.post(inputData);
} else {
return super.post(inputData);
}
}
@Override
public Map put(Object inputData,
String id) throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.put(): inputData = " + inputData + "; id = " + id);
if(decoratedClient != null) {
return decoratedClient.put(inputData, id);
} else {
return super.put(inputData, id);
}
}
@Override
public Map patch(Object partialData, String id) throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.patch(): partialData = " + partialData + "; id = " + id);
if(decoratedClient != null) {
return decoratedClient.patch(partialData, id);
} else {
return super.patch(partialData, id);
}
}
@Override
public Map delete(String id,
Map params) throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.delete(): id = " + id + "; params = " + params);
if(decoratedClient != null) {
return decoratedClient.delete(id, params);
} else {
return super.delete(id, params);
}
}
@Override
public Object get(String id) throws RestApiException, IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestApiUserClient.get(): id = " + id);
if(decoratedClient != null) {
return decoratedClient.get(id);
} else {
return super.get(id);
}
}
@Override
public List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy