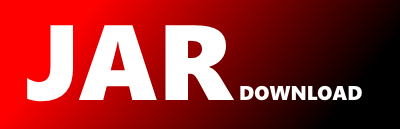
org.miniclient.mock.MockRestServiceClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of miniclient-core-mock Show documentation
Show all versions of miniclient-core-mock Show documentation
Universal REST API client library in Java. Core-Mock Module
package org.miniclient.mock;
import java.io.IOException;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.miniclient.RestServiceClient;
import org.miniclient.common.ResourceUrlBuilder;
import org.miniclient.core.ContentFormat;
import org.miniclient.core.HttpMethod;
import org.miniclient.core.StatusCode;
import org.miniclient.credential.UserCredential;
import org.miniclient.credential.impl.AbstractUserCredential;
import org.miniclient.impl.AbstractRestServiceClient;
import org.miniclient.maker.RestServiceClientMaker;
import org.miniclient.maker.mock.MockRestServiceClientMaker;
import org.miniclient.proxy.DecoratedRestServiceClient;
import org.miniclient.util.ResponseUtil;
// "Mock" object.
// We have a very strange "dual" implementation.
// We use either inheritance or decoration, based on how the object is constructed.
public class MockRestServiceClient extends AbstractRestServiceClient implements DecoratedRestServiceClient, ResourceUrlBuilder, Serializable
{
private static final Logger log = Logger.getLogger(MockRestServiceClient.class.getName());
private static final long serialVersionUID = 1L;
private final RestServiceClient decoratedClient;
// Based on the use of a particular ctor,
// we use either inheritance or decoration.
public MockRestServiceClient(RestServiceClient decoratedClient)
{
this(decoratedClient, (decoratedClient != null) ? decoratedClient.getResourceBaseUrl() : null);
}
public MockRestServiceClient(String resourceBaseUrl)
{
this(null, resourceBaseUrl);
}
// public MockRestServiceClient(ResourceUrlBuilder resourceUrlBuilder)
// {
// super(resourceUrlBuilder);
// }
private MockRestServiceClient(RestServiceClient decoratedClient, String resourceBaseUrl)
{
super(resourceBaseUrl);
this.decoratedClient = decoratedClient;
}
@Override
protected void init()
{
super.init();
// Set the default values.
setAuthCredentialRequired(false); // ???
super.setDefaultAuthCredential(new AbstractUserCredential() {});
setRequestFormat(ContentFormat.JSON);
setResponseFormat(ContentFormat.JSON);
setTimeoutSeconds(10); // ???
// setFollowRedirect(false);
// setMaxFollow(0);
// ...
}
// Factory methods.
@Override
protected RestServiceClientMaker makeRestServiceClientMaker()
{
return MockRestServiceClientMaker.getInstance();
}
// The "main" implementation of the framework (literally)
// We need to override this....
@Override
protected Map process(String method,
UserCredential userCredential, Object inputData, String id,
Map params, boolean retrying) throws IOException
{
if(log.isLoggable(Level.FINE)) log.fine("MockRestServiceClient.get(): method = " + method + "; userCredential = " + userCredential + "; inputData" + inputData + "; id = " + id + "; params = " + params);
// temporary
String endpointUrl = null;
int statusCode = 0;
Object payload = null;
String location = null;
String errorCode = null;
String errorMessage = null;
// errorCode = String.valueOf(statusCode);
// String errorMessage = "Processing failed: statusCode = " + statusCode + " for endpointUrl, " + endpointUrl;
Map response = null;
switch(method) {
case HttpMethod.GET:
endpointUrl = getResourceGetUrl(id, params);
statusCode = StatusCode.OK;
if(id != null && !id.isEmpty()) {
Map obj1 = new HashMap<>();
obj1.put("name", "value");
payload = obj1;
} else {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy