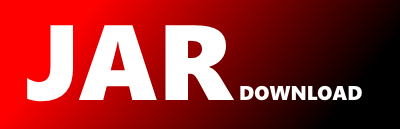
org.miniclient.tool.json.profiler.MiniClientJsonParserRunner Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of miniclient-tool Show documentation
Show all versions of miniclient-tool Show documentation
Universal REST API client library in Java. Tool Module
The newest version!
package org.miniclient.tool.json.profiler;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.Reader;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.miniclient.json.LiteJsonBuilder;
import org.miniclient.json.MiniClientJsonException;
import org.miniclient.json.builder.MiniClientJsonBuilder;
import org.miniclient.json.parser.MiniClientJsonParser;
public class MiniClientJsonParserRunner
{
private static final Logger log = Logger.getLogger(MiniClientJsonParser.class.getName());
private MiniClientJsonParser jsonParser = null;
public MiniClientJsonParserRunner()
{
init();
}
private void init()
{
jsonParser = new MiniClientJsonParser();
}
public void runParse()
{
// Recreate MiniClientJsonParser for every run.
// For profiling purposes. (when done through a loop, we need to re-initialize it at every run).
// init();
String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\mirrorapi.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\random-json0.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\random-json1.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\random-json2.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\random-json3.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\fastjson-bug.json";
// String filePath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\sample.json";
Object node = null;
try {
Reader reader = new FileReader(filePath);
node = jsonParser.parse(reader);
// System.out.println("node = " + node);
String str = node.toString();
// System.out.println("str = " + str);
int len = str.length();
System.out.println("str.lenth = " + len);
} catch (FileNotFoundException e) {
log.log(Level.WARNING, "Failed to find the JSON file: filePath = " + filePath, e);
} catch (IOException e) {
log.log(Level.WARNING, "Failed to parse the JSON file: filePath = " + filePath, e);
} catch (MiniClientJsonException e) {
log.log(Level.WARNING, "Failed to parse the JSON file: filePath = " + filePath, e);
}
// ..
String outputPath = "C:\\Projects\\gitprojects\\glass\\app\\appengine\\miniclient\\extra\\sample-output.json";
// format the jsonStr
LiteJsonBuilder jsonBuilder = new MiniClientJsonBuilder();
// Writer writer = null;
// try {
// writer = new BufferedWriter(new FileWriter(outputPath));
// // jsonBuilder.build(writer, node);
// // jsonBuilder.build(writer, node, 4);
// jsonBuilder.build(writer, node, -1);
// } catch (IOException e) {
// e.printStackTrace();
// }
String jsonStr = null;
try {
jsonStr = jsonBuilder.build(node);
// String jsonStr = jsonBuilder.build(node, 4);
// String jsonStr = jsonBuilder.build(node, -1);
// System.out.println("jsonStr = " + jsonStr);
} catch (MiniClientJsonException e) {
e.printStackTrace();
}
if(jsonStr != null) {
int jsonLen = jsonStr.length();
System.out.println("jsonStr.length = " + jsonLen);
} else {
System.out.println("Failed build JSON string.");
}
}
public static void main(String[] args)
{
System.out.println("Running...");
MiniClientJsonParserRunner runner = new MiniClientJsonParserRunner();
int counter = 0;
while(counter < 200) {
System.out.println(">>>> counter = " + counter);
runner.runParse();
try {
Thread.sleep(10000);
} catch (InterruptedException e) {
e.printStackTrace();
}
++counter;
}
System.out.println("Done.");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy