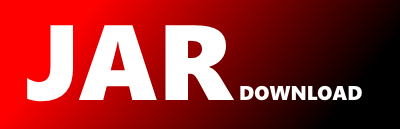
org.afplib.afplib.GSPCOL Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of afplib Show documentation
Show all versions of afplib Show documentation
Java object library able to read and write AFP (Advanced Function Presentation) files.
/**
*/
package org.afplib.afplib;
import org.afplib.base.Triplet;
/**
*
* A representation of the model object 'GSPCOL'.
*
*
*
* The following features are supported:
*
*
* - {@link org.afplib.afplib.GSPCOL#getRES1 RES1}
* - {@link org.afplib.afplib.GSPCOL#getCOLSPCE COLSPCE}
* - {@link org.afplib.afplib.GSPCOL#getRES2 RES2}
* - {@link org.afplib.afplib.GSPCOL#getCOLSIZE1 COLSIZE1}
* - {@link org.afplib.afplib.GSPCOL#getCOLSIZE2 COLSIZE2}
* - {@link org.afplib.afplib.GSPCOL#getCOLSIZE3 COLSIZE3}
* - {@link org.afplib.afplib.GSPCOL#getCOLSIZE4 COLSIZE4}
* - {@link org.afplib.afplib.GSPCOL#getCOLVALUE COLVALUE}
*
*
* @see org.afplib.afplib.AfplibPackage#getGSPCOL()
* @model
* @generated
*/
public interface GSPCOL extends Triplet {
/**
* Returns the value of the 'RES1' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'RES1' attribute.
* @see #setRES1(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_RES1()
* @model required="true"
* @generated
*/
Integer getRES1();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getRES1 RES1}' attribute.
*
*
* @param value the new value of the 'RES1' attribute.
* @see #getRES1()
* @generated
*/
void setRES1(Integer value);
/**
* Returns the value of the 'COLSPCE' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'COLSPCE' attribute.
* @see #setCOLSPCE(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLSPCE()
* @model required="true"
* @generated
*/
Integer getCOLSPCE();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLSPCE COLSPCE}' attribute.
*
*
* @param value the new value of the 'COLSPCE' attribute.
* @see #getCOLSPCE()
* @generated
*/
void setCOLSPCE(Integer value);
/**
* Returns the value of the 'RES2' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'RES2' attribute.
* @see #setRES2(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_RES2()
* @model required="true"
* @generated
*/
Integer getRES2();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getRES2 RES2}' attribute.
*
*
* @param value the new value of the 'RES2' attribute.
* @see #getRES2()
* @generated
*/
void setRES2(Integer value);
/**
* Returns the value of the 'COLSIZE1' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'COLSIZE1' attribute.
* @see #setCOLSIZE1(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLSIZE1()
* @model required="true"
* @generated
*/
Integer getCOLSIZE1();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLSIZE1 COLSIZE1}' attribute.
*
*
* @param value the new value of the 'COLSIZE1' attribute.
* @see #getCOLSIZE1()
* @generated
*/
void setCOLSIZE1(Integer value);
/**
* Returns the value of the 'COLSIZE2' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'COLSIZE2' attribute.
* @see #setCOLSIZE2(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLSIZE2()
* @model required="true"
* @generated
*/
Integer getCOLSIZE2();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLSIZE2 COLSIZE2}' attribute.
*
*
* @param value the new value of the 'COLSIZE2' attribute.
* @see #getCOLSIZE2()
* @generated
*/
void setCOLSIZE2(Integer value);
/**
* Returns the value of the 'COLSIZE3' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'COLSIZE3' attribute.
* @see #setCOLSIZE3(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLSIZE3()
* @model required="true"
* @generated
*/
Integer getCOLSIZE3();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLSIZE3 COLSIZE3}' attribute.
*
*
* @param value the new value of the 'COLSIZE3' attribute.
* @see #getCOLSIZE3()
* @generated
*/
void setCOLSIZE3(Integer value);
/**
* Returns the value of the 'COLSIZE4' attribute.
*
*
*
* mandatory
fixed length
*
* @return the value of the 'COLSIZE4' attribute.
* @see #setCOLSIZE4(Integer)
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLSIZE4()
* @model required="true"
* @generated
*/
Integer getCOLSIZE4();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLSIZE4 COLSIZE4}' attribute.
*
*
* @param value the new value of the 'COLSIZE4' attribute.
* @see #getCOLSIZE4()
* @generated
*/
void setCOLSIZE4(Integer value);
/**
* Returns the value of the 'COLVALUE' attribute.
*
*
*
* mandatory
variable length
*
* @return the value of the 'COLVALUE' attribute.
* @see #setCOLVALUE(byte[])
* @see org.afplib.afplib.AfplibPackage#getGSPCOL_COLVALUE()
* @model required="true"
* @generated
*/
byte[] getCOLVALUE();
/**
* Sets the value of the '{@link org.afplib.afplib.GSPCOL#getCOLVALUE COLVALUE}' attribute.
*
*
* @param value the new value of the 'COLVALUE' attribute.
* @see #getCOLVALUE()
* @generated
*/
void setCOLVALUE(byte[] value);
} // GSPCOL
© 2015 - 2025 Weber Informatics LLC | Privacy Policy