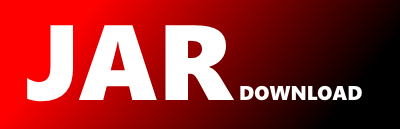
org.afplib.base.SF Maven / Gradle / Ivy
/**
*/
package org.afplib.base;
import java.nio.charset.Charset;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.ecore.EObject;
/**
*
* A representation of the model object 'SF'.
*
*
*
* The following features are supported:
*
*
* - {@link org.afplib.base.SF#getNumber Number}
* - {@link org.afplib.base.SF#getOffset Offset}
* - {@link org.afplib.base.SF#getId Id}
* - {@link org.afplib.base.SF#getLength Length}
* - {@link org.afplib.base.SF#getChildren Children}
* - {@link org.afplib.base.SF#getRawData Raw Data}
* - {@link org.afplib.base.SF#getCharset Charset}
*
*
* @see org.afplib.base.BasePackage#getSF()
* @model abstract="true"
* @generated
*/
public interface SF extends EObject {
/**
* Returns the value of the 'Number' attribute.
*
*
* If the meaning of the 'Number' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Number' attribute.
* @see #setNumber(long)
* @see org.afplib.base.BasePackage#getSF_Number()
* @model
* @generated
*/
long getNumber();
/**
* Sets the value of the '{@link org.afplib.base.SF#getNumber Number}' attribute.
*
*
* @param value the new value of the 'Number' attribute.
* @see #getNumber()
* @generated
*/
void setNumber(long value);
/**
* Returns the value of the 'Offset' attribute.
*
*
* If the meaning of the 'Offset' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Offset' attribute.
* @see #setOffset(long)
* @see org.afplib.base.BasePackage#getSF_Offset()
* @model
* @generated
*/
long getOffset();
/**
* Sets the value of the '{@link org.afplib.base.SF#getOffset Offset}' attribute.
*
*
* @param value the new value of the 'Offset' attribute.
* @see #getOffset()
* @generated
*/
void setOffset(long value);
/**
* Returns the value of the 'Id' attribute.
*
*
* If the meaning of the 'Id' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Id' attribute.
* @see #setId(int)
* @see org.afplib.base.BasePackage#getSF_Id()
* @model
* @generated
*/
int getId();
/**
* Sets the value of the '{@link org.afplib.base.SF#getId Id}' attribute.
*
*
* @param value the new value of the 'Id' attribute.
* @see #getId()
* @generated
*/
void setId(int value);
/**
* Returns the value of the 'Length' attribute.
*
*
* If the meaning of the 'Length' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Length' attribute.
* @see #setLength(int)
* @see org.afplib.base.BasePackage#getSF_Length()
* @model
* @generated
*/
int getLength();
/**
* Sets the value of the '{@link org.afplib.base.SF#getLength Length}' attribute.
*
*
* @param value the new value of the 'Length' attribute.
* @see #getLength()
* @generated
*/
void setLength(int value);
/**
* Returns the value of the 'Children' containment reference list.
* The list contents are of type {@link org.afplib.base.SF}.
*
*
* If the meaning of the 'Children' containment reference list isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Children' containment reference list.
* @see org.afplib.base.BasePackage#getSF_Children()
* @model containment="true" resolveProxies="true"
* @generated
*/
EList getChildren();
/**
* Returns the value of the 'Raw Data' attribute.
*
*
* If the meaning of the 'Raw Data' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Raw Data' attribute.
* @see #setRawData(byte[])
* @see org.afplib.base.BasePackage#getSF_RawData()
* @model
* @generated
*/
byte[] getRawData();
/**
* Sets the value of the '{@link org.afplib.base.SF#getRawData Raw Data}' attribute.
*
*
* @param value the new value of the 'Raw Data' attribute.
* @see #getRawData()
* @generated
*/
void setRawData(byte[] value);
/**
* Returns the value of the 'Charset' attribute.
*
*
* If the meaning of the 'Charset' attribute isn't clear,
* there really should be more of a description here...
*
*
* @return the value of the 'Charset' attribute.
* @see #setCharset(Charset)
* @see org.afplib.base.BasePackage#getSF_Charset()
* @model dataType="org.afplib.base.Charset"
* @generated
*/
Charset getCharset();
/**
* Sets the value of the '{@link org.afplib.base.SF#getCharset Charset}' attribute.
*
*
* @param value the new value of the 'Charset' attribute.
* @see #getCharset()
* @generated
*/
void setCharset(Charset value);
/**
*
*
* @model kind="operation"
* @generated
*/
boolean isBegin();
/**
*
*
* @model kind="operation"
* @generated
*/
boolean isEnd();
/**
*
*
* @model
* @generated
*/
boolean isDefault(String value);
} // SF