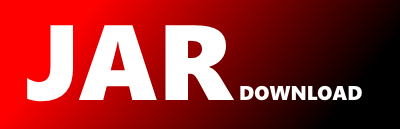
org.agileware.test.AbstractTester Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of smartunit Show documentation
Show all versions of smartunit Show documentation
Unit test utilities library
The newest version!
package org.agileware.test;
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import java.sql.Date;
import java.sql.Time;
import java.sql.Timestamp;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.TreeMap;
import java.util.TreeSet;
import org.mockito.Mockito;
public abstract class AbstractTester> {
protected Map, ValueBuilder>> mappings = new HashMap, ValueBuilder>>();
public AbstractTester() {
// primitives
mappings.put(boolean.class, new ValueBuilder() {
public Boolean build() {
return new Boolean((Math.random() * 100 % 2) == 0);
}
});
mappings.put(Boolean.class, new ValueBuilder() {
public Boolean build() {
return new Boolean((Math.random() * 100 % 2) == 0);
}
});
mappings.put(byte.class, new ValueBuilder() {
public Byte build() {
return new Byte((byte) (Math.random() * Byte.MAX_VALUE));
}
});
mappings.put(Byte.class, new ValueBuilder() {
public Byte build() {
return new Byte((byte) (Math.random() * Byte.MAX_VALUE));
}
});
mappings.put(char.class, new ValueBuilder() {
public Character build() {
return new Character((char) (Math.random() * Character.MAX_VALUE));
}
});
mappings.put(Character.class, new ValueBuilder() {
public Character build() {
return new Character((char) (Math.random() * Character.MAX_VALUE));
}
});
mappings.put(short.class, new ValueBuilder() {
public Short build() {
return new Short((short) (Math.random() * Short.MAX_VALUE));
}
});
mappings.put(Short.class, new ValueBuilder() {
public Short build() {
return new Short((short) (Math.random() * Short.MAX_VALUE));
}
});
mappings.put(int.class, new ValueBuilder() {
public Integer build() {
return new Integer((int) (Math.random() * Integer.MAX_VALUE));
}
});
mappings.put(Integer.class, new ValueBuilder() {
public Integer build() {
return new Integer((int) (Math.random() * Integer.MAX_VALUE));
}
});
mappings.put(long.class, new ValueBuilder() {
public Long build() {
return new Long((long) (Math.random() * Long.MAX_VALUE));
}
});
mappings.put(Long.class, new ValueBuilder() {
public Long build() {
return new Long((long) (Math.random() * Long.MAX_VALUE));
}
});
mappings.put(float.class, new ValueBuilder() {
public Float build() {
return new Float((float) (Math.random() * Float.MAX_VALUE));
}
});
mappings.put(Float.class, new ValueBuilder() {
public Float build() {
return new Float((float) (Math.random() * Float.MAX_VALUE));
}
});
mappings.put(double.class, new ValueBuilder() {
public Double build() {
return new Double((double) (Math.random() * Double.MAX_VALUE));
}
});
mappings.put(Double.class, new ValueBuilder() {
public Double build() {
return new Double((double) (Math.random() * Double.MAX_VALUE));
}
});
// collections
mappings.put(Collection.class, new ValueBuilder>() {
public Collection> build() {
return new ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy