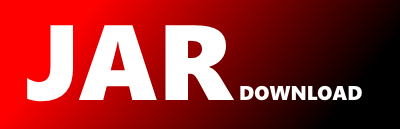
org.aiflow.notification.proto.NotificationServiceGrpc Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of notification-client Show documentation
Show all versions of notification-client Show documentation
A java client for AIFlow notification service.
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing,
* software distributed under the License is distributed on an
* "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY
* KIND, either express or implied. See the License for the
* specific language governing permissions and limitations
* under the License.
*/
package org.aiflow.notification.proto;
import static io.grpc.MethodDescriptor.generateFullMethodName;
import static io.grpc.stub.ClientCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ClientCalls.asyncClientStreamingCall;
import static io.grpc.stub.ClientCalls.asyncServerStreamingCall;
import static io.grpc.stub.ClientCalls.asyncUnaryCall;
import static io.grpc.stub.ClientCalls.blockingServerStreamingCall;
import static io.grpc.stub.ClientCalls.blockingUnaryCall;
import static io.grpc.stub.ClientCalls.futureUnaryCall;
import static io.grpc.stub.ServerCalls.asyncBidiStreamingCall;
import static io.grpc.stub.ServerCalls.asyncClientStreamingCall;
import static io.grpc.stub.ServerCalls.asyncServerStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnaryCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedStreamingCall;
import static io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall;
/**
*
* AirFlowService provides notification function rest endpoint of NotificationService for Notification Service component.
* Functions of NotificationService include:
* 1.Send event.
* 2.List events.
*
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.29.0-SNAPSHOT)",
comments = "Source: notification_service.proto")
public final class NotificationServiceGrpc {
private NotificationServiceGrpc() {}
public static final String SERVICE_NAME = "notification_service.NotificationService";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getSendEventMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "sendEvent",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getSendEventMethod() {
io.grpc.MethodDescriptor getSendEventMethod;
if ((getSendEventMethod = NotificationServiceGrpc.getSendEventMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getSendEventMethod = NotificationServiceGrpc.getSendEventMethod) == null) {
NotificationServiceGrpc.getSendEventMethod = getSendEventMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "sendEvent"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventsResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("sendEvent"))
.build();
}
}
}
return getSendEventMethod;
}
private static volatile io.grpc.MethodDescriptor getListEventsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "listEvents",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListEventsMethod() {
io.grpc.MethodDescriptor getListEventsMethod;
if ((getListEventsMethod = NotificationServiceGrpc.getListEventsMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getListEventsMethod = NotificationServiceGrpc.getListEventsMethod) == null) {
NotificationServiceGrpc.getListEventsMethod = getListEventsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "listEvents"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("listEvents"))
.build();
}
}
}
return getListEventsMethod;
}
private static volatile io.grpc.MethodDescriptor getListAllEventsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "listAllEvents",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListAllEventsMethod() {
io.grpc.MethodDescriptor getListAllEventsMethod;
if ((getListAllEventsMethod = NotificationServiceGrpc.getListAllEventsMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getListAllEventsMethod = NotificationServiceGrpc.getListAllEventsMethod) == null) {
NotificationServiceGrpc.getListAllEventsMethod = getListAllEventsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "listAllEvents"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("listAllEvents"))
.build();
}
}
}
return getListAllEventsMethod;
}
private static volatile io.grpc.MethodDescriptor getNotifyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "notify",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getNotifyMethod() {
io.grpc.MethodDescriptor getNotifyMethod;
if ((getNotifyMethod = NotificationServiceGrpc.getNotifyMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getNotifyMethod = NotificationServiceGrpc.getNotifyMethod) == null) {
NotificationServiceGrpc.getNotifyMethod = getNotifyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "notify"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("notify"))
.build();
}
}
}
return getNotifyMethod;
}
private static volatile io.grpc.MethodDescriptor getListMembersMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "listMembers",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListMembersMethod() {
io.grpc.MethodDescriptor getListMembersMethod;
if ((getListMembersMethod = NotificationServiceGrpc.getListMembersMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getListMembersMethod = NotificationServiceGrpc.getListMembersMethod) == null) {
NotificationServiceGrpc.getListMembersMethod = getListMembersMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "listMembers"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("listMembers"))
.build();
}
}
}
return getListMembersMethod;
}
private static volatile io.grpc.MethodDescriptor getNotifyNewMemberMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "notifyNewMember",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getNotifyNewMemberMethod() {
io.grpc.MethodDescriptor getNotifyNewMemberMethod;
if ((getNotifyNewMemberMethod = NotificationServiceGrpc.getNotifyNewMemberMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getNotifyNewMemberMethod = NotificationServiceGrpc.getNotifyNewMemberMethod) == null) {
NotificationServiceGrpc.getNotifyNewMemberMethod = getNotifyNewMemberMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "notifyNewMember"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("notifyNewMember"))
.build();
}
}
}
return getNotifyNewMemberMethod;
}
private static volatile io.grpc.MethodDescriptor getGetLatestVersionByKeyMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "getLatestVersionByKey",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getGetLatestVersionByKeyMethod() {
io.grpc.MethodDescriptor getGetLatestVersionByKeyMethod;
if ((getGetLatestVersionByKeyMethod = NotificationServiceGrpc.getGetLatestVersionByKeyMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getGetLatestVersionByKeyMethod = NotificationServiceGrpc.getGetLatestVersionByKeyMethod) == null) {
NotificationServiceGrpc.getGetLatestVersionByKeyMethod = getGetLatestVersionByKeyMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "getLatestVersionByKey"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("getLatestVersionByKey"))
.build();
}
}
}
return getGetLatestVersionByKeyMethod;
}
private static volatile io.grpc.MethodDescriptor getRegisterClientMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "registerClient",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRegisterClientMethod() {
io.grpc.MethodDescriptor getRegisterClientMethod;
if ((getRegisterClientMethod = NotificationServiceGrpc.getRegisterClientMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getRegisterClientMethod = NotificationServiceGrpc.getRegisterClientMethod) == null) {
NotificationServiceGrpc.getRegisterClientMethod = getRegisterClientMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "registerClient"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("registerClient"))
.build();
}
}
}
return getRegisterClientMethod;
}
private static volatile io.grpc.MethodDescriptor getDeleteClientMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "deleteClient",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getDeleteClientMethod() {
io.grpc.MethodDescriptor getDeleteClientMethod;
if ((getDeleteClientMethod = NotificationServiceGrpc.getDeleteClientMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getDeleteClientMethod = NotificationServiceGrpc.getDeleteClientMethod) == null) {
NotificationServiceGrpc.getDeleteClientMethod = getDeleteClientMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "deleteClient"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("deleteClient"))
.build();
}
}
}
return getDeleteClientMethod;
}
private static volatile io.grpc.MethodDescriptor getIsClientExistsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "isClientExists",
requestType = org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest.class,
responseType = org.aiflow.notification.proto.NotificationServiceOuterClass.isClientExistsResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getIsClientExistsMethod() {
io.grpc.MethodDescriptor getIsClientExistsMethod;
if ((getIsClientExistsMethod = NotificationServiceGrpc.getIsClientExistsMethod) == null) {
synchronized (NotificationServiceGrpc.class) {
if ((getIsClientExistsMethod = NotificationServiceGrpc.getIsClientExistsMethod) == null) {
NotificationServiceGrpc.getIsClientExistsMethod = getIsClientExistsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "isClientExists"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
org.aiflow.notification.proto.NotificationServiceOuterClass.isClientExistsResponse.getDefaultInstance()))
.setSchemaDescriptor(new NotificationServiceMethodDescriptorSupplier("isClientExists"))
.build();
}
}
}
return getIsClientExistsMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static NotificationServiceStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public NotificationServiceStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceStub(channel, callOptions);
}
};
return NotificationServiceStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static NotificationServiceBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public NotificationServiceBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceBlockingStub(channel, callOptions);
}
};
return NotificationServiceBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static NotificationServiceFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public NotificationServiceFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceFutureStub(channel, callOptions);
}
};
return NotificationServiceFutureStub.newStub(factory, channel);
}
/**
*
* AirFlowService provides notification function rest endpoint of NotificationService for Notification Service component.
* Functions of NotificationService include:
* 1.Send event.
* 2.List events.
*
*/
public static abstract class NotificationServiceImplBase implements io.grpc.BindableService {
/**
*
* Send event.
*
*/
public void sendEvent(org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getSendEventMethod(), responseObserver);
}
/**
*
* List events.
*
*/
public void listEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getListEventsMethod(), responseObserver);
}
/**
*
* List all events
*
*/
public void listAllEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getListAllEventsMethod(), responseObserver);
}
/**
*
* Accepts notifications from other members.
*
*/
public void notify(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getNotifyMethod(), responseObserver);
}
/**
*
* List current living members.
*
*/
public void listMembers(org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getListMembersMethod(), responseObserver);
}
/**
*
* Notify current members that there is a new member added.
*
*/
public void notifyNewMember(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getNotifyNewMemberMethod(), responseObserver);
}
/**
*
* Get latest version by key
*
*/
public void getLatestVersionByKey(org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getGetLatestVersionByKeyMethod(), responseObserver);
}
/**
*
* Register notification client in the db of notification service
*
*/
public void registerClient(org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getRegisterClientMethod(), responseObserver);
}
/**
*
* Delete notification client
*
*/
public void deleteClient(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getDeleteClientMethod(), responseObserver);
}
/**
*
* Check if a notification client has been registered in the db
*
*/
public void isClientExists(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnimplementedUnaryCall(getIsClientExistsMethod(), responseObserver);
}
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getSendEventMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventsResponse>(
this, METHODID_SEND_EVENT)))
.addMethod(
getListEventsMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse>(
this, METHODID_LIST_EVENTS)))
.addMethod(
getListAllEventsMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse>(
this, METHODID_LIST_ALL_EVENTS)))
.addMethod(
getNotifyMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse>(
this, METHODID_NOTIFY)))
.addMethod(
getListMembersMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersResponse>(
this, METHODID_LIST_MEMBERS)))
.addMethod(
getNotifyNewMemberMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse>(
this, METHODID_NOTIFY_NEW_MEMBER)))
.addMethod(
getGetLatestVersionByKeyMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionResponse>(
this, METHODID_GET_LATEST_VERSION_BY_KEY)))
.addMethod(
getRegisterClientMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientResponse>(
this, METHODID_REGISTER_CLIENT)))
.addMethod(
getDeleteClientMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse>(
this, METHODID_DELETE_CLIENT)))
.addMethod(
getIsClientExistsMethod(),
asyncUnaryCall(
new MethodHandlers<
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest,
org.aiflow.notification.proto.NotificationServiceOuterClass.isClientExistsResponse>(
this, METHODID_IS_CLIENT_EXISTS)))
.build();
}
}
/**
*
* AirFlowService provides notification function rest endpoint of NotificationService for Notification Service component.
* Functions of NotificationService include:
* 1.Send event.
* 2.List events.
*
*/
public static final class NotificationServiceStub extends io.grpc.stub.AbstractAsyncStub {
private NotificationServiceStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected NotificationServiceStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceStub(channel, callOptions);
}
/**
*
* Send event.
*
*/
public void sendEvent(org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getSendEventMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List events.
*
*/
public void listEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getListEventsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List all events
*
*/
public void listAllEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getListAllEventsMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Accepts notifications from other members.
*
*/
public void notify(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getNotifyMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* List current living members.
*
*/
public void listMembers(org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getListMembersMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Notify current members that there is a new member added.
*
*/
public void notifyNewMember(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getNotifyNewMemberMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Get latest version by key
*
*/
public void getLatestVersionByKey(org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getGetLatestVersionByKeyMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Register notification client in the db of notification service
*
*/
public void registerClient(org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getRegisterClientMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Delete notification client
*
*/
public void deleteClient(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getDeleteClientMethod(), getCallOptions()), request, responseObserver);
}
/**
*
* Check if a notification client has been registered in the db
*
*/
public void isClientExists(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request,
io.grpc.stub.StreamObserver responseObserver) {
asyncUnaryCall(
getChannel().newCall(getIsClientExistsMethod(), getCallOptions()), request, responseObserver);
}
}
/**
*
* AirFlowService provides notification function rest endpoint of NotificationService for Notification Service component.
* Functions of NotificationService include:
* 1.Send event.
* 2.List events.
*
*/
public static final class NotificationServiceBlockingStub extends io.grpc.stub.AbstractBlockingStub {
private NotificationServiceBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected NotificationServiceBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceBlockingStub(channel, callOptions);
}
/**
*
* Send event.
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventsResponse sendEvent(org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest request) {
return blockingUnaryCall(
getChannel(), getSendEventMethod(), getCallOptions(), request);
}
/**
*
* List events.
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse listEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest request) {
return blockingUnaryCall(
getChannel(), getListEventsMethod(), getCallOptions(), request);
}
/**
*
* List all events
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsResponse listAllEvents(org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest request) {
return blockingUnaryCall(
getChannel(), getListAllEventsMethod(), getCallOptions(), request);
}
/**
*
* Accepts notifications from other members.
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse notify(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest request) {
return blockingUnaryCall(
getChannel(), getNotifyMethod(), getCallOptions(), request);
}
/**
*
* List current living members.
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersResponse listMembers(org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest request) {
return blockingUnaryCall(
getChannel(), getListMembersMethod(), getCallOptions(), request);
}
/**
*
* Notify current members that there is a new member added.
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse notifyNewMember(org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest request) {
return blockingUnaryCall(
getChannel(), getNotifyNewMemberMethod(), getCallOptions(), request);
}
/**
*
* Get latest version by key
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionResponse getLatestVersionByKey(org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest request) {
return blockingUnaryCall(
getChannel(), getGetLatestVersionByKeyMethod(), getCallOptions(), request);
}
/**
*
* Register notification client in the db of notification service
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientResponse registerClient(org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest request) {
return blockingUnaryCall(
getChannel(), getRegisterClientMethod(), getCallOptions(), request);
}
/**
*
* Delete notification client
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.CommonResponse deleteClient(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request) {
return blockingUnaryCall(
getChannel(), getDeleteClientMethod(), getCallOptions(), request);
}
/**
*
* Check if a notification client has been registered in the db
*
*/
public org.aiflow.notification.proto.NotificationServiceOuterClass.isClientExistsResponse isClientExists(org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request) {
return blockingUnaryCall(
getChannel(), getIsClientExistsMethod(), getCallOptions(), request);
}
}
/**
*
* AirFlowService provides notification function rest endpoint of NotificationService for Notification Service component.
* Functions of NotificationService include:
* 1.Send event.
* 2.List events.
*
*/
public static final class NotificationServiceFutureStub extends io.grpc.stub.AbstractFutureStub {
private NotificationServiceFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected NotificationServiceFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new NotificationServiceFutureStub(channel, callOptions);
}
/**
*
* Send event.
*
*/
public com.google.common.util.concurrent.ListenableFuture sendEvent(
org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest request) {
return futureUnaryCall(
getChannel().newCall(getSendEventMethod(), getCallOptions()), request);
}
/**
*
* List events.
*
*/
public com.google.common.util.concurrent.ListenableFuture listEvents(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest request) {
return futureUnaryCall(
getChannel().newCall(getListEventsMethod(), getCallOptions()), request);
}
/**
*
* List all events
*
*/
public com.google.common.util.concurrent.ListenableFuture listAllEvents(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest request) {
return futureUnaryCall(
getChannel().newCall(getListAllEventsMethod(), getCallOptions()), request);
}
/**
*
* Accepts notifications from other members.
*
*/
public com.google.common.util.concurrent.ListenableFuture notify(
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest request) {
return futureUnaryCall(
getChannel().newCall(getNotifyMethod(), getCallOptions()), request);
}
/**
*
* List current living members.
*
*/
public com.google.common.util.concurrent.ListenableFuture listMembers(
org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest request) {
return futureUnaryCall(
getChannel().newCall(getListMembersMethod(), getCallOptions()), request);
}
/**
*
* Notify current members that there is a new member added.
*
*/
public com.google.common.util.concurrent.ListenableFuture notifyNewMember(
org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest request) {
return futureUnaryCall(
getChannel().newCall(getNotifyNewMemberMethod(), getCallOptions()), request);
}
/**
*
* Get latest version by key
*
*/
public com.google.common.util.concurrent.ListenableFuture getLatestVersionByKey(
org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest request) {
return futureUnaryCall(
getChannel().newCall(getGetLatestVersionByKeyMethod(), getCallOptions()), request);
}
/**
*
* Register notification client in the db of notification service
*
*/
public com.google.common.util.concurrent.ListenableFuture registerClient(
org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest request) {
return futureUnaryCall(
getChannel().newCall(getRegisterClientMethod(), getCallOptions()), request);
}
/**
*
* Delete notification client
*
*/
public com.google.common.util.concurrent.ListenableFuture deleteClient(
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request) {
return futureUnaryCall(
getChannel().newCall(getDeleteClientMethod(), getCallOptions()), request);
}
/**
*
* Check if a notification client has been registered in the db
*
*/
public com.google.common.util.concurrent.ListenableFuture isClientExists(
org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest request) {
return futureUnaryCall(
getChannel().newCall(getIsClientExistsMethod(), getCallOptions()), request);
}
}
private static final int METHODID_SEND_EVENT = 0;
private static final int METHODID_LIST_EVENTS = 1;
private static final int METHODID_LIST_ALL_EVENTS = 2;
private static final int METHODID_NOTIFY = 3;
private static final int METHODID_LIST_MEMBERS = 4;
private static final int METHODID_NOTIFY_NEW_MEMBER = 5;
private static final int METHODID_GET_LATEST_VERSION_BY_KEY = 6;
private static final int METHODID_REGISTER_CLIENT = 7;
private static final int METHODID_DELETE_CLIENT = 8;
private static final int METHODID_IS_CLIENT_EXISTS = 9;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final NotificationServiceImplBase serviceImpl;
private final int methodId;
MethodHandlers(NotificationServiceImplBase serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_SEND_EVENT:
serviceImpl.sendEvent((org.aiflow.notification.proto.NotificationServiceOuterClass.SendEventRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_EVENTS:
serviceImpl.listEvents((org.aiflow.notification.proto.NotificationServiceOuterClass.ListEventsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_ALL_EVENTS:
serviceImpl.listAllEvents((org.aiflow.notification.proto.NotificationServiceOuterClass.ListAllEventsRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_NOTIFY:
serviceImpl.notify((org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_MEMBERS:
serviceImpl.listMembers((org.aiflow.notification.proto.NotificationServiceOuterClass.ListMembersRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_NOTIFY_NEW_MEMBER:
serviceImpl.notifyNewMember((org.aiflow.notification.proto.NotificationServiceOuterClass.NotifyNewMemberRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_GET_LATEST_VERSION_BY_KEY:
serviceImpl.getLatestVersionByKey((org.aiflow.notification.proto.NotificationServiceOuterClass.GetLatestVersionByKeyRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_REGISTER_CLIENT:
serviceImpl.registerClient((org.aiflow.notification.proto.NotificationServiceOuterClass.RegisterClientRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_DELETE_CLIENT:
serviceImpl.deleteClient((org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_IS_CLIENT_EXISTS:
serviceImpl.isClientExists((org.aiflow.notification.proto.NotificationServiceOuterClass.ClientIdRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
private static abstract class NotificationServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
NotificationServiceBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return org.aiflow.notification.proto.NotificationServiceOuterClass.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("NotificationService");
}
}
private static final class NotificationServiceFileDescriptorSupplier
extends NotificationServiceBaseDescriptorSupplier {
NotificationServiceFileDescriptorSupplier() {}
}
private static final class NotificationServiceMethodDescriptorSupplier
extends NotificationServiceBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final String methodName;
NotificationServiceMethodDescriptorSupplier(String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (NotificationServiceGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new NotificationServiceFileDescriptorSupplier())
.addMethod(getSendEventMethod())
.addMethod(getListEventsMethod())
.addMethod(getListAllEventsMethod())
.addMethod(getNotifyMethod())
.addMethod(getListMembersMethod())
.addMethod(getNotifyNewMemberMethod())
.addMethod(getGetLatestVersionByKeyMethod())
.addMethod(getRegisterClientMethod())
.addMethod(getDeleteClientMethod())
.addMethod(getIsClientExistsMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy