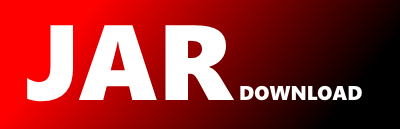
org.ajoberstar.grgit.Status.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grgit-core Show documentation
Show all versions of grgit-core Show documentation
The Groovy way to use Git
package org.ajoberstar.grgit
import groovy.transform.EqualsAndHashCode
import groovy.transform.ToString
/**
* Status of the current working tree and index.
*/
@EqualsAndHashCode
@ToString(includeNames=true)
class Status {
final Changes staged
final Changes unstaged
final Set conflicts
Status(Map args = [:]) {
def invalidArgs = args.keySet() - ['staged', 'unstaged', 'conflicts']
if (invalidArgs) {
throw new IllegalArgumentException("Following keys are not supported: ${invalidArgs}")
}
this.staged = 'staged' in args ? new Changes(args.staged) : new Changes()
this.unstaged = 'unstaged' in args ? new Changes(args.unstaged) : new Changes()
this.conflicts = 'conflicts' in args ? args.conflicts : []
}
@EqualsAndHashCode
@ToString(includeNames=true)
class Changes {
final Set added
final Set modified
final Set removed
Changes(Map args = [:]) {
def invalidArgs = args.keySet() - ['added', 'modified', 'removed']
if (invalidArgs) {
throw new IllegalArgumentException("Following keys are not supported: ${invalidArgs}")
}
this.added = 'added' in args ? args.added : []
this.modified = 'modified' in args ? args.modified : []
this.removed = 'removed' in args ? args.removed : []
}
/**
* Gets all changed files.
* @return all changed files
*/
Set getAllChanges() {
return added + modified + removed
}
}
/**
* Whether the repository has any changes or conflicts.
* @return {@code true} if there are no changes either staged or unstaged or
* any conflicts, {@code false} otherwise
*/
boolean isClean() {
return (staged.allChanges + unstaged.allChanges + conflicts).empty
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy