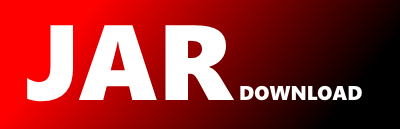
org.ajoberstar.grgit.operation.CheckoutOp.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of grgit Show documentation
Show all versions of grgit Show documentation
The Groovy way to use Git.
/*
* Copyright 2012-2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ajoberstar.grgit.operation
import java.util.concurrent.Callable
import org.ajoberstar.grgit.Repository
import org.ajoberstar.grgit.exception.GrgitException
import org.ajoberstar.grgit.service.ResolveService
import org.eclipse.jgit.api.CheckoutCommand
import org.eclipse.jgit.api.errors.GitAPIException
/**
* Checks out a branch to the working tree. Does not support checking out
* specific paths.
*
* To checkout an existing branch.
*
*
* grgit.checkout(branch: 'existing-branch')
* grgit.checkout(branch: 'existing-branch', createBranch: false)
*
*
* To checkout a new branch starting at, but not tracking, the current HEAD.
*
*
* grgit.checkout(branch: 'new-branch', createBranch: true)
*
*
* To checkout a new branch starting at, but not tracking, a start point.
*
*
* grgit.checkout(branch: 'new-branch', startPoint: 'any-branch', createBranch: true)
*
*
* To checkout a new orphan branch starting at, but not tracking, the current HEAD.
*
*
* grgit.checkout(branch: 'new-branch', orphan: true)
*
*
* To checkout a new orphan branch starting at, but not tracking, a start point.
*
*
* grgit.checkout(branch: 'new-branch', startPoint: 'any-branch', orphan: true)
*
*
* See git-checkout Manual Page.
*
* @since 0.1.0
* @see git-checkout Manual Page
*/
class CheckoutOp implements Callable {
private final Repository repo
/**
* The branch or commit to checkout.
* @see {@link ResolveService#toBranchName(Object)}
*/
Object branch
/**
* {@code true} if the branch does not exist and should be created,
* {@code false} (the default) otherwise
*/
boolean createBranch = false
/**
* If {@code createBranch} or {@code orphan} is {@code true}, start the new branch
* at this commit.
* @see {@link ResolveService#toRevisionString(Object)}
*/
Object startPoint
/**
* {@code true} if the new branch is to be an orphan,
* {@code false} (the default) otherwise
*/
boolean orphan = false
CheckoutOp(Repository repo) {
this.repo = repo
}
Void call() {
if (startPoint && !createBranch && !orphan) {
throw new IllegalArgumentException('Cannot set a start point if createBranch and orphan are false.')
} else if ((createBranch || orphan) && !branch) {
throw new IllegalArgumentException('Must specify branch name to create.')
}
CheckoutCommand cmd = repo.jgit.checkout()
ResolveService resolve = new ResolveService(repo)
if (branch) { cmd.name = resolve.toBranchName(branch) }
cmd.createBranch = createBranch
cmd.startPoint = resolve.toRevisionString(startPoint)
cmd.orphan = orphan
try {
cmd.call()
return null
} catch (GitAPIException e) {
throw new GrgitException('Problem checking out.', e)
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy