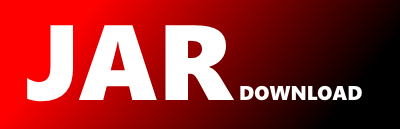
org.ajoberstar.grgit.Grgit.groovy Maven / Gradle / Ivy
Show all versions of grgit Show documentation
/*
* Copyright 2012-2015 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.ajoberstar.grgit
import org.ajoberstar.grgit.operation.*
import org.ajoberstar.grgit.service.*
import org.ajoberstar.grgit.util.JGitUtil
import org.ajoberstar.grgit.util.OpSyntaxUtil
import org.eclipse.jgit.api.Git
/**
* Provides support for performaing operations on and getting information about
* a Git repository.
*
* A Grgit instance can be obtained via 3 methods.
*
*
* -
*
{@link org.ajoberstar.grgit.operation.OpenOp Open} an existing repository.
* def grgit = Grgit.open(dir: 'path/to/my/repo')
*
* -
*
{@link org.ajoberstar.grgit.operation.InitOp Initialize} a new repository.
* def grgit = Grgit.init(dir: 'path/to/my/repo')
*
* -
*
{@link org.ajoberstar.grgit.operation.CloneOp Clone} an existing repository.
* def grgit = Grgit.clone(dir: 'path/to/my/repo', uri: '[email protected]:ajoberstar/grgit.git')
*
*
*
*
* Once obtained, operations can be called with two syntaxes.
*
*
*
* -
*
Map syntax. Any public property on the {@code *Op} class can be provided as a Map entry.
* grgit.commit(message: 'Committing my code.', amend: true)
*
* -
*
Closure syntax. Any public property or method on the {@code *Op} class can be used.
*
* grgit.log {
* range 'master', 'my-new-branch'
* maxCommits = 5
* }
*
*
*
*
*
* Details of each operation's properties and methods are available on the
* doc page for the class. The following operations are supported directly on a
* Grgit instance.
*
*
*
* - {@link org.ajoberstar.grgit.operation.AddOp add}
* - {@link org.ajoberstar.grgit.operation.ApplyOp apply}
* - {@link org.ajoberstar.grgit.operation.CheckoutOp checkout}
* - {@link org.ajoberstar.grgit.operation.CleanOp clean}
* - {@link org.ajoberstar.grgit.operation.CommitOp commit}
* - {@link org.ajoberstar.grgit.operation.DescribeOp describe}
* - {@link org.ajoberstar.grgit.operation.FetchOp fetch}
* - {@link org.ajoberstar.grgit.operation.LogOp log}
* - {@link org.ajoberstar.grgit.operation.MergeOp merge}
* - {@link org.ajoberstar.grgit.operation.PullOp pull}
* - {@link org.ajoberstar.grgit.operation.PushOp push}
* - {@link org.ajoberstar.grgit.operation.RmOp remove}
* - {@link org.ajoberstar.grgit.operation.ResetOp reset}
* - {@link org.ajoberstar.grgit.operation.RevertOp revert}
* - {@link org.ajoberstar.grgit.operation.ShowOp show}
* - {@link org.ajoberstar.grgit.operation.StatusOp status}
*
*
*
* And the following operations are supported statically on the Grgit class.
*
*
*
* - {@link org.ajoberstar.grgit.operation.CloneOp clone}
* - {@link org.ajoberstar.grgit.operation.InitOp init}
* - {@link org.ajoberstar.grgit.operation.OpenOp open}
*
*
*
* Further operations are available on the following services.
*
*
*
* - {@link org.ajoberstar.grgit.service.BranchService branch}
* - {@link org.ajoberstar.grgit.service.RemoteService remote}
* - {@link org.ajoberstar.grgit.service.ResolveService resolve}
* - {@link org.ajoberstar.grgit.service.TagService tag}
*
*
* @since 0.1.0
*/
class Grgit {
private static final Map STATIC_OPERATIONS = [
init: InitOp, clone: CloneOp, open: OpenOp
]
private static final Map OPERATIONS = [
clean: CleanOp, status: StatusOp, add: AddOp, remove: RmOp,
reset: ResetOp, apply: ApplyOp, pull: PullOp, push: PushOp,
fetch: FetchOp, checkout: CheckoutOp, log: LogOp, commit: CommitOp,
revert: RevertOp, merge: MergeOp, describe: DescribeOp, show: ShowOp
].asImmutable()
/**
* The repository opened by this object.
*/
final Repository repository
/**
* Supports operations on branches.
*/
final BranchService branch
/**
* Supports operations on remotes.
*/
final RemoteService remote
/**
* Convenience methods for resolving various objects.
*/
final ResolveService resolve
/**
* Supports operations on tags.
*/
final TagService tag
private Grgit(Repository repository) {
this.repository = repository
this.branch = new BranchService(repository)
this.remote = new RemoteService(repository)
this.tag = new TagService(repository)
this.resolve = new ResolveService(repository)
}
/**
* Returns the commit located at the current HEAD of the repository.
* @return the current HEAD commit
*/
Commit head() {
return resolve.toCommit('HEAD')
}
/**
* Checks if {@code base} is an ancestor of {@code tip}.
* @param base the version that might be an ancestor
* @param tip the tip version
* @since 0.2.2
*/
boolean isAncestorOf(Object base, Object tip) {
Commit baseCommit = resolve.toCommit(base)
Commit tipCommit = resolve.toCommit(tip)
return JGitUtil.isAncestorOf(repository, baseCommit, tipCommit)
}
/**
* Release underlying resources used by this instance. After calling close
* you should not use this instance anymore.
*/
void close() {
repository.jgit.close()
}
def methodMissing(String name, args) {
OpSyntaxUtil.tryOp(this.class, OPERATIONS, [repository] as Object[], name, args)
}
static {
Grgit.metaClass.static.methodMissing = { name, args ->
OpSyntaxUtil.tryOp(Grgit, STATIC_OPERATIONS, [] as Object[], name, args)
}
}
/**
* Opens a {@code Grgit} instance by looking up the correct repo dir.
* This is a workaround due to the existing deprecated methods.
*/
static Grgit open() {
return OpSyntaxUtil.tryOp(Grgit, STATIC_OPERATIONS, [] as Object[], 'open', [] as Object[])
}
/**
* Opens a {@code Grgit} instance in {@code rootDirPath}. If credentials
* are provided they will be used for all operations with using remotes.
* @param rootDirPath path to the repository's root directory
* @param creds harcoded credentials to use for remote operations
* @deprecated replaced by {@link org.ajoberstar.grgit.operation.OpenOp}
* @throws GrgitException if an existing Git repository can't be opened
* in {@code rootDirPath}
*/
@Deprecated
static Grgit open(String rootDirPath, Credentials creds = null) {
return open(new File(rootDirPath), creds)
}
/**
* Opens a {@code Grgit} instance in {@code rootDir}. If credentials
* are provided they will be used for all operations with using remotes.
* @param rootDir path to the repository's root directory
* @param creds harcoded credentials to use for remote operations
* @deprecated replaced by {@link org.ajoberstar.grgit.operation.OpenOp}
* @throws GrgitException if an existing Git repository can't be opened
* in {@code rootDir}
*/
@Deprecated
static Grgit open(File rootDir, Credentials creds = null) {
return OpSyntaxUtil.tryOp(Grgit, STATIC_OPERATIONS, [] as Object[], 'open', [dir: rootDir, creds: creds])
}
}