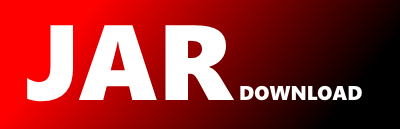
org.aksw.commons.collections.MapUtils Maven / Gradle / Ivy
package org.aksw.commons.collections;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import com.google.common.base.Objects;
import com.google.common.collect.HashMultimap;
import com.google.common.collect.Multimap;
import com.google.common.collect.Sets;
/**
* Created by IntelliJ IDEA.
* User: raven
* Date: 4/22/11
* Time: 2:36 PM
* To change this template use File | Settings | File Templates.
*/
public class MapUtils {
public static void removeAll(Map, ?> map, Iterable> items) {
for(Object o : items) {
map.remove(o);
}
}
/**
* Set the same value for a given set of keys
*
* @param map
* @param keys
* @param value
*/
public static void putForAll(Map map, Iterable keys, V value) {
for(K key : keys) {
map.put(key, value);
}
}
/**
* Compatible means that merging the two maps would not result in the same
* key being mapped to distinct values.
*
* Or put differently:
* The union of the two maps retains a functional mapping.
*
* @param
* @param
* @param a
* @param b
* @return
*/
public static boolean isCompatible(Map a, Map b) {
Set commonKeys = Sets.intersection(a.keySet(), b.keySet());
boolean result = isCompatible(commonKeys, a, b);
return result;
}
public static boolean isCompatible(Set keysToTest, Map a, Map b) {
boolean result = true;
for(K key : keysToTest) {
V av = a.get(key);
V bv = b.get(key);
result = Objects.equal(av, bv);
if(!result) {
break;
}
}
return result;
}
// A version written before guava - Sets.intersection can make sure that any tested key is actually contained in the keyset.
@Deprecated
public static boolean isPartiallyCompatible(Map a, Map b) {
boolean result = isCompatible(a, b);
return result;
}
public static Multimap reverse(Map map) {
Multimap result = HashMultimap.create();
for(Map.Entry entry : map.entrySet()) {
result.put(entry.getValue(), entry.getKey());
}
return result;
}
public static V getOrElse(Map extends K, ? extends V> map, K key, V elze)
{
if(map.containsKey(key)) {
return map.get(key);
}
return elze;
}
public static Map createChainMap(Map a, Map, V> b) {
Map result = new HashMap();
for(Map.Entry entry : a.entrySet()) {
if(b.containsKey(entry.getValue())) {
result.put(entry.getKey(), b.get(entry.getValue()));
}
}
return result;
}
public static V getOrCreate(Map map, K key, Class clazz, Object ... ctorArgs)
{
V result = map.get(key);
if(result == null) {
// TODO Invoke the correct constructor based on the arguments
//Class[] classes = new Class[ctorArgs.length];
//clazz.getConstructor();
if(ctorArgs.length > 0) {
throw new RuntimeException("Constructor arguments not supported yet");
} else {
try {
result = (V)clazz.newInstance();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
map.put(key, result);
}
return result;
}
/**
* Find a mapping of variables from cand to query, such that the pattern of
* cand becomes a subset of that of query
*
* null if no mapping can be established
*
* @param query
* @param cand
* @return
*/
//
// public Iterator
© 2015 - 2025 Weber Informatics LLC | Privacy Policy