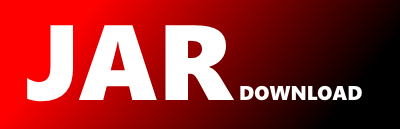
org.aksw.commons.collections.Sample Maven / Gradle / Ivy
package org.aksw.commons.collections;
/**
* Created by IntelliJ IDEA.
* User: raven
* Date: 4/22/11
* Time: 1:47 PM
* To change this template use File | Settings | File Templates.
*/
import java.util.Collection;
import java.util.HashSet;
import java.util.Set;
/**
* Created by Claus Stadler
* Date: Oct 12, 2010
* Time: 5:51:59 PM
*
* A pair of sets of positives and negative examples.
* This class is intended to be used e.g. for training sets, test sets, in information retrieval / machine learning scenarios and such.
* But also for keeping track of the sets of constant values a variable in e.g. jena expression may have.
*
*/
public class Sample
{
public static Sample create()
{
return new Sample(new HashSet(), new HashSet());
}
public static Sample createCopy(Sample other)
{
return createCopy(other.getPositives(), other.getNegatives());
}
public static Sample createCopy(Collection positives, Collection negatives)
{
return new Sample(new HashSet(positives), negatives == null ? null : new HashSet(negatives));
}
public static Sample create(Sample other)
{
return create(other.getPositives(), other.getNegatives());
}
public static Sample create(Set positives, Set negatives)
{
return new Sample(positives, negatives);
}
private Set positives;
private Set negatives;
public Sample(Set positives, Set negatives) {
this.positives = positives;
this.negatives = negatives;
}
public Set getPositives() {
return positives;
}
public Set getNegatives() {
return negatives;
}
public void addAll(Sample other)
{
addAll(other.getPositives(), other.getNegatives());
}
public void addAll(Collection extends T> positives, Collection extends T> negatives)
{
this.positives.addAll(positives);
this.negatives.addAll(negatives);
}
public void clear()
{
positives.clear();
negatives.clear();
}
public boolean isEmpty()
{
return positives.isEmpty() && negatives.isEmpty();
}
public String toString()
{
return "Positives: " + positives + "\nNegatives: " + negatives;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy