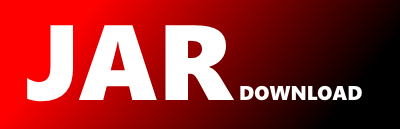
org.aksw.commons.collections.SampleStats Maven / Gradle / Ivy
package org.aksw.commons.collections;
import com.google.common.base.Joiner;
import com.google.common.collect.Sets;
import java.text.DecimalFormat;
import java.util.Collection;
import java.util.Set;
/**
* Created by Claus Stadler
* Date: Oct 19, 2010
* Time: 11:36:20 PM
*/
/**
* all.negatives may be null, however all other fields must be given!
*
* @param
*/
public class SampleStats
{
private static final DecimalFormat formatter = new DecimalFormat("0.######");
private Sample all;
private Set examples;
private Sample correctHits;
private Sample falseHits;
private SampleStats(Sample all, Set examples)
{
this.all = all;
this.examples = examples;
correctHits = new Sample(
Sets.intersection(all.getPositives(), examples),
all.getNegatives() == null ? null : Sets.difference(all.getNegatives(), examples));
falseHits = new Sample(Sets.difference(examples, all.getPositives()),
Sets.difference(all.getPositives(), examples));
}
public static SampleStats create(Sample all, Set examples)
{
return new SampleStats(all, examples);
}
/**
* Statistics for the pool
* All positives that are not in the pool are removed from the examples
*/
public static SampleStats create(Sample pool, Set examples, Sample all)
{
Set deltaPositives = Sets.difference(all.getPositives(), pool.getPositives());
Set refinedExamples = Sets.difference(examples, deltaPositives);
return new SampleStats(pool, refinedExamples);
}
public static SampleStats create(Set allPositives, Set allNegatives, Set examples)
{
return new SampleStats(new Sample(allPositives, allNegatives), examples);
}
public Sample getAll() {
return all;
}
public Set getExamples() {
return examples;
}
public Sample extends T> getTrue() {
return correctHits;
}
// Returns a sample with a view on the true negatives and positives
public Sample extends T> getFalse() {
return falseHits;
}
public double getPrecision()
{
if(getExamples().isEmpty())
return 0.0;
return getTrue().getPositives().size() / (double)getExamples().size();
}
public double getRecall()
{
if(getAll().getPositives().isEmpty())
return 0.0;
return getTrue().getPositives().size() / (double)getAll().getPositives().size();
}
public double getFMeasure()
{
return fMeasure(getPrecision(), getRecall());
}
/*
public double getAccuracy()
{
return null;
}*/
public static double fMeasure(double precision, double recall)
{
double denominator = precision + recall;
return (denominator == 0.0)
? 0.0
: 2 * precision * recall / denominator;
}
public String toString()
{
return "Precision/Recall/FMeasure = " + Joiner.on("/").join(
formatter.format(getPrecision()), formatter.format(getRecall()), formatter.format(getFMeasure())) + " --- " +
"False Negatives " + toStringWithSize(getFalse().getNegatives());
}
public static String formatHumanReadable(SampleStats> stats) {
DecimalFormat formatter = new DecimalFormat("0.##");
return "Precision/Recall/FMeasure = " + Joiner.on("/").join(
"" + formatter.format(stats.getPrecision() * 100.0) + "%",
"" + formatter.format(stats.getRecall() * 100.0) + "%",
"" + formatter.format(stats.getFMeasure() * 100.0) + "%") +
" --- " +
"False Negatives " + toStringWithSize(stats.getFalse().getNegatives());
}
public static String toStringWithSize(Collection> collection)
{
return collection == null ? "(null)" : "(" + collection.size() + ")" + collection;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy