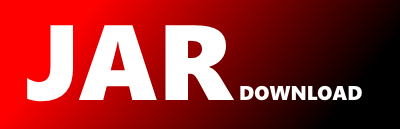
org.aksw.commons.collections.utils.StreamUtils Maven / Gradle / Ivy
package org.aksw.commons.collections.utils;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import com.google.common.collect.AbstractIterator;
public class StreamUtils {
public static Stream> mapToBatch(Stream stream, long batchSize) {
Iterator baseIt = stream.iterator();
Iterator> it = new AbstractIterator>() {
@Override
protected List computeNext() {
List items = new ArrayList<>((int)batchSize);
for(int i = 0; baseIt.hasNext() && i < batchSize; ++i) {
T item = baseIt.next();
items.add(item);
}
List r = items.isEmpty()
? endOfData()
: items;
return r;
}
};
Iterable> tmp = () -> it;
Stream> result = StreamUtils.stream(tmp);
result.onClose(() -> stream.close());
return result;
}
public static Stream stream(Iterator it) {
Iterable i = () -> it;
return stream(i);
}
public static Stream stream(Iterable i) {
Stream result = StreamSupport.stream(i.spliterator(), false);
return result;
}
/**
* Creates a new stream which upon reaching its end performs and action.
* It concatenates the original stream with one having a single item
* that is filtered out again. The action is run as- part of the filter.
*
* @param stream
* @param runnable
* @return
*/
public static Stream appendAction(Stream extends T> stream, Runnable runnable) {
Stream result = Stream.concat(
stream,
Stream
.of((T)null)
.filter(x -> {
runnable.run();
return false;
})
);
return result;
}
// TODO Add to StreamUtils
public static Stream stream(BiConsumer> fn, S baseSolution) {
List result = new ArrayList<>();
fn.accept(baseSolution, (item) -> result.add(item));
return result.stream();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy