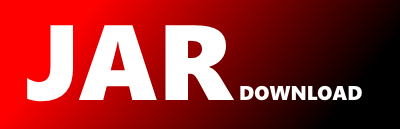
org.aksw.commons.accessors.LazyCollection Maven / Gradle / Ivy
package org.aksw.commons.accessors;
import java.util.AbstractCollection;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.Iterator;
import java.util.Optional;
import java.util.Set;
import java.util.function.Supplier;
import org.aksw.commons.collections.SinglePrefetchIterator;
/**
* Collection that forwards method calls to another one that is
* only instantiated on addition of items if it did not yet exist
*
* Delegates removals to it
*
* @author raven Apr 9, 2018
*
* @param
*/
public class LazyCollection>
extends AbstractCollection
{
protected SingleValuedAccessor accessor;
protected Supplier extends C> ctor;
protected boolean setNullOnEmpty;
public LazyCollection(SingleValuedAccessor accessor,
Supplier extends C> ctor,
boolean setNullOnEmpty) {
super();
this.accessor = accessor;
this.ctor = ctor;
this.setNullOnEmpty = setNullOnEmpty;
}
@Override
public boolean add(T e) {
C backend = accessor.get();
if(backend == null) {
backend = ctor.get();
accessor.set(backend);
}
boolean result = backend.add(e);
return result;
}
public void checkUnset(Collection backend) {
if(setNullOnEmpty && backend.isEmpty()) {
accessor.set(null);
}
}
@Override
public boolean remove(Object o) {
C backend = accessor.get();
boolean result = backend == null ? false : backend.remove(o);
checkUnset(backend);
return result;
}
@Override
public boolean contains(Object o) {
C backend = accessor.get();
boolean result = backend == null ? false : backend.contains(o);
return result;
}
@Override
public Iterator iterator() {
Collection backend = accessor.get();
Iterator baseIt = Optional.ofNullable(backend).orElse(Collections.emptyList()).iterator();
return new SinglePrefetchIterator() {
@Override
protected T prefetch() throws Exception {
while(baseIt.hasNext()) {
T b = baseIt.next();
return b;
}
return finish();
}
@Override
public void doRemove(T item) {
baseIt.remove();
checkUnset(backend);
}
};
}
@Override
public int size() {
Collection backend = accessor.get();
int result = backend == null ? 0 : backend.size();
return result;
}
public static Set test;
public static void main(String[] args) {
SingleValuedAccessor> accessor = new SingleValuedAccessorImpl<>(
() -> LazyCollection.test,
val -> LazyCollection.test = val);
Collection tmp = new LazyCollection<>(accessor, HashSet::new, true);
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
System.out.println("Adding item");
tmp.add("Hello");
System.out.println("Adding item");
tmp.add("World");
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
System.out.println("Removing item");
tmp.remove("World");
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
System.out.println("Removing item");
tmp.remove("Hello");
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
System.out.println("Adding item");
tmp.add("World");
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
System.out.println("Clearing");
tmp.clear();
System.out.println("Content: " + tmp);
System.out.println("Test: " + test);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy