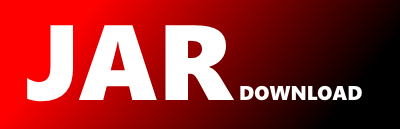
org.aksw.commons.collection.observable.ObservableCollection Maven / Gradle / Ivy
package org.aksw.commons.collection.observable;
import java.beans.PropertyChangeListener;
import java.beans.VetoableChangeListener;
import java.util.Collection;
import java.util.function.Function;
import java.util.function.Predicate;
import com.google.common.base.Converter;
public interface ObservableCollection
extends DeltaCollection
{
/** Whether to notify listeners */
// void setEnableEvents(boolean onOrOff);
// boolean isEnableEvents();
// Runnable addListener(Consumer> listener);
Runnable addVetoableChangeListener(VetoableChangeListener listener);
Registration addPropertyChangeListener(PropertyChangeListener listener);
/** Replace the content of this collection thereby firing only a single event */
// boolean replace(Collection extends T> newValues);
default ObservableCollection filter(Predicate super T> predicate) {
return new FilteredObservableCollection<>(this, predicate);
}
default ObservableCollection map(Function super T, ? extends U> predicate) {
throw new UnsupportedOperationException("not implemented yet");
}
/**
* Return a view of this collection as a scalar value:
* If the collection contains a single item then this item becomes the view's value.
* Otherwise the view's value is null.
*
* @return
*/
default ObservableValue mapToValue() {
return ObservableValueFromObservableCollection.decorate(this);
}
default ObservableValue mapToValue(
Function super Collection extends T>, O> xform,
Function super O, ? extends T> valueToItem) {
return new ObservableValueFromObservableCollection<>(this, xform, valueToItem);
}
default ObservableCollection convert(Converter converter) {
return new ObservableConvertingCollection<>(this, converter);
}
// default ObservableValue mapToValue(Function super Collection super T>, ? extends U> fn) {
// return new ObservableValueFromObservableCollection<>(this);
// }
// default ObservableCollection mapToSet(Predicate predicate) {
// return null;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy