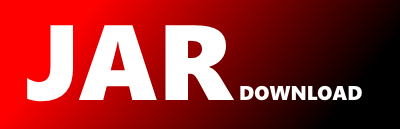
org.aksw.commons.collections.MutableCollectionViews Maven / Gradle / Ivy
package org.aksw.commons.collections;
import java.util.Collection;
import java.util.List;
import java.util.Set;
import java.util.function.Predicate;
import org.aksw.commons.collections.sets.SetFromCollection;
import com.google.common.base.Converter;
public class MutableCollectionViews {
/**
* Return a live-view of the given collection with conflicting elements filtered out.
* Conflicting elements are those for which the converter raises an exception.
*
* @param
* @param backend
* @param converter
* @return
*/
public static Collection filteringCollection(Collection backend, Converter super T, ?> converter) {
Predicate
© 2015 - 2025 Weber Informatics LLC | Privacy Policy