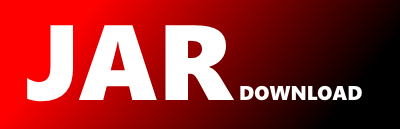
org.aksw.isomorphism.ProblemSolver Maven / Gradle / Ivy
package org.aksw.isomorphism;
import java.util.Collections;
import java.util.function.BinaryOperator;
import java.util.stream.Stream;
/**
* Default implementation of the core algorithm for solving problems.
* TODO Give it some fancy name
*
* @author Claus Stadler
*
* @param The solution type
*/
public class ProblemSolver {
protected ProblemContainer problemContainer;
protected S baseSolution;
protected BinaryOperator solutionCombiner;
public ProblemSolver(ProblemContainer problemContainer, S baseSolution, BinaryOperator solutionCombiner) {
super();
this.problemContainer = problemContainer;
this.baseSolution = baseSolution;
this.solutionCombiner = solutionCombiner;
}
public Stream streamSolutions() {
ProblemContainerPick pick = problemContainer.pick();
Problem picked = pick.getPicked();
ProblemContainer remaining = pick.getRemaining();
Stream result = picked
.generateSolutions()
.flatMap(solutionContribution -> {
S partialSolution = solutionCombiner.apply(baseSolution, solutionContribution);
Stream r;
// If the partial solution is null, then indicate the
// absence of a solution by returning a stream that yields
// null as a 'solution'
if (partialSolution == null) {
r = Collections. singleton(null).stream();
} else {
// This step is optional: it refines problems
// based on the current partial solution
// Depending on your setting, this can give a
// performance boost or penalty
//ProblemContainerImpl openProblems = remaining;
ProblemContainer openProblems = remaining.refine(partialSolution);
if (openProblems.isEmpty()) {
r = Collections. emptySet().stream();
} else {
ProblemSolver nextState = new ProblemSolver(openProblems, baseSolution, solutionCombiner);
r = nextState.streamSolutions();
}
}
return r;
});
return result;
}
public static Stream solve(ProblemContainer problemContainer, S baseSolution, BinaryOperator solutionCombiner) {
ProblemSolver problemSolver = new ProblemSolver(problemContainer, baseSolution, solutionCombiner);
Stream result = problemSolver.streamSolutions();
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy